Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial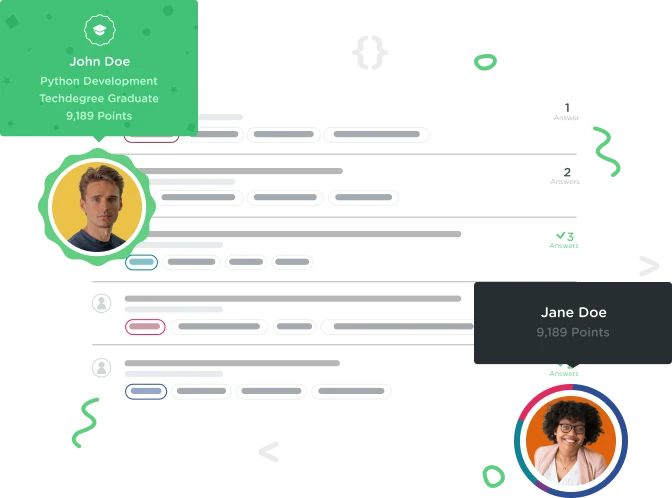
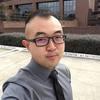
BOHAN ZHANG
4,446 Pointsphp stuck
Extending from a parent class allows the child to inherit all attributes and abilities of the parent. Step 1: Add a new method to the Developer class named displayUserSkills. Step 2: Use the 'name' from the PARENT class, and the 'skills' from the CURRENT class, to replace the <PLACEHOLDERS> and RETURN following string (NOTE that skills separated with a comma and space): <NAME> has the following skills: <SKILL1>, <SKILL2>, <SKILL3> Example Output: Alena has the following skills: PHP, MySQL, HTML
My code:
<?php
//add new child class here class Developer extends User { protected $skills = array();
public function getSkills(){ return $this->skills; }
public function setSkills($an_array){ $this->skills = $an_array; }
public function displayUserSkills(){ return $this -> getSkills();
}
}
$stuff = new Developer ;
$stuff -> setSkills('PHP','MySQL','HTML');
$stuff -> setName('Alena');
echo $stuff->getName() . " has the following skills: " . $stuff->displayUserSkills();
?>
what's wrong with my code?
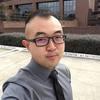
BOHAN ZHANG
4,446 PointsSo, How should I edit my code ?
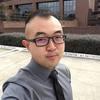
BOHAN ZHANG
4,446 Points$stuff = new Developer ; $stuff -> setSkills(array("PHP","MySQL","HTML")); $stuff -> setName('Alena');
echo $stuff->getName() . " has the following skills: " . $stuff->displayUserSkills();
I edited it but this still not working
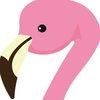
Dave StSomeWhere
19,870 PointsLooks like you also need to handle the array output - have you tried something like implode() - http://php.net/manual/en/function.implode.php?
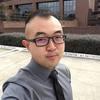
BOHAN ZHANG
4,446 Points$an_array = array("PHP","MySQL","HTML");
$an_array_1 = implode(",",$an_array);
$stuff = new Developer ;
$stuff -> setSkills($an_array_1);
$stuff -> setName('Alena');
echo $stuff->getName() . " has the following skills: " . $stuff->displayUserSkills();
hmm, I tried, but still not go through
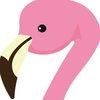
Dave StSomeWhere
19,870 PointsPlease try the implode on $stuff->displayUserSkills()
- the output as opposed to using it when setting the skills (and don't forget the NOTE that wants a comma and a space)
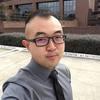
BOHAN ZHANG
4,446 Points$an_array = array("PHP","MySQL","HTML");
$stuff = new Developer ;
$stuff -> setSkills($an_array);
$stuff -> setName('Alena');
echo $stuff->getName() . " has the following skills: " . implode(", ",$stuff->displayUserSkills()); it keeps pop up this line of error: Alena has the following skills: PHP, MySQL, HTMLPHP Warning: strtolower() expects parameter 1 to be string, array given in f1d58377-1ba6-4812-9200-bbc920a21315.php on line 224 Warning: strtolower() expects parameter 1 to be string, array given in f1d58377-1ba6-4812-9200-bbc920a21315.php on line 224
1 Answer
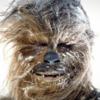
codingchewie
8,764 PointsI figured this out by referencing the Basics video for the recipe, "Object-Oriented PHP Basics" and it will show you at the bottom the Code Sample using the implode which is how I came up with this:
public function displayUserSkills()
{
$userName = parent::getName();
$userSkills = implode(', ', $this->skills);
return "{$userName} has the following skills: {$userSkills}";
}
then you can use:
$foobar = new Developer();
$foobar -> $this->setName("Foobar");
$foobar -> $this->displayUserSkills("PHP", "MySQL", "HTML");
but when coding protected
I've read its better to do:
<?php
//add new child class here
class Developer extends User
{
protected $_skills = [];
public function getSkills()
{
return $this->_skills;
}
public function setSkills($someSkill)
{
$this->_skills = $someSkill;
}
public function displayUserSkills()
{
$userName = parent::getName();
$userSkills = implode(', ', $this->_skills);
return "{$userName} has the following skills: {$userSkills}";
}
}
$foobar = new Developer();
$foobar -> setName("Alena");
$foobar -> displayUserSkills("PHP", "MySQL", "HTML");
Hope this helps you and the next person.

Xheni Myrtaj
Courses Plus Student 2,636 PointsHey, this is not working
Dave StSomeWhere
19,870 PointsDave StSomeWhere
19,870 PointsAre you supposed to pass 1 parameter to the setSkills() method and isn't it supposed to be an array - looks like you are passing 3 strings?