Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial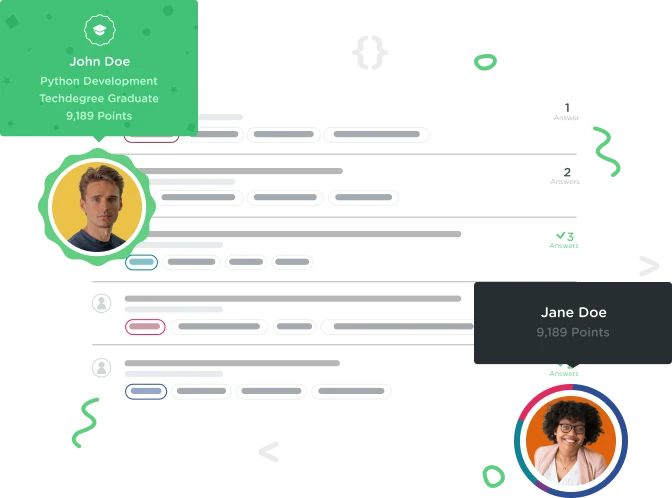

JingChi Liang
1,081 PointsQuestion if Directly set IsActive
Original Invader.cs code from video
namespace TreehouseDefense
{
class Invader
{
private readonly Path _path;
private int _pathStep = 0;
public MapLocation Location => _path.GetLocationAt(_pathStep);
public int Health {get; private set;} = 2;
public bool HasScored {get {return _pathStep >= _path.Length;}}
public bool IsNeutralised => Health <= 0;
public bool IsActive => !(IsNeutralised || HasScored);
public Invader(Path path)
{
_path = path;
}
public void Move() => _pathStep += 1;
public void DecreaseHealth(int factor)
{
Health -= factor;
}
}
}
why console will return
Invader.cs(16,32): error CS0236: A field initializer cannot reference the nonstatic field, method, or property
TreehouseDefense.Invader.IsNeutralised'
Invader.cs(16,49): error CS0236: A field initializer cannot reference the nonstatic field, method, or property
TreehouseDefense.Invader.HasScored'
if I directly put
public bool IsActive = !(IsNeutralised || HasScored);
I think that although I didnt make it into property which allows other classes to get, this bool should can be set in this way, does it?
1 Answer
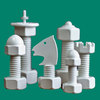
Steven Parker
231,122 PointsYou want this to be a property, not a field.
The compiler error is reminding you that you cannot use instance fields or properties to initialize other instance fields. But even if this was possible you would not want it. You want IsActive to be a calculated property that will reflect the current state of the invader every time you check it. If you initialzed this as field, it would always reflect that state it was in as it was constructed, which would not be useful to the program later.