Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial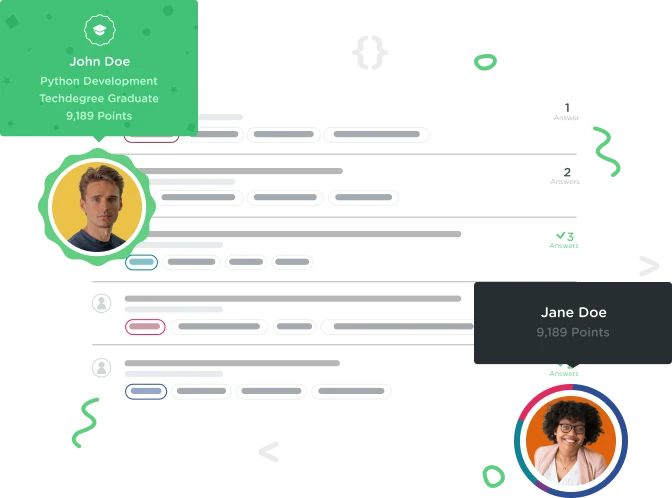

devin blair
4,955 PointsRestarting program rather than exiting when entering an invalid number
I'm trying to make it to where rather than just printing a message and exiting the program it just loops back to the beginning so you don't have to manually restart it but I can't figure it out. I've tried using while loops and using another function but none of it has worked so far.
import math
def split_check(total, number_of_people):
if (number_of_people <= 0) or (total <=0):
raise ValueError("Value cannot be 0 or negative")
return math.ceil(total / number_of_people)
try:
total_due = float(input("What is the total? "))
number_of_people = int(input("How many people "))
amount_due = split_check(total_due, number_of_people)
except ValueError:
print("value cannot be 0 or negative")
else:
print("each person owes ${}".format(amount_due))
1 Answer
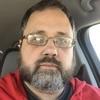
Mark Sebeck
Treehouse Moderator 38,304 PointsGood question Devin. There are many ways to do loops so think of my solution as one of many. I simply made an infinite loop and then break out of the loop when there is a non zero value entered for number of persons.
import math
def split_check(total, number_of_people):
if (number_of_people <= 0) or (total <=0):
raise ValueError("Value cannot be 0 or negative")
return math.ceil(total / number_of_people)
while 1:
try:
total_due = float(input("What is the total? "))
number_of_people = int(input("How many people "))
amount_due = split_check(total_due, number_of_people)
except ValueError:
print("value cannot be 0 or negative")
else:
print("each person owes ${}".format(amount_due))
break
I challenge you to find another way to implement a loop here. Keep at it Devin!
devin blair
4,955 Pointsdevin blair
4,955 PointsI was so close! I was actually putting the break statement in the wrong place, now I'm trying to change it to where it prompts the user "Value cannot be 0 or negative press r to restart or x to exit" so far I've tried this with nested functions, I'll post it if I figure it out thank you for the help.