Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial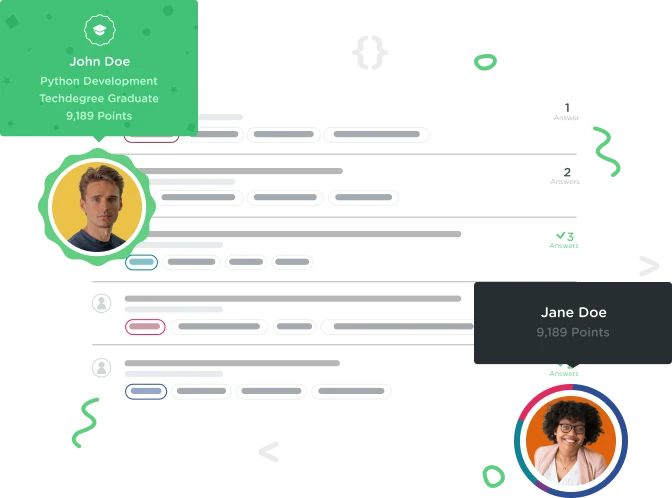

Seyfullah Erdogan
1,922 PointsPlease help me with this Code Challenge!
So I was wondering if you could help me with some of these code challenges. As you might've seen when a member field name doesn't match the style we've talked about, I send an error about it. Let's write some code to validate the style of the field name.
I've added the method validatedFieldName it will return the validated field name. If the value passed in doesn't meet the requirements, throw an IllegalArgumentException. I'll keep you posted on the results every time you press check work.
The link is http://teamtreehouse.com/library/java-objects/delivering-the-mvp/validation-2
Thanks!
public class TeacherAssistant {
public static String validatedFieldName(String fieldName) {
if(! Character.isLetter("m")){
throw new IllegalArgumentException();
}
// These things should be verified:
// 1. Member fields must start with an 'm'
// 2. The second letter in the field name must be uppercased to ensure camel-casing
// NOTE: To check if something is not equal use the != symbol. eg: 3 != 4
return fieldName;
}
}
2 Answers

Chris Shaw
26,676 PointsHi Seyfullah,
Let me start of by saying I know very little about Java but I took a stab at the challenge you're working on and managed to get through it with some minor assistance from Google, below is the solution I've come up and I'll explain what's going on.
startsWithLetterM
This variable simply checks if the first character within the fieldName
string is an lowercase m, if it's not it returns a boolean value of false
otherwise it returns true
.
secondLetterIsUppercase
secondLetterIsUppercase
is a bit more tricky and I'm not even sure it's if a good way of writing it out but what I'm doing is getting the character at index one which results in the second character of the fieldName
string, once I have that I'm then checking if it's an uppercase character by using Character.isUpperCase
which accepts a char
type which the charAt
method returns to us, if the character is uppercase it returns true
otherwise it returns false
.
IF
statement
Finally we have the IF
statement which is pretty simple, all that's happening here is a check to see whether one or both sides of the equality operation equals false
, if at least one of them do equal false
the IllegalArgumentException
is triggered otherwise the fieldName
value gets returned and the code execution continues as normal.
public class TeacherAssistant {
public static String validatedFieldName(String fieldName) {
Boolean startsWithLetterM = fieldName.startsWith("m");
Boolean secondLetterIsUppercase = Character.isUpperCase(fieldName.charAt(1));
if (!startsWithLetterM || !secondLetterIsUppercase) {
throw new IllegalArgumentException();
}
return fieldName;
}
}
Hope that helps.

Jess Sanders
12,086 Pointsprivate char validateGuess(char letter) {
if (!Character.isLetter(letter)) {
throw new IllegalArgumentException("A letter is required."
}
letter = Character.toLowerCase(letter);
if (mMisses.indexOf(letter) >= 0 || mHits.indexOf(letter) >= 0) {
throw new IllegalArgumentException(letter + " has already been guessed") }
return letter;
}
The above code is the example to follow.
In pseudocode, we want to check for the following condition, and throw an error: " if the character at index 0 of fieldName does not equal 'm' OR the character at index 1 of fieldName is not upper case"
public class TeacherAssistant {
public static String validatedFieldName(String fieldName) {
if (fieldName.charAt(0) != 'm' || !Character.isUpperCase(fieldName.charAt(1))) {
throw new IllegalArgumentException("The field name does not match the style");
}
return fieldName;
}
}
Franklin Mark Aguba
4,082 PointsFranklin Mark Aguba
4,082 PointsThanks mate! This code saved my butt. I was struggling on it for hours. I forgot about that Character stuff that was just discussed before that quiz.
Natenda Manyau
3,005 PointsNatenda Manyau
3,005 PointsHi Chris in java we are told that double quotes are used for string, so why is it that we are using them on a char and surprisingly there are no syntax errors?
Chris Shaw
26,676 PointsChris Shaw
26,676 PointsHi Natasha,
The reason I did that is because the
startsWith
method doesn't have an overload forchar
therefore it would trigger a syntax error, the main use for thestartsWith
method is to match one or more characters in a string thus it's perfect for this example.In saying that you can also compare the first character against a
char
as seen in the below example which would line up better with the course overall.Boolean startsWithLetterM = fieldName.charAt(0) == 'm';
Hope that helps.
Natenda Manyau
3,005 PointsNatenda Manyau
3,005 PointsThank you.
i know understand