Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial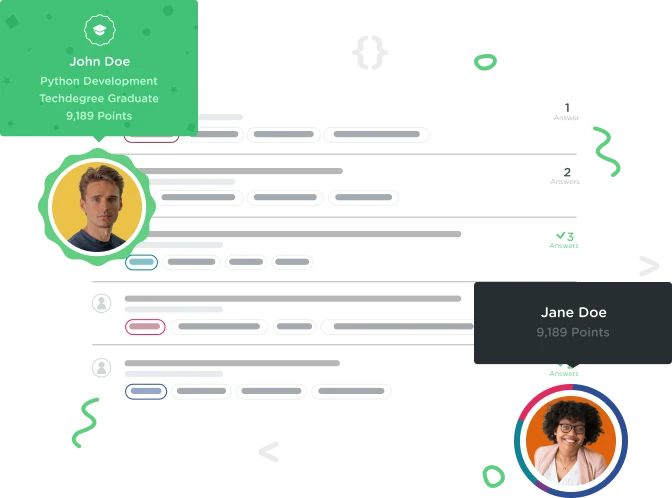

Kevin Dunn
6,623 PointsPressing button takes user to php file
Hi, I have a contact form and it seems to work, but there is a small problem. When clicking the send email button, it takes me to the php file/code. So how do I prevent the button from taking me to the php file and stay on the main page?
HTML
<form id="contact-me-form" action="form_process.php" method="post">
<input type="text" name="userName" placeholder="Your Name">
<input type="email" name="userEmail" placeholder="Email Address">
<textarea name="userMessage" placeholder="Type Your Message Here"></textarea>
<input id="sendEmail" type="submit" name="userSubmit" value="Send">
</form>
<p id="confirmationMessage">Your message has been sent!</p>
PHP
<?php
$userName = $_POST['userName'];
$userEmail = $_POST['userEmail'];
$userMessage = $_POST['userMessage'];
$to = "test@testmail.com";
$subject = "New Message";
mail ($to, $subject, $userMessage, "From: " . $userName);
?>
JAVASCRIPT
const sendEmail = document.getElementById('sendEmail');
const contactMeForm = document.getElementById('contact-me-form');
/Remove text after sending email
sendEmail.addEventListener('click', function(){
contactMeForm.reset();
});
//Confirmation message
$('#sendEmail').click(function(){
$("#confirmationMessage").show().delay(4000).fadeOut('slow');
});
Thanks!
3 Answers
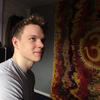
Daniel Lucas
3,604 PointsTo use PHP your file needs to have a .php extension however HTML can be used in both .html and .php files.
(Not sure if you need help with this bit, but just in case) You want to redirect your .php script back to the file with your message on it. For example if you had a file called message.html or message.php, You would redirect using the following code.
<?php
header('Location:message.html');
// or
header('Location:message.php');
?>
If you want to output a message you could create a server variable with $_SESSION. This value will persist as long as your server is running and acts like a normal variable.
If you wanted to output a message after a redirect you could use $_SESSION as follows on your message sending PHP script.
<?php
session_start();
$_SESSION['message'] = 'sent';
?>
And check it with a bit of PHP on your message page.
<?php
session_start();
if ( isset( $_SESSION[ 'message' ] ) ) {
if ( $_SESSION[ 'message' ] == 'sent' ) {
echo 'Message Sent msg here';
unset($_SESSION['message'];
};
};
?>
The session_start() function is used so PHP knows to listen out for $_SESSION.
The isset() function is used to check if a value is set. If $_SESSION['message'] is called when it's not set PHP will output an error message that the user will see and you don't want that. If you don't understand it try running the code without the isset() if statement and see what happens.
The unset() function just removes the value set to $_SESSION['message']. I do this so that the message won't be sent to the user if they come back to the page later on without sending a message. If you don't really understand it, play around with it or read up on it from the PHP manual.
You could also store different values in $_SESSION['message'] which means you could check for and then set errors to the value that could be output to the user, for example the following.
<?php
$_SESSION['message'] = 'sent';
$_SESSION['message'] = 'invalid email';
$_SESSION['message'] = 'no message';
$_SESSION['message'] = 'no subject';
$_SESSION['message'] = 'error';
?>
If you really want to use JS to output your message you would have to use AJAX to call the message sending PHP script without refreshing the page and then run a bit of JS that would output a message, but you'd have to check somewhere else because I don't know AJAX yet.
Hope this helps
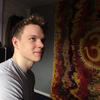
Daniel Lucas
3,604 PointsYou could either use AJAX to stay on the same page and run the code, but I don't know much about AJAX so I can't help you there.
Your other option is to use a redirect.
<?php
header('Location:main_page.php');
?>
You'll want to add this to the end of your script so it runs after your script executes.
<?php
$userName = $_POST['userName'];
$userEmail = $_POST['userEmail'];
$userMessage = $_POST['userMessage'];
$to = "test@testmail.com";
$subject = "New Message";
mail ($to, $subject, $userMessage, "From: " . $userName);
header('Location:YOUR_FILE.php');
?>

Kevin Dunn
6,623 PointsThanks for your answer! But for some reason I'm still not able to stay on the same page after clicking the send button. I am still being directed to my php file.
I tried to use index.html instead of index.php. Not even 100% sure if php accepts .html as an extension since I have very little php experience.
I even tried to keep the action method in the form empty like so:
<form id="contact-me-form" action="" method="post">
This method above kind of works. It doesn't redirect me to my php file, but the problem here is that the page refreshes, meaning that the "Your message has been sent!" message will be gone due to the page being refreshed...