Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial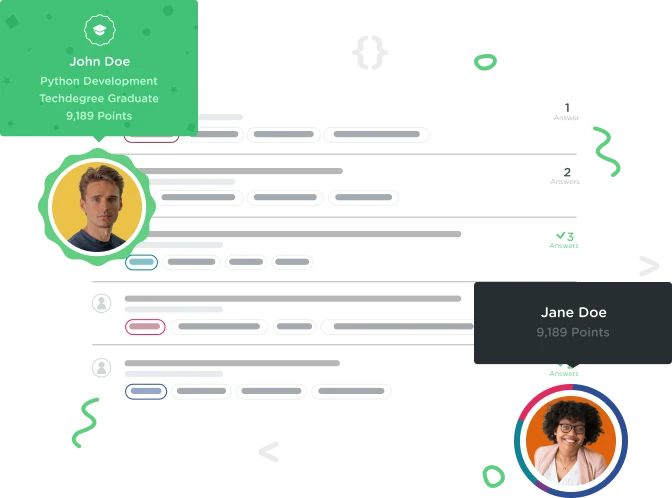

Unsubscribed User
2,263 PointsProblem with struct
In the Swift Recap code challenge, part 1, this code was accepted as a correct answer:
// Code Challenge Part 1
struct Tag {
let name: String
}
struct Post {
let title: String
let author: String
let tag = Tag(name: "Swift")
init(title: String, author: String) {
self.title = title
self.author = author
}
}
let firstPost = Post(title: "Hello World", author: "Brian Cassidy")
Yet in part 2 of the challenge, this failed even though it compiled in the Xcode playground that I tried first:
// Code Challenge Part 2
struct Tag {
let name: String
}
struct Post {
let title: String
let author: String
let tag = Tag(name: "Swift")
init(title: String, author: String) {
self.title = title
self.author = author
}
func description() -> String {
return "\(title) by \(author). Filed under \(tag)"
}
}
let firstPost = Post(title: "Hello World", author: "Brian Cassidy")
let postDescription = firstPost.description()
I had trouble passing a String in as an init parameter for firstPost, so I simply set the stored property in the body of the struct, and it worked fine. When I set postDescription = firstPost.description(), the output appears as:
"Hello World by Brian Cassidy. Filed under Tag(name: "Swift")"
Obviously, that's not correct either.
I can't seem to figure it out with Apple's Swift 2.2 documentation.
Any tips on what I'm doing wrong? Thanks in advance...
struct Tag {
let name: String
}
struct Post {
let title: String
let author: String
let tag = Tag(name: "Swift")
init(title: String, author: String) {
self.title = title
self.author = author
}
func description() -> String {
return "\(title) by \(author). Filed under \(tag)"
}
}
let firstPost = Post(title: "Hello World", author: "Brian Cassidy")
let postDescription = firstPost.description()
1 Answer

Reed Carson
8,306 PointsWell even though that code passed the first part of the challenge for whatever reason, its not quite what its looking for. First, youve hard coded the tag, and created an initializer which is cool but not asked for by the challenge. Neither is it necessary because structs have their own free memberwise initializers if no custom init method is written, which does exactly what the one you wrote does.
struct Tag {
let name: String
}
struct Post {
var title: String
var author: String
var tag: Tag
func description() -> String {
return ("\(title) by \(author). Filed under \(tag.name)")
}
}
let firstPost = Post(title: "Reed", author: "yaaaayy", tag: Tag(name: "name"))
let postDescription = firstPost.description()
It shouldnt have let your code pass at first when it was going to reject it later.

Unsubscribed User
2,263 PointsThanks, Reed. I didn't initially add an init() method to Post, knowing that structs have member wise initializers, but I was having trouble passing a string for "tag" in the firstPost instantiation. I hadn't thought of instantiating the string for "tag" right in the parameters for the firstPost instantiation. I appreciate the feedback.
Unsubscribed User
2,263 PointsUnsubscribed User
2,263 PointsI made it a bit farther, but the code challenge is still failing even the my Xcode playground executes and produces the desired results. I had forgotten to access the name property of tag using dot notation (duh):
I'm still confused about why the second part of code challenge is failing though.