Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial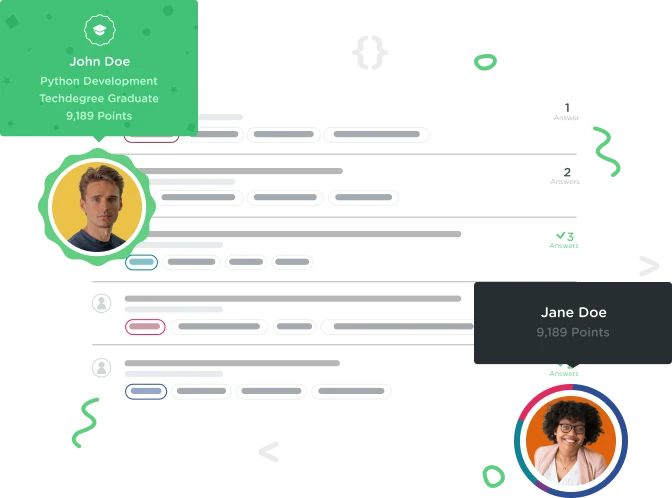

John Weland
42,478 PointsReturning an array of objects form a database in PHP
I am having a huge issue writing out this function to connect to he database return an array of objects and then do an action on those object.
I am using PostgreSQL. I have consulted the documentation for PostgreSQL and PHP as well as looked at other code co-workers have done in a similar vein and I cannot get this to work
<?php
// Connect to database
$conn_string = "host=HOST port=PORT dbname=DATABASE user=USERNAME password=PASSWORD";
$dbconnect = pg_connect ($conn_string) or die ('Could not connect: ' . pg_last_error());
// Get all emails
function sanitizeEmail() {
$sql = "SELECT u.user_acct_nbr,
u.updt_ts,
u.email_id,
u.first_nm,
u.last_nm,
u.btn,
u.user_pin,
u.login,
u.last_login_ts
FROM user_account AS u
WHERE u.email_id IS NOT NULL
LIMIT 5";
$result = pg_query($sql) or die('Query failed: ' . pg_last_error());
$emails = array();
while ( ($row = pg_fetch_object($result) ) !== false) {
$key = $row->email_id;
if (!array_key_exists($key, $emails)) {
$accountObject = array();
$accountObject["accountNumber"] = $row->user_acct_nbr;
$accountObject["lastUpdated"] = $row->updt_ts;
$accountObject["firstName"] = $row->first_nm;
$accountObject["lastName"] = $row->last_nm;
$accountObject["btn"] = $row->btn;
$accountObject["pin"] = $row->user_pin;
$accountObject["login"] = $row->login;
$accountObject["lastLogin"] = $row->last_login_ts;
$accountObject["email"] = $row->email_id;
$emails[$key][] = $accountObject;
}
}
foreach ($emails as $key => $email) {
print_r($email); // Trying to see my returned results
if(filter_var($email, FILTER_VALIDATE_EMAIL) !==false) {
// invalid address
echo "invalid";
} else {
// valid address... continue
echo "valid";
}
}
pg_free_result($result);
pg_close();
}
sanitizeEmail();
?>
While I realize the code below is marked as JSON I did that because the JSON syntax highlighting in the forums works better.
with my print_r($email) statment I was expecting something like
{
[1] : {
userAccount : "####",
lastUpdated : "####",
firstName : "John",
lastName : "Doe",
btn : "###-###-####",
userPin : "####",
login : "XXXXXX",
email : "xxx@example.com"
},
[2] : {
userAccount : "####",
lastUpdated : "####",
firstName : "Jane",
lastName : "Doe",
btn : "###-###-####",
userPin : "####",
login : "XXXXXX",
email : "xxx2@example.com"
},
[3] : {
userAccount : "####",
lastUpdated : "####",
firstName : "Jim",
lastName : "Doe",
btn : "###-###-####",
userPin : "####",
login : "XXXXXX",
email : "xxx3@example.com"
}
}
Instead I get
Array ( [0] => Array ( [accountNumber] => [lastUpdated] => [firstName] => [lastName] => [btn] => [pin] => [login] => [lastLogin] => [email] => ) )
1 Answer
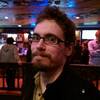
Chris Andruszko
18,392 PointsI don't know if this will help you at all, but I had a similar project a while ago. For part of the plugin, I needed to put an array into a variable, then echo it for each user on the database. I'll try to write a very condensed version of the code I wrote for a part of the plugin. It echos the description for each user on the database. The result looks exactly like you'd want it to.
<?php
//This gets the info
$query = mysqli_query($conn, "SELECT `meta_value` as 'value' FROM `{$userMeta_conn}` WHERE `meta_key` = 'description'") or die(mysqli_error($conn));
//This loops while putting the info into a variable, thus filling in the array
while ($row = mysqli_fetch_assoc($query)) {
$description[] = $row["value"];
}
//Now let's echo the descriptions.
$i = 0;
while($i <= $user_count-1) {
echo $description[$i];
$i++;
}
?>
By the way, I fetched how many users exist on the database and put that number into the #user_count variable (although I didn't include that code here). The result of the code above will echo the user description for each user and stop running when there are no more users to find in the database.
I hope this helps!