Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial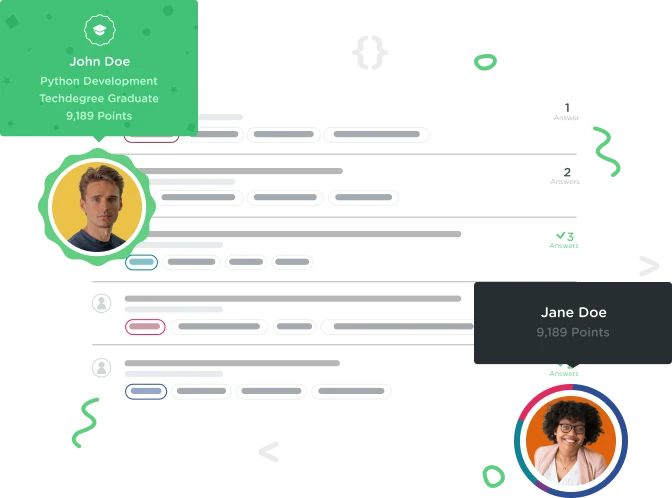
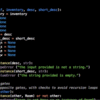
Hammad Ahmed
Full Stack JavaScript Techdegree Student 11,910 PointsQuiz Challenge Code Not Working
function print(message) {
document.write(message);
}
var correctAnswers = [];
var wrongAnswers = [];
var questions = [
["What is the capital of Mexico?", "mexico city"],
["How many legs does a insect has?", "6"],
["Name the author of Laravel?", "taylor otwell"],
["Who invented PHP?", "Hammad"],
["Which programming language is named after a snake?", "Phyton"],
["Which programming language is the name of a gem?", "Ruby"],
["Who is the first president of USA?", "Washington"]
]
print(questions[1][0]);
for ( var i = 0; i <= questions.length; ++i ) {
var answer = prompt(questions[i][0]);
answer.toLowerCase();
if ( answer === questions[i][1] ) {
correctAnswers.push(questions[i][0]);
} else if ( answer !== questions[i][1] ) {
wrongAnswers.push(questions[i][0]);
}
}
print("You answered " + correctAnswers.length + " correctly");
for ( var i = 0; i <= correctAnswers.length; i++ ) {
print(i + ". " + correctAnswers[i]);
}
for ( var i = 0; i <= wrongAnswers.length; i++ ) {
print(i + ". " + wrongAnswers[i]);
}
1 Answer

mathieu blanchette
8,254 PointsI believe it's because your for loops give an "Index Out of Range Error".
for ( var i = 0; i <= correctAnswers.length; i++ )
shouldn't have an "=" sign otherwise it's trying to get a property of something that hasn't been declared yet. You should remove the "=" like so:
for ( var i = 0; i < correctAnswers.length; i++ )
Nicholas Jensen
5,969 PointsNicholas Jensen
5,969 PointsThis answer is correct, but the explanation is a bit strange to me. I think it would be better said as a reminder that when using
correctAnswers.length
counts the number of elements in the array, starting with one, while the index starts with 0. So the length variable is always 1 more than the last index, so you shouldn't use <= but <.