Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial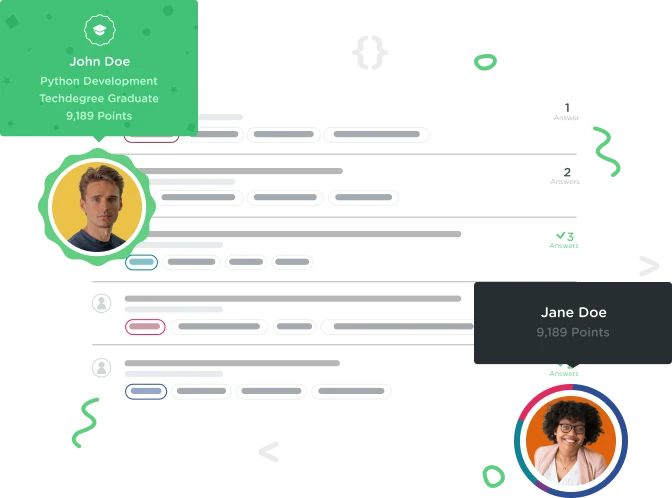

Dominic Bishop
Full Stack JavaScript Techdegree Graduate 15,302 PointsRandom Quote Generator: Is this rubbish?
Ive spent a lot time writing this and I feel that I have just made myself look silly. Is this any good? Does it work? Im a super noob and dont know where the best place to test this is. I've tried using the chrome console and my script wont run in my text editor. Sorry again.
3 Answers
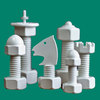
Steven Parker
231,171 PointsYou're close, but there's a few issues:
- setting an interval doesn't do anything if that interval is immediately cleared
- a function calling itself unconditionally (as "getRandomQuote" does) creates an infinite recursion loop
- to call a function (like "getRandomQuote") you must put parentheses after the function name
- the "getRandomQuote" function requires an argument
- the use of the brackets in "printQuote" is not proper syntax, did you mean to use "+" symbols instead?

Dominic Bishop
Full Stack JavaScript Techdegree Graduate 15,302 PointsHello Steven, thank you very much for your help I really appreciate it. I'm sorry for my late reply. I think I have made the necessary corrections. However could I ask you to double check my code before submission? Than you again.
var quotes = [
//Quote 1
{quote: "Better to remain silent and be thought a fool, then to speak out and remove all doubt.",
source: "Abraham Lincoln",
citation: "Speech",
year: "Unknown"},
//Quote 2
{quote: "I am so clever that sometimes I don't understand a single word of what I am saying.",
source: "Oscar Wilde",
citation: "Speech",
year: "1885"},
//Quote 3
{quote: "Success is not final; failure is not fatal: It is the courage to continue that counts.",
source: "Winston Churchill",
citation: "Speech",
year: "1943"},
//Quote 4
{quote: "You have brains in your head. You have feet in your shoes. You can steer yourself any direction you choose.",
source: "Dr. Seuss",
citation: "Book",
year: "1990"},
//Quote 4
{quote: "You have to call me night hawk.",
source: "Step Brothers",
citation: "Movie",
year: "2008"},
//Quote 5
{quote: "It's just a flesh wound.",
source: "Monty python and the holy grail",
citation: "Movie",
year: "2008"},
//Quote 6
{quote: "What is this? A school for ants!",
source: "Zoolander",
citation: "Movie",
year: "2001"},
//Quote 7
{quote: "No, I am your father!",
source: "Darth Vader",
citation: "Movie",
year: "1980"},
//Quote 8
{quote: "Maybe ever’body in the whole damn world is scared of each other.",
source: "John Steinbeck",
citation: "Book",
year: "1937"},
//Quote 9
{quote: "Life is what happens to you while you're busy making other plans.",
source: "John Lennon",
citation: "Speech",
year: "1972"},
//Quote 10
{quote: "Anything worth doing good takes a little chaos.",
source: "Flea",
citation: "Book",
year: "2005"}
];
/*
Here I am creating a function to randomize the colour
of the background.This was taken from my first week of study with
treehouse.
*/
function getRandomColour() {
var red = Math.floor(Math.random() * 256 );
var green = Math.floor(Math.random() * 256 );
var blue = Math.floor(Math.random() * 256 );
var rgbColor = 'rgb(' + red + ',' + green + ',' + blue + ')';
document.body.style.background = rbgColor;
}
/*
I have created two variables to set timers on my
random colour and print function.
*/
var colourTimer = setInterval(getRandomColour, 10000);
var quoteTimer = setInterval(printQuote, 10000);
/*
This function randomly goes through the quotes
array and returns one to the randomQuote variable
*/
function getRandomQuote(quotes) {
var randomQuote = Math.floor(Math.random() * (quotes.length -1));
console.log(getRandomQuote());
return quotes[randomQuote];
}
getRandomQuote(quotes);
function printQuote() {
var randomResult = getRandomQuote;
var htmlMessage = '';
htmlMessage += '<p class="quote">' + randomResult.quote + '</p>'
htmlMessage += '<p class="source">' + randomResult.source + '</p>'
htmlMessage += '<p class="citation">' + randomResult.citation + '</p>'
htmlMessage += '<p class="year">' + randomResult.year + '</p>'
document.getElementById('quote-box').innerHTML = htmlMessage;
}
printQuote();
clearInterval(colourTimer);
clearInterval(quoteTimer);
document.getElementById('load-quote').addEventListener("click", printQuote, false);
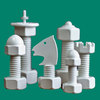
Steven Parker
231,171 PointsIt looks like you implemented my last hint, but the first 4 issues still need fixing.

