Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial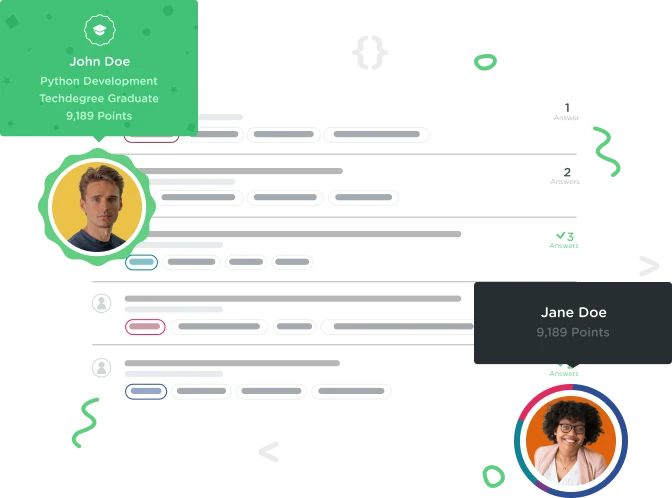
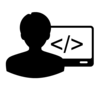
Abe Layee
8,378 PointsPHP username and password within array not matching
Hello, How are you doing? I have this little PHP bug within my code is driving me crazy. Basically I store username and passowrd within my array. I want the users within the array should be able to login into the site and print welcome or invalid information depending if their passowrd and username matches. With the OR || operator, it works fine. On the ohter hand AND operator, it doesn't work. What am I doing wrong in my code?
<?php
$userName = $_POST['userName'];
$userPassword = $_POST['pass_word'];
//echo "user name: " .$getUserName. " <br>" . "password " . $getUserPassword;
//use array exit array_key_exists to print out one time
$user_info = array(
"John"=>"css",
"David"=>"html",
"solo"=>"Js",
"Ellie"=>"tr789ial",
"GreatGuy"=>"abc123",
"blogger"=>"23seventeen23",
"Joe"=>"php",
"Zola"=>"football",
"Messi"=>"Barca",
"Etoo"=>"Africa"
);
foreach ($user_info as $value=> $user_value) {
if ($value === $userName || $user_value === $userPassword) {
if (array_key_exists($userName, $user_info) && array_key_exists($userPassword, $user_info) ) {
echo "<p><h4>You have successfully logged in</h4></p>";
} else {
echo "<p><h4>Sorry wrong information has been entered</h4></p>" . "<br>";
}
}
}
?>
2 Answers
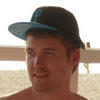
Algirdas Lalys
9,389 PointsHi Abe Layee,
I think the problem is with your second if statement, because it checks for only array_key_exist but not for value I would use in_array() function which checks for values. I think It should be something like.
if (array_key_exists($userName, $user_info) && in_array($userPassword, $user_info) ) {
On the other hand your code would never show else statement, because of the first if statement. Actually I have never done something like that before. But I experimented and found another solution, maybe you will like it.
<?php
// For testing purposes
$userName = 'Joe';
// For testing purposes
$userPassword = 'php';
$login = false;
$user_info = array(
"John"=>"css",
"David"=>"html",
"solo"=>"Js",
"Ellie"=>"tr789ial",
"GreatGuy"=>"abc123",
"blogger"=>"23seventeen23",
"Joe"=>"php",
"Zola"=>"football",
"Messi"=>"Barca",
"Etoo"=>"Africa"
);
// Go through every $key => $value pair
foreach ($user_info as $key => $value) {
// It's ternary operator like if else statement, but less code and much cleaner
// It's like if($key === $username && $value === $userPassword) { $login = true; } else { $login = false;}
$login = ($key === $userName && $value === $userPassword) ? true : false;
// If we found a pair which mathes $key => value then we want to break out of loop, if we don't break than $login might be set to false
// I hope it makes sense:)
if($login) break;
}
// Then we only check if our $login is true then we show login message
if($login) {
echo "<p><h4>You have successfully logged in</h4></p>";
} else {
echo "<p><h4>Sorry wrong information has been entered</h4></p>" . "<br>";
}
?>
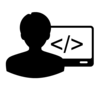
Abe Layee
8,378 PointsThank you very much. I appreciate your effort and the ternary operator
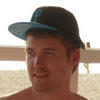
Algirdas Lalys
9,389 PointsCool, I'm glad you like it:)