Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial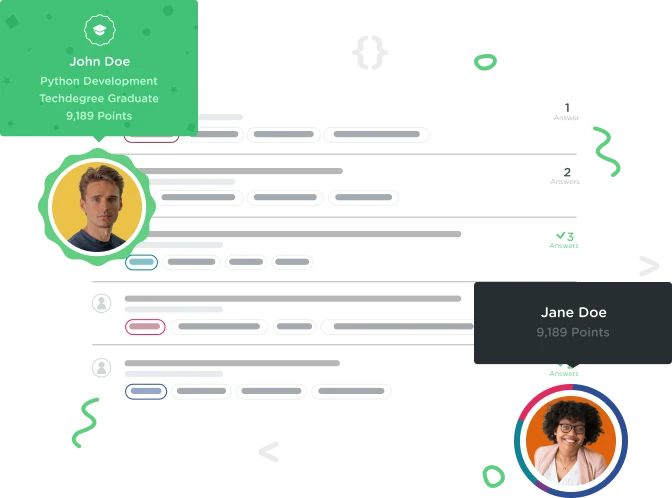

Dejan Delev
461 Pointsprints out the names of all the names in the list instead of printing them out separately
my problem is with the FOR statement, when I want it to show the players on the team, it shows as Player 1: [all the names on the list], Player 2: [all the names on the list] etc.
# TODO Create an empty list to maintain the player names
player_names = []
# TODO Ask the user if they'd like to add players to the list.
# If the user answers "Yes", let them type in a name and add it to the list.
# If the user answers "No", print out the team 'roster'
add_player = input("Would you like to add a player to your team? Yes/No: ")
while add_player.lower() == "yes":
name = input("\nEnter the name of the player to add to the team: ")
player_names.append(name)
add_player = input("Would you like to add a player to your team? Yes/No: ")
# TODO print the number of players on the team
print("There are {} players on the team.".format(len(player_names)))
# TODO Print the player number and the player name
# The player number should start at the number one
player_number = 1
for player in player_names:
print("Player {}: {}".format(player_number, player_names))
player_number += 1
# TODO Select a goalkeeper from the above roster
keeper = input("Select a goalkeeper by selecting the player's number. (1-{}) ".format(len(player_names)))
keeper = int(keeper)
# TODO Print the goal keeper's name
# Remember that lists use a zero based index
print("Great! The goalkeeper for the game will be {}.".format(player_names[keeper - 1]))
2 Answers
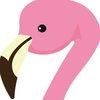
Dave StSomeWhere
19,870 PointsThe issue is that you are passing the entire list (player_names
) to the format function. You should be passing player
- that's the purpose of creating it as the for loop variable.
for player in player_names: # you want to use this player
print("Player {}: {}".format(player_number, player_names)) # player_names is the whole list
# change to
print("Player {}: {}".format(player_number, player)) # Should be player
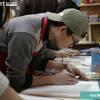
Cao Tru
Courses Plus Student 1,103 PointsThanks Dave StSomeWhere really make sense
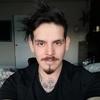
Carlos Muñoz Sanchezllanes
4,407 PointsDave!! Thank you so much, this helped a ton!! My raccoon hands thank you!
Dejan Delev
461 PointsDejan Delev
461 Pointsthat makes a lot of sense! Thanks!
Cao Tru
Courses Plus Student 1,103 PointsCao Tru
Courses Plus Student 1,103 PointsCan you explain for me about player lable because i didn't see player label before and now we used it in for loop. I really confused. <p>for player in player_names:</p>
Dave StSomeWhere
19,870 PointsDave StSomeWhere
19,870 PointsCao Tru, The
player
variable is dynamically created in thefor
loop's scope as a nice/builtin feature of thefor
loop. It is common practice to use a plural on the list likeplayer_names
and then use (create on the fly) a singular value for the iterator - likeplayer
orplayer_name
(without out 's').