Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial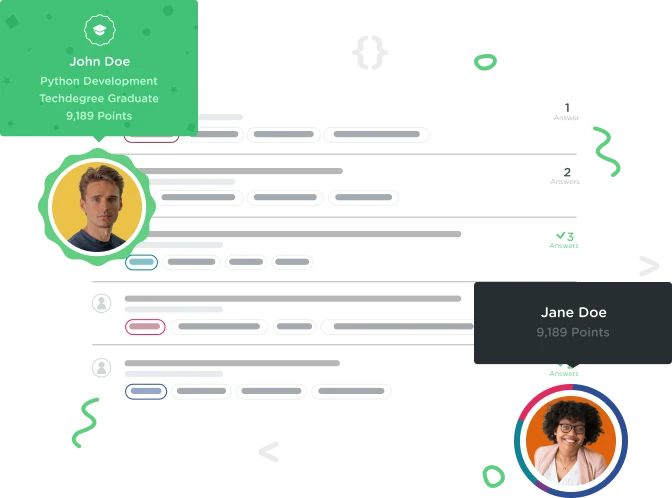

Jason Brown
6,878 Pointsrandom.shuffle crashes when passed a range?!?! >>> some_nums = random.shuffle(range(5,250))
import random some_nums = random.shuffle(range(5,250)) Traceback (most recent call last): File "<stdin>", line 1, in <module> File "/Library/Frameworks/Python.framework/Versions/3.4/lib/python3.4/random.py", line 272, in shuffle x[i], x[j] = x[j], x[i] TypeError: 'range' object does not support item assignment random <module 'random' from '/Library/Frameworks/Python.framework/Versions/3.4/lib/python3.4/random.py'> dir(random) ['BPF', 'LOG4', 'NV_MAGICCONST', 'RECIP_BPF', 'Random', 'SG_MAGICCONST', 'SystemRandom', 'TWOPI', 'BuiltinMethodType', '_MethodType', '_Sequence', '_Set', 'all', 'builtins', 'cached', 'doc', 'file', 'loader', 'name', 'package', 'spec_', '_acos', '_ceil', '_cos', '_e', '_exp', '_inst', '_log', '_pi', '_random', '_sha512', '_sin', '_sqrt', '_test', '_test_generator', '_urandom', '_warn', 'betavariate', 'choice', 'expovariate', 'gammavariate', 'gauss', 'getrandbits', 'getstate', 'lognormvariate', 'normalvariate', 'paretovariate', 'randint', 'random', 'randrange', 'sample', 'seed', 'setstate', 'shuffle', 'triangular', 'uniform', 'vonmisesvariate', 'weibullvariate']
2 Answers

Iain Simmons
Treehouse Moderator 32,305 PointsIt's also because random.shuffle
returns None
, since it actually shuffles a list in place.
So you'd need to use the following:
some_nums = list(range(5,250)) # make sure some_nums is a list/mutable sequence
random.shuffle(some_nums)
You could also use the list comprehension that Alvin McNair suggested, but only to assign to the variable, not as an argument to the random.shuffle
function.
Kenneth Love, perhaps you need another example in your video... even though it was meant to be a group of bad examples!

Alvin McNair
3,398 Pointsrange(start, stop[, step]) Rather than being a function, range is actually an immutable sequence type, as documented in Ranges and Sequence Types — list, tuple, range.
Since its immutable you need to make it a list.
nums=random.shuffle([x for x in range(5,250)])
Kenneth Love
Treehouse Guest TeacherKenneth Love
Treehouse Guest TeacherMaybe so! Lists are just a source of headaches when it comes to functional programming...and yet they're your primary data type.