Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial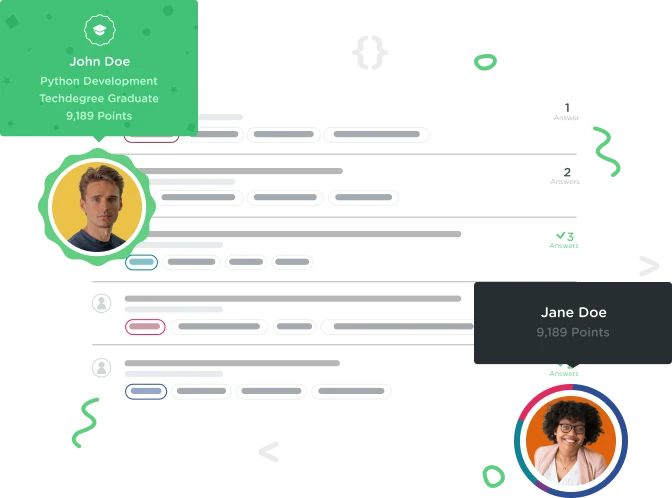
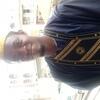
Temitope Ogunleye
Full Stack JavaScript Techdegree Student 2,186 PointsPlease review my code
// Declare all variables at the top
let num1;
let num2;
let message;
// 2. Add an alert to announce the program with a message like "Let's do some math!"
alert("Let's do some math!");
// 3. Create a variable and use the prompt() method to collect a number from a visitor
num1 = prompt("Give me a number");
// 4. Convert that value from a string to a floating point number
num1 = parseFloat(num1);
// 5. Repeat steps 3 and 4 to create a second variable and collect a second number
num2 = prompt("Give me a second number");
num2 = parseFloat(num2);
// 6. Create a new variable -- message -- which you'll use to build
// a complete message to print to the document
// Start by creating a template string that includes <h1> tags as well
// and the two input numbers. The string should look something like this:
// `<h1>Math with the numbers 3 and 4</h1>
// "3 + 4 = 7"`
// "3 * 4 = 12"
// "3 / 4 = 0.75"
// "3 - 4 = -1"
// `;
//where the two numbers are the varlues input from the user. Using Template strings to create this
// Check if input is a number
if (isNaN(num1 || num2) || (isNaN(num1 && num2))) {
message = `Please enter a number`
} else if (num2 === 0){
message = `<h1>Math with the numbers ${num1} and ${num2}</h1>
<p>${num1} + ${num2} = ${num1 + num2}</p>
<p>${num1} * ${num2} = ${num1 * num2}</p>
<p>Division by 0 is not possible</p>
<p>${num1} - ${num2} = ${num1 + num2}</p>
`;
} else {
message = `<h1>Math with the numbers ${num1} and ${num2}</h1>
<p>${num1} + ${num2} = ${num1 + num2}</p>
<p>${num1} * ${num2} = ${num1 * num2}</p>
<p>${num1} / ${num2} = ${num1 / num2}</p>
<p>${num1} - ${num2} = ${num1 - num2}</p>
`
};
// 10. Use the document.write() method to print the message variable
// to the web page. Open the finished.png file in this workspace
// to see what the completed output should look like
document.write(message);