Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial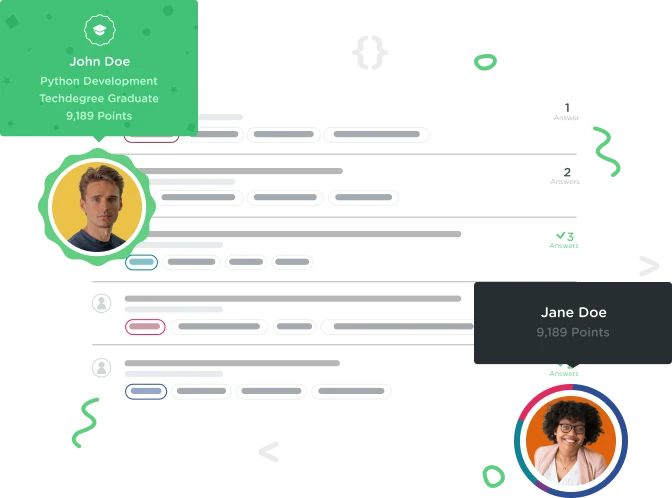
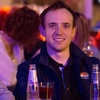
Björn Wauben
10,748 Pointsproblem writing function
I have been stuck on this challenge for more then two hours allready. It's the last bullet point from the JavaScript Basics course I need to pass. At a certain point after rewatching several segments I skipped it and saved it for last. Somehow I am still clueless about what I need to do here.
The question is: "Create a new function named max() which accepts two numbers as arguments. The function should return the larger of the two numbers. You'll need to use a conditional statement to test the 2 numbers to see which is the larger of the two. "
What's my game plan here? Should I declare a return value which includes a conditional statement and then call the function? Hopefully someone can push me in the wright direction. For now I call it a day and start fresh tomorrow so I can pass this last challenge.
- side-question: am I in for a hard time if i'm struggling with these basic types of situations allready?
function max(10,20) {
}
2 Answers
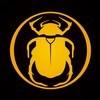
rydavim
18,814 PointsYou're creating a function to compare any two numbers passed to it, and return the larger of the two values.
Try breaking it down into steps:
- Define a function named max that accepts two variables.
- Compare the two variables.
- Return the larger of the two variables.
You can compare the variables using an if else structure. You don't even need an else if!
Let me know if you'd prefer a walkthrough of the solution, sometimes hints just aren't enough! If you've gotten through the rest of the course, I wouldn't worry too much about continuing.
Solution :
// The function should receive two variables. You can name these anything you like.
// I picked x and y because they're common mathematical variables for numbers.
function max(x, y) {
// You want to compare the two numbers. Keep using the variables you defined.
if (x > y) {
// If x is greater than y, we want to return x.
return x;
} else {
// Otherwise, x is not greater than y, so we want to return y.
return y;
}
};
Note that the challenge doesn't explicitly deal with the numbers being equal, so you don't really need an else if clause.

John Magee
Courses Plus Student 9,058 PointsFirstly, you need to rethink how you've defined your function and the arguments.
Secondly, you need to build your function so it compares any two numbers and returns the larger of the two numbers.
Björn Wauben
10,748 PointsBjörn Wauben
10,748 PointsIf you could walk me through the solution I would be very thankful. I tried the code below but i'm not sure about the variables and the way I tried to "return" them. The workcase gives a syntax error.
'''JavaScript function max(number1, number2) { if number1 < number2 { return(number2); } else { return(number1) } } '''
rydavim
18,814 Pointsrydavim
18,814 PointsEdited a solution into the answer post. In your answer you've got the right idea, but let me know if there's anything that still not clicking for you.