Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial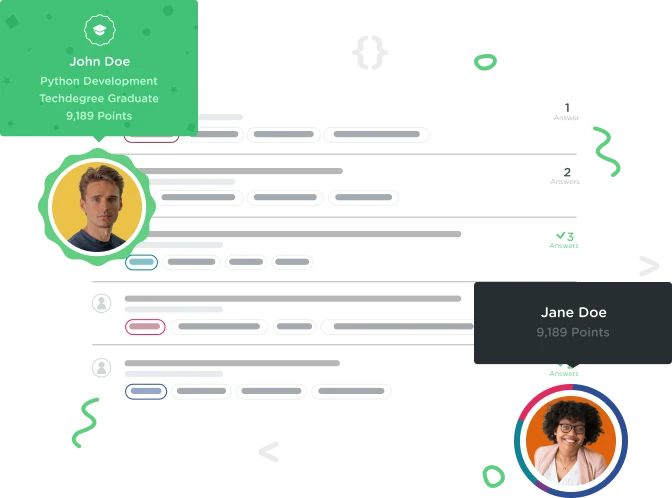

Eilam Maimoni
Courses Plus Student 9,618 PointsProcess is terminated due to StackOverflowException.
class Student { // Personal Info public string Name { get; set; } public string ID { get; set; }
// Grades
public int BagrutGrade { get { return BagrutGrade; } set
{
if (value > 135 || value < 60)
throw new ArgumentOutOfRangeException("The Bagrut grade is out of range");
else
BagrutGrade = value;
} }
public int PsychometricGrade{ get { return PsychometricGrade; } set
{
if (value > 800 || value < 450)
throw new ArgumentOutOfRangeException("The Bagrut grade is out of range");
else
PsychometricGrade = value;
} }
public int InterviewGrade { get { return InterviewGrade; } set
{
if (value > 100 || value < 70)
throw new ArgumentOutOfRangeException("The Bagrut grade is out of range");
else
InterviewGrade = value;
} }
public Student(string name, string iD, int bagrutGrade, int psychometricGrade, int interviewGrade)
{
Name = name;
ID = iD;
BagrutGrade = bagrutGrade;
PsychometricGrade = psychometricGrade;
InterviewGrade = interviewGrade;
}
}
This is the code that I've been requested to write, and for some reason, after I create a student object and trying to access one of the properties, it is printing the console the exception in the name of this question. Somebody knows why it's throwing this exception?
1 Answer
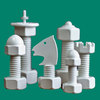
Steven Parker
231,110 PointsA "StackOverflowException" is a common result of a recursive loop, which is what is occurring when any of the properties defined here are accessed. For example:
public int BagrutGrade { get { return BagrutGrade; } //...
This code says that when an access to "BagrutGrade" is made, it should return the value of "BagrutGrade", but that means calling the "get" accessor again, and again, and again, etc.
You probably intended to use a backing field, which must have a different name from the property and a separate declaration. A common convention is to name the backing field starting with an underscore (_), followed by the property name.