Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial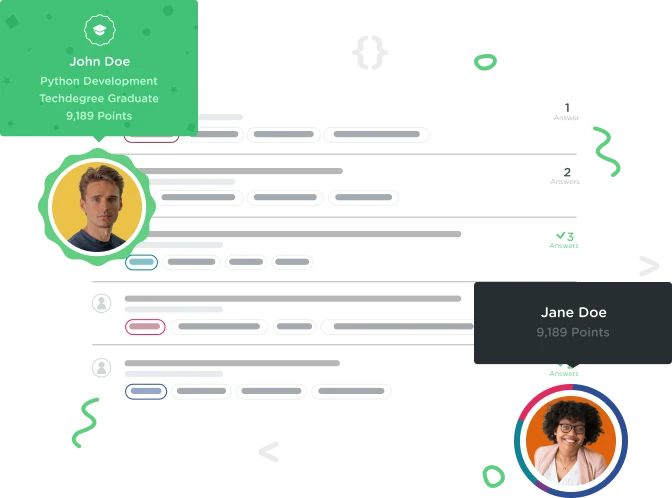

Jeff Hamilton
11,724 PointsRefactor the LoadBirds method by using object initialization to add the pelican to the birds variable.
Keep getting "Did you forget the new keyword?". What am I doing wrong?
using System.Collections.Generic;
using System.Linq;
namespace Treehouse.CodeChallenges
{
public class Bird
{
public string Name { get; set; }
public string Color { get; set; }
public int Sightings { get; set; }
}
}
using System.Collections.Generic;
using System.Linq;
namespace Treehouse.CodeChallenges
{
public static class BirdRepository
{
public List<Bird> birds = new List<Bird>
{
new birds { Name = "Pelican", Color = "White", Sightings = 3 }
}
}
}
3 Answers
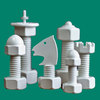
Steven Parker
241,970 Points
It looks like the LoadBirds method is missing.
You still need the method, but you'll change how it works. Your object initialization itself is pretty close, but remember that the pelican is an object of type "Bird" (capital "B", no "s"). You'll also need to retain the method's return statement.
A refactored LoadBirds method might look like this:
public static List<Bird> LoadBirds()
{
var birds = new List<Bird>
{
new Bird { Name = "Pelican", Color = "White", Sightings = 3 }
};
return birds;
}
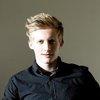
Adam Parish
27,797 PointsJeff,
I totally agree with you the command line vs. code editor is super confusing I wish they would just use visual studio.

Jake Kennard
4,493 PointsI found this a really weird challenge for this point in the course... like wouldn't it be better to use constructors here instead? and what does it have to do with lists?
Jeff Hamilton
11,724 PointsJeff Hamilton
11,724 PointsIt works! Thank you very much for this explanation. I am having difficulties switching between the videos showing how it is done in the command line and typing out the challenges correctly when I get to them.