Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial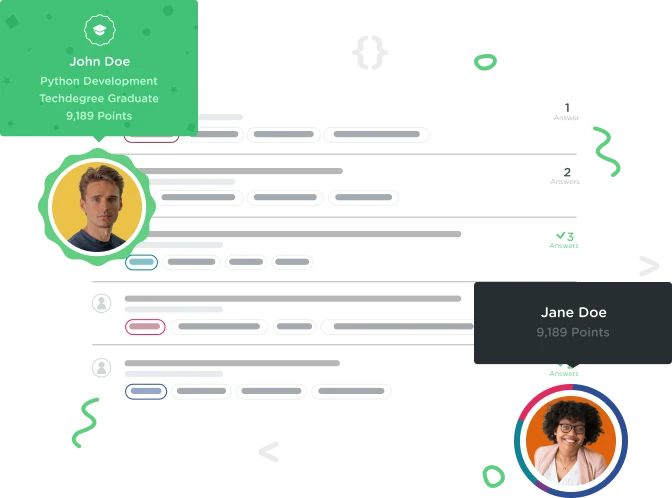
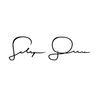
Filip Diarra
6,647 PointsPlease help solve this challenge on Classes
I cannot for the life of me figure out this challenge. I don't quite grasp how to initialize the subclass properly and what function to write here.
If you could provide an answer with an explanation, that would be AMAZING!
Thank you!
3 Answers

Anjali Pasupathy
28,883 PointsTo subclass a class, you need to use the following format:
class SubClass: ParentClass {
// DEFINE SUBCLASS PROPERTIES AND METHODS
}
For this challenge, SubClass is Robot, and ParentClass is Machine.
This challenge also asks you to override Machine's move: function. To do this, you write Machine's move: function header with the keyword "override" in front of it, and provide the new implementation for that function inside the body.
class Robot: Machine {
override func move(direction: String) {
// PROVIDE NEW IMPLEMENTATION FOR move: HERE
}
}
The challenge provides instructions for how to implement move. It asks you to use a switch statement to determine whether direction is equal to "Up", "Down", "Left", or "Right", and change the location accordingly. For the default case, the challenge tells you to use the keyword "break".
I hope this helps!
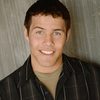
Kyler Smith
10,110 PointsThis one is tricky!!! To make a subclass you need to create the class followed by a colon and the Main class. The class Machine already has a function called move, so you need to override it in your robot class, using the override keyword. In the function, you can either use 'if else' statements or switch statements, I used switch. You want to switch on the String that is being passed in, in our case it is held in the variable 'direction'. Good luck to you!
class Robot: Machine {
override func move(direction: String) {
// implement
}
}
}
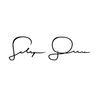
Filip Diarra
6,647 PointsThis helped a lot, thank you!
Why is is that we don't have to override the init nor we have to add the super.init instance here?
Thanks!

Anjali Pasupathy
28,883 PointsYou're welcome! You only need to override init if you're changing what the init does, or adding properties that need to be initialized in the init method. For example, if you added a property called name of type String, you would need to override the init method and call super.init():
class Robot: Machine {
let name: String
override init(name: String) {
self.name = name
super.init()
}
// OVERRIDE NECESSARY METHODS OR ADD METHODS BELOW
}
I hope this helps!