Dominic Bishop
Full Stack JavaScript Techdegree Graduate 15,302 PointsAhaha sorry Steven, I am poo at this. How embarrassing. I've tried once more but if any of this is wrong please can you just put me out of my misery
var quotes = [
//Quote 1
{quote: "Better to remain silent and be thought a fool, then to speak out and remove all doubt.",
source: "Abraham Lincoln",
citation: "Speech",
year: "Unknown"},
//Quote 2
{quote: "I am so clever that sometimes I don't understand a single word of what I am saying.",
source: "Oscar Wilde",
citation: "Speech",
year: "1885"},
//Quote 3
{quote: "Success is not final; failure is not fatal: It is the courage to continue that counts.",
source: "Winston Churchill",
citation: "Speech",
year: "1943"},
//Quote 4
{quote: "You have brains in your head. You have feet in your shoes. You can steer yourself any direction you choose.",
source: "Dr. Seuss",
citation: "Book",
year: "1990"},
//Quote 4
{quote: "You have to call me night hawk.",
source: "Step Brothers",
citation: "Movie",
year: "2008"},
//Quote 5
{quote: "It's just a flesh wound.",
source: "Monty python and the holy grail",
citation: "Movie",
year: "2008"},
//Quote 6
{quote: "What is this? A school for ants!",
source: "Zoolander",
citation: "Movie",
year: "2001"},
//Quote 7
{quote: "No, I am your father!",
source: "Darth Vader",
citation: "Movie",
year: "1980"},
//Quote 8
{quote: "Maybe ever’body in the whole damn world is scared of each other.",
source: "John Steinbeck",
citation: "Book",
year: "1937"},
//Quote 9
{quote: "Life is what happens to you while you're busy making other plans.",
source: "John Lennon",
citation: "Speech",
year: "1972"},
//Quote 10
{quote: "Anything worth doing good takes a little chaos.",
source: "Flea",
citation: "Book",
year: "2005"}
];
function getRandomColour() {
var red = Math.floor(Math.random() * 256 );
var green = Math.floor(Math.random() * 256 );
var blue = Math.floor(Math.random() * 256 );
var rgbColor = 'rgb(' + red + ',' + green + ',' + blue + ')';
document.body.style.background = rbgColor;
}
var timer;
function colourAndQuoteTimer() {
timer += setInterval(getRandomColour(), 10000);
timer += setInterval(printQuote(), 10000);
}
function clearTimer() {
if (printQuote() && colourAndQuoteTimer()) {
clearInterval(timer);
}
}
function getRandomQuote(array) {
var arrayQuote = Math.floor(Math.random() * (quotes.length));
for (var i = 0; i < quotes.length; i += 1) {
var randomQuoteResult = array[arrayQuote];
}
return randomQuoteResult;
}
getRandomQuote(quotes);
function printQuote() {
var printResult = getRandomQuote(quotes);
var htmlMessage = '';
htmlMessage += '<p class="quote">' + printResult.quote + '</p>'
htmlMessage += '<p class="source">' + printResult.source + '</p>'
htmlMessage += '<p class="citation">' + printResult.citation + '</p>'
htmlMessage += '<p class="year">' + printResult.year + '</p>'
document.getElementById('quote-box').innerHTML = htmlMessage;
}
printQuote();
document.getElementById('load-quote').addEventListener("click", printQuote, false);
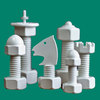
Steven Parker
231,171 PointsLooks like you've got it working now with the button. But check the spelling of "rbgColor", and you've got some new issues with the timers (including that they are never invoked).
You might find it helpful to study the MDN pages on setInterval and clearInterval.
Dominic Bishop
Full Stack JavaScript Techdegree Graduate 15,302 PointsDominic Bishop
Full Stack JavaScript Techdegree Graduate 15,302 Points