Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial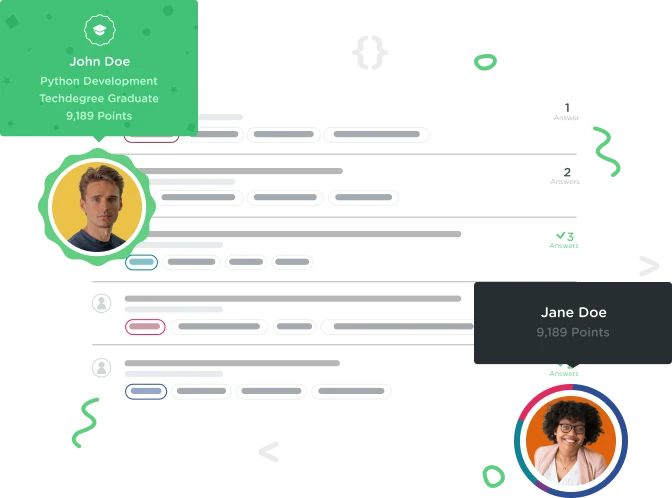
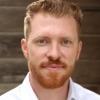
Marc Balestreri
8,211 PointsQuestion about Not Operator Logic
I noticed something that has confused me. The not operator used in Jason's second example still executes when the if statement is false, which can't be right - I must not be understanding how this works.
car1_speed = 50 car2_speed = 60
if !(car1_speed == car2_speed) puts "Car 1 and Car 2 are not going the same speed." end
if !((car1_speed > 40) && (car1_speed > car2_speed)) puts "Car 1 is going fast, but not as fast as car 2." end
As I understand it, the not operator should influence the second IF statement that car1_speed is NOT greater than 40 and, therefore, renders the if statement false (since car1_speed = 50). But when Jason runs the code it works and prints the puts statement. When you separate it out as follows:
car1_speed = 50 car2_speed = 60
if !(car1_speed == car2_speed) puts "Car 1 and Car 2 are not going the same speed." end
if !(car1_speed > 40) && !(car1_speed > car2_speed) puts "Car 1 is going fast, but not as fast as car 2." end
The second IF statement doesn't print. It's as if the Not Operator doesn't follow order of operations when used outside of parentheses. What am I misunderstanding - the NOT operator should be using "opposite" logic, correct?
2 Answers

Gavin Ralston
28,770 PointsOk so...
car1_speed = 50 car2_speed = 60
if !(car1_speed == car2_speed)
puts "Car 1 and Car 2 are not going the same speed."
end
if !(car1_speed > 40) && !(car1_speed > car2_speed)
puts "Car 1 is going fast, but not as fast as car 2."
end
Work from the inside out and it should make more sense. The negation (or not) operation is going to "flip" each individual boolean first, as it has a higher precedence than the && operator.
So you'll flip the first part of the second statement around:
!(car1_speed > 40)
!(50 > 40)
!(true)
false
...and then not even run the second evaluation, since it'll short circuit as they both have to be true (the && part), and you've already evaluated the first one to false.

Gavin Ralston
28,770 PointsSo it looks like (based on the puts string) that the idea would be to see if:
car1 is going faster than 40, AND car1 isn't as fast as car2
So maybe double-check the video and see if that first condition isn't negated. That seems to make more sense, given the output message:
if (car1_speed > 40) && !(car1_speed > car2_speed)
puts "fast test passed, and not going as fast as the other car test also passes, so this prints"
end

Paulo Moreira
2,963 PointsI got the same doubt. If it's FALSE why does it yet present the puts message? I'm a begginer and what I understand so far, is that when something is false there's no message to output on the screen. Am I that wrong?
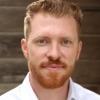
Marc Balestreri
8,211 PointsThanks Gavin,
Ya, it appears that I wasn't paying attention to operator precedence like you mentioned and that the following video tutorial was, in fact, operator precedence - which helped shine the light on my issue. Thanks for the quick response.

Gavin Ralston
28,770 PointsCool! Hope I helped, or help the next person along if you already had that problem squashed.
Marc Balestreri
8,211 PointsMarc Balestreri
8,211 PointsJason notes that it negates anything inside of the parentheses, which I assume must make the if statement true and allow it to execute the 2nd puts statement. I'm assuming, since the if statement would be false since the car1_speed > car2_speed is false, that the whole statement is rendered true because the ! (not operator) makes is opposite the false statement. In essence, its not an order of operations type deal and I answered my original question, correct?