Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial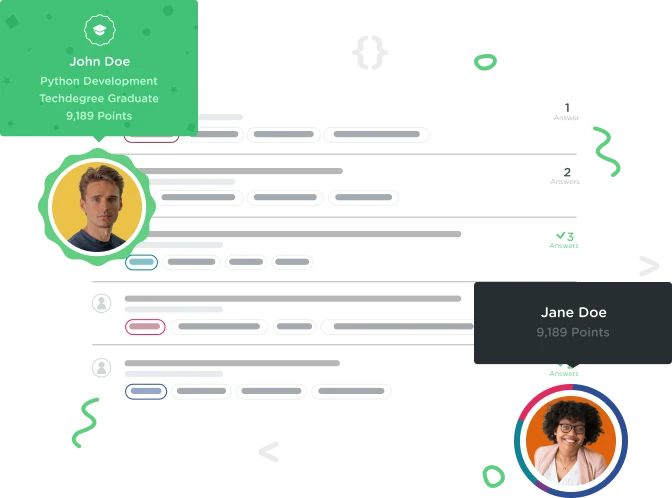

Aaron HARPT
19,845 PointsPHPMailer question
I am having an issue with PHPMailer. Here is my specific error message: "Message could not be sent.Mailer Error: Could not instantiate mail function."
Here is the appropriate code:
<?php
if ($_SERVER["REQUEST_METHOD"] == "POST") {
$name = trim(filter_input(INPUT_POST,"name",FILTER_SANITIZE_STRING));
$email = trim(filter_input(INPUT_POST,"email",FILTER_SANITIZE_EMAIL));
$message = trim(filter_input(INPUT_POST,"message",FILTER_SANITIZE_SPECIAL_CHARS));
// form validation
if ($name == "" || $email == "" || $message == "") {
echo "Please fill in all the required fields: Name, Email, Message";
exit;
}
if ($_POST["address"] != "") {
echo "Bad form input";
exit;
}
require("inc/phpmailer/class.phpmailer.php");
$mail = new PHPMailer;
// make sure email is valid
if (!$mail->ValidateAddress($email)) {
echo "Invalid Email Address";
exit;
}
// $mail->IsSMTP();
// echo "<pre>";
$email_body = "";
$email_body .= "Name " . $name . "\n";
$email_body .= "Email " . $email . "\n";
$email_body .= "Message " . $message . "\n";
// echo $email_body;
// echo "</pre>";
// sending email
$mail->setFrom($email, $name);
// Name is optional
$mail->addAddress('<email address>', '<name>');
// Set email format to HTML
$mail->isHTML(false);
$mail->Subject = 'Comments regarding Website from ' . $name;
$mail->Body = $email_body;
if(!$mail->send()) {
echo 'Message could not be sent.';
echo 'Mailer Error: ' . $mail->ErrorInfo;
exit;
}
header("location:contact.php?status=thanks");
}
?>
Thanks, Aaron
1 Answer

Aaron HARPT
19,845 PointsI actually do have an email and name inserted in my code, but removed them when I posted the code. After working on it some more, here is what my current code looks like:
<?php
// turning on output buffering
ob_start();
if ($_SERVER["REQUEST_METHOD"] == "POST") {
$name = trim(filter_input(INPUT_POST,"name",FILTER_SANITIZE_STRING));
$email = trim(filter_input(INPUT_POST,"email",FILTER_SANITIZE_EMAIL));
$message = trim(filter_input(INPUT_POST,"message",FILTER_SANITIZE_SPECIAL_CHARS));
// form validation
if ($name == "" || $email == "" || $message == "") {
$error_message = "Please fill in all the required fields: Name, Email, Message";
}
// Test: remove this comment
// checking to make sure error_message is not set and that spam honeypot field is blank
// end of where to remove
if (!isset($error_message) && $_POST["address"] != "") {
$error_message = "Bad form input";
}
// requiring phpmailer file
require("inc/phpmailer/class.phpmailer.php");
// new PHPMailer object
$mail = new PHPMailer;
// make sure error_message is not set and that email is valid
if (!isset($error_message) && !$mail->ValidateAddress($email)) {
$error_message = "Invalid Email Address";
}
if (!isset($error_message)) {
// $mail->IsSMTP();
// echo "<pre>";
$email_body = "";
$email_body .= "Name " . $name . "\n";
$email_body .= "Email " . $email . "\n";
$email_body .= "Message " . $message . "\n";
// echo $email_body;
// echo "</pre>";
// sending email
$mail->setFrom($email, $name);
// setting the name and email address of the person receiving the email
// Name is optional
$mail->addAddress('aaron.harpt@gmail.com', 'Aaron Harpt');
// Set email format to HTML. Decide if want it true or false.
$mail->isHTML(false);
// Email subject
$mail->Subject = 'Comments regarding Website from ' . $name;
// Email Body
$mail->Body = $email_body;
// sending the email
if ($mail->Send()) {
// redirecting to thank you message
header("location:contact.php?status=thanks");
exit;
$error_message = 'Message could not be sent.';
$error_message .= 'Mailer Error: ' . $mail->ErrorInfo;
}
}
}
?>
// some content....
<?php
if (isset($_GET["status"]) && $_GET["status"] == "thanks") {
?>
<?php
echo "<div class='col-sm-4 col-xs-12'>";
echo "<h1 id='h1-thanks'>Thank You</h1>";
echo "<p id='p-thanks'>Thank you for contacting us. We will be in contact shortly.</p>";
echo "</div>";
?>
<?php
} else {
?>
// more content....
<?php
if (isset($error_message)) {
echo "<p id='error-message'>" . $error_message . "</p>";
} else {
echo "<p>Please complete the form below.</p>";
}
?>
Currently, when I fill out the form and click submit, I get a blank page, and the url stays at the current page and does not redirect to the thank you message? Also, what do you use to develop with PHP (MAMP, other)?
Zachary Vacek
3,265 PointsZachary Vacek
3,265 PointsLooks like this was posted a few weeks ago, but it looks like the message is not sending because you haven't added an email address and name for the message to be sent to.
You have:
$mail->addAddress('<email address>', '<name>');
You need to include an email address and name for the message to be sent to. Try:
$mail->addAddress('treehouse@localhost', 'Alena');
Hope that helps!