Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial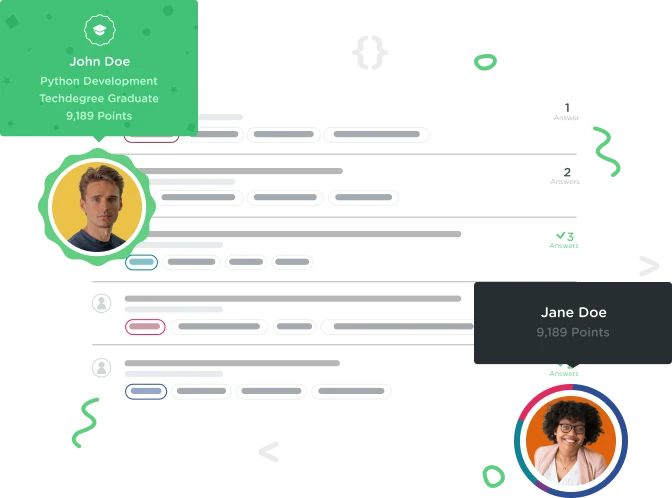
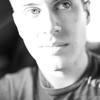
Eric Ewers
13,976 PointsReturn MySQL Query that matches PHP Array
I would like to set a variable equal to an array like so:
$match = array(5000, 5020, 5040);
Then return the values from the database:
mysqli_query ($con,"SELECT * FROM table WHERE Barcode='$match'");
Basically, it should return any combination of numbers in the array, if it exists in the database, and if it's not found then just skip that number and move on to the next.
Any help would be much appreciated! Let me know if I need to elaborate.
1 Answer
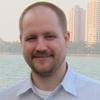
Ryan Field
Courses Plus Student 21,242 PointsHi, Eric. You can do this using an IN()
statement. If you need to use an array specifically, you'll have to turn that array into a comma-separated string, but then you can do this:
<?php
$pdo = new PDO(your database information)
$match = array(5000, 5020, 5040);
$match_list = join(",", $match);
$select = "SELECT * FROM table WHERE Barcode IN (?);";
$statement = $pdo->prepare($select);
$statement->bindParam(1, $match_list);
$statement->execute();
?>
This is using the PDO method, but it's the same idea for mySQLi. You can find more information in this StackOverflow thread. I apologize if there are any mistakes in my code; it's late here and I'm half asleep, but I'm sure you get the idea! XD
Eric Ewers
13,976 PointsEric Ewers
13,976 PointsThanks for your answer, but I think I found a better method what for I need it to do.
Do you happen to know if there are any SQL Injection flaws with this?
Ryan Field
Courses Plus Student 21,242 PointsRyan Field
Courses Plus Student 21,242 PointsSince it's a SELECT query, then there's less danger of having a SQL injection, but as a good practice, you should always sanitize input. The
prepare
method sanitizes your calls for you, and I believe it's also available withmysqli
, so you could use that instead of manual sanitization.