Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial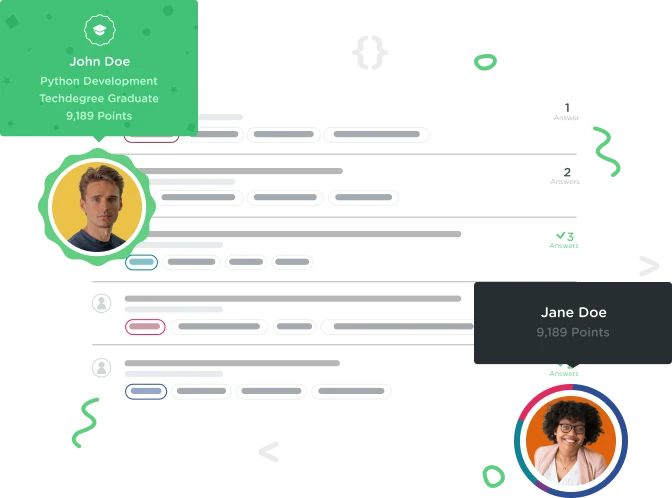

Cristiano Pires
173 PointsplayerAnimator Problems
When I type void FixedUpdate () { if (movement != Vector3.zero) { playerAnimator.SetFloat ("Speed", 3f); } else { playerAnimator.SetFloat ("Speed", 0f); } }
}
I get a error message saying playerAnimator does not exist in the current context. I dont know how to solve this. I double checked my spelling
2 Answers

Oğuzhan Emre Özdoğan
3,579 PointsYou need to assign the animator component to playerAnimator variable, not playerAnimation. First, remove playerAnimation from your code because you don't even need it.
Secondly, assign the animator component to the playerAnimator variable:
void Start () {
// Assign the Animator component to playerAnimator, NOT playerAnimation.
playerAnimator = GetComponent<Animator>();
}
In the end, your code should look like this:
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class PlayerMovement : MonoBehaviour {
private Animator playerAnimator;
private float moveHorizontal;
private float moveVertical;
private Vector3 movement;
void Start() {
// Assign the Animator component to playerAnimator, NOT playerAnimation.
playerAnimator = GetComponent<Animator>();
}
// Update is called once per frame
void Update() {
moveHorizontal = Input.GetAxisRaw("Horizontal");
moveVertical = Input.GetAxisRaw("Vertical");
movement = new Vector3(moveHorizontal, 0.0f, moveVertical);
}
void FixedUpdate() {
if (movement != Vector3.zero)
{
playerAnimator.SetFloat("Speed", 3f);
}
else
{
playerAnimator.SetFloat("Speed", 0f);
}
}
}
I have tried this code, and it is working. Watch the video until it ends to clear any further questions, and have a good day! I hope this helps!

Oğuzhan Emre Özdoğan
3,579 PointsYou need to declare the variable for playerAnimator before you use it. First you should declare it like this;
public class PlayerMovement : MonoBehaviour {
private Animator playerAnimator;
After that, you should initialize it in the Start method, like this:
void Start () {
// Get the animator component of the frog and assign it to playerAnimator.
playerAnimator = GetComponent<Animator>();
}
Your code seems OK but I think it's because you forgot to declare the playerAnimator variable or initialize it.
Note that if you want to paste your code, you should use c# before your code and
again after it ends, so it looks more understandable.
If you have any further issues, please paste all of your PlayerMovement.cs script. I hope this helps!

Cristiano Pires
173 PointsI'm still getting a error message: NullReferenceException: Object reference not set to an instance of an object PlayerMovement.FixedUpdate () (at Assets/Scripts/PlayerMovement.cs:30)
I tried to figure it out but couldn't Also is this code correct I didn't quite understand you
using System.Collections; using System.Collections.Generic; using UnityEngine;
public class PlayerMovement : MonoBehaviour { private Animator playerAnimator;
private Animator playerAnimation;
private float moveHorizontal;
private float moveVertical;
private Vector3 movement;
// Use this for initialization
void Start () { // Get the animator component of the frog and assign it to playerAnimator. playerAnimator = GetComponent<Animator>(); }
playerAnimation = GetComponent<Animator> ();
}
// Update is called once per frame
void Update () {
moveHorizontal = Input.GetAxisRaw ("Horizontal");
moveVertical = Input.GetAxisRaw ("Vertical");
movement = new Vector3 (moveHorizontal, 0.0f, moveVertical);
}
void FixedUpdate () {
if (movement != Vector3.zero) {
playerAnimator.SetFloat ("Speed", 3f);
} else {
playerAnimator.SetFloat ("Speed", 0f);
}
}
}