Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial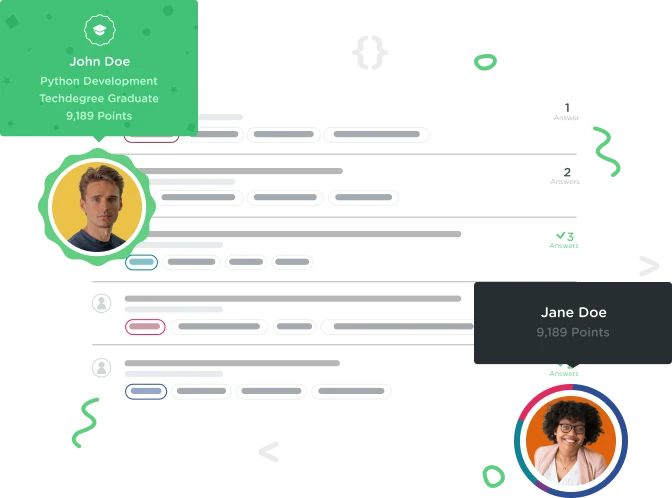
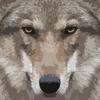
Benneth Paredis
4,597 PointsPlease help im stuck on the last excercise of C# basics
I tried doing if (numberOfTimes < 0) but treehouse keeps telling me to try again. Thanks in advanced.
using System;
namespace Treehouse.CodeChallenges
{
class Program
{
static void Main()
{
Console.Write("Enter the number of times to print \"Yay!\": ");
try {
String yeah = Console.ReadLine();
int numberOfTimes = int.Parse(yeah);
int counter = 0;
while (true) {
if (counter == numberOfTimes) {
break;
}
else {
counter++;
Console.Write("Yay!");
}
}
}
catch (FormatException) {
Console.Write("You must enter a whole number.");
}
}
}
}
3 Answers

srikarvedantam
8,369 PointsYou should validate the input before processing it. Also, you will have easier time if you apply "for" loop in this scenario (rather than a "while" loop). How about the following solution?
using System;
namespace Treehouse.CodeChallenges
{
class Program
{
static void Main()
{
Console.Write("Enter the number of times to print \"Yay!\": ");
int loopLimit = 0;
try {
loopLimit = int.Parse(Console.ReadLine());
}
catch(FormatException) {
Console.WriteLine("You must enter a whole number.");
}
if(loopLimit < 0) {
Console.WriteLine("You must enter a positive number.");
}
else {
for(int i = 0; i < loopLimit; ++i) {
Console.WriteLine("Yay!");
}
}
}
}
}
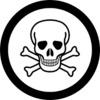
John Weber
6,273 PointsHey! I was also having trouble on the C# Basics final exercise... thanks for posting this!!
Our exercises didn't cover that last line of code... the
for(int i =0; i < loopLimit; ++i) {}
Can you help me understand it? Does it read:
"Integer 'i' begins at zero, and while 'i' is less than loopLimit, print 'yay', then add one to i"?
I was trying to do this with a while loop. :
using System;
namespace Treehouse.CodeChallenges
{
class Program
{
static void Main()
{
Console.Write("Enter the number of times to print \"Yay!\": ");
var entry = int.Parse(Console.ReadLine());
var counter = 0;
while (entry < counter)
{
Console.Write("Yay!");
counter += 1;
}
}
}
}
Assuming the user entered a positive, whole number, why wasn't this working?
Thank you so much for posting helpful stuff on here.... I wish I could upvote your answer twice!
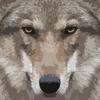
Benneth Paredis
4,597 PointsThank you, worked out great !!!

srikarvedantam
8,369 PointsHi John,
A typical "for" loop functions just as you guessed. Roughly, it's syntax / functionality is as follows:
for (index declaration / initialization; index limit check / condition; index increments / decrements) {
// An iteration of loop logic involving index
}
You can straight away convert a "for" loop to "while" loop as below:
index initialization / initialization;
while(index limit check / condition) {
// An iteration of loop logic involving index
index increments / decrements;
}
Your loop is not iterating as the loop's condition is incorrect. The loop's condition (entry < counter) is checking whether user entered input (i.e. "entry" value) is less than "counter" value (which is initialized to 0). But, it should be the other way around i.e. "counter" value should be checked whether it's value is less than "entry" value (counter < entry) or equivalently (entry > counter). Then, the loop condition will hold true and control flow enters the loop block.
Benneth Paredis
4,597 PointsBenneth Paredis
4,597 PointsOh , and the excercise is telling me to write "You must write a positive number" if 'numberOfTimes' is negative.