Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial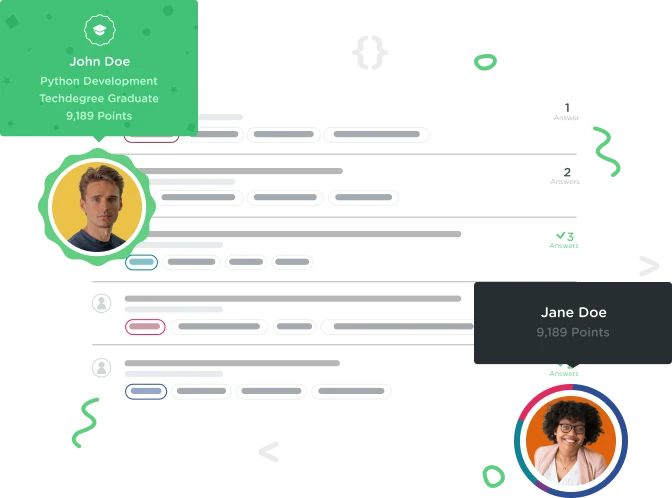

Roger Sullivan
10,992 Pointsreceiving "error Bummer! I entered "bogus". I expected "You entered bogus." but got "You entered ." instead"
for some reason the concatenation in my else statement is not working. i spits out the "You entered ."and ignores the two things after it.
using System;
namespace Treehouse.CodeChallenges
{
class Program
{
static void Main()
{
if ( Console.ReadLine() == "quit")
{
string output = "Goodbye.";
Console.WriteLine(output);
}
else
{
string input = Console.ReadLine();
string output = "You entered " + input + ".";
Console.WriteLine(output);
}
}
}
}
1 Answer
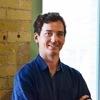
tjgrist
6,553 PointsThat's because you are never taking an input string before you enter the if else blocks. So, it always defaults to else, and then in your code you're asking the user for input with the Console.Readline() in the else block.
This exercise is about scope. You have to declare variables, and in this case get user input before you enter the if else blocks. So, you have to do something like this:
using System;
namespace Treehouse.CodeChallenges
{
class Program
{
static void Main()
{
string input = Console.ReadLine();
string output;
if (input == "quit")
{
output = "Goodbye.";
}
else
{
output = "You entered " + input + ".";
}
Console.WriteLine(output);
}
}
}
Here I am taking the user's input at the top with Readline(), declaring an output variable (but not assigning anything to it), once the code evaluates the if else condition, either "quit" or something else, it will assign a string to output, and that string will be printed. In c# you can declare something and assign it a value later. That is what we are doing here with scope. Console.writeline() at the bottom of my code does not have access to the variables inside the if else blocks, but it does have access to the variables input and output because those were declared outside of the if/else block, (in the same scope as the console.writeline()).