Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial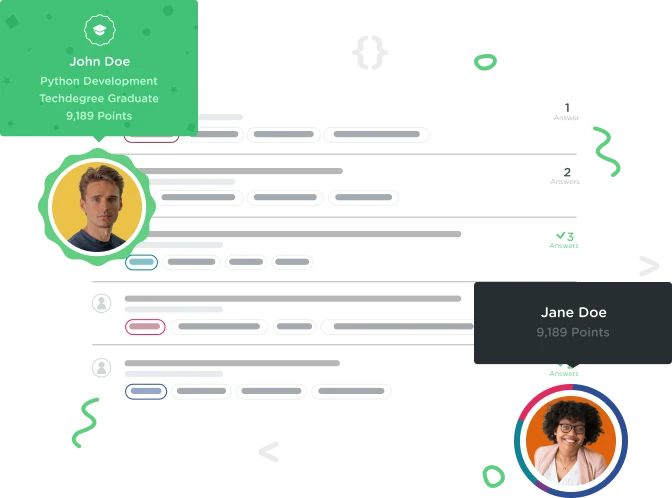
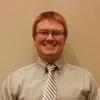
Patrick Shushereba
10,911 PointsReversing String Ruby
I'm trying to reverse a string in Ruby without using the reverse method.
def reverse(string)
reverse_array = []
reverse_array.push(string.split(""))
end
print reverse("This is some input")
I've managed to take the string and split the characters while pushing it into an array. I'm trying to find a way to reverse the characters now. I thought about using the unshift method, but I'm not sure how to implement it. I know I have to iterate over the array, but that's as far as I've gotten. Can anyone provide a small push in the right direction? I'd still like to work towards an answer so I can learn.
5 Answers
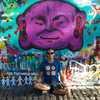
Andrew Stelmach
12,583 PointsNice little problem this.
There are many ways to skin a cat, but I'll give you some hints towards one solution (I've just solved it in irb).
One way to do this, given your array of characters is to setup an 'until' loop. The condition for the loop to end is when a variable, let's call it 'i', gets to a certain value.
'i' is assigned to a value before the loop. That value is derived from the length of your array.
Also before the loop, a new empty array is created, assigned to a variable.
On each cycle, 'i' is increased or decreased (I'll let you work that out).
On each cycle, a character from your original array is 'pushed' into the new empty array. The character is selected by using 'i' to select a character from the original array by its index.
The tricky part is tweaking the initial value of 'i' and the exact condition for when the loop is exited.
This will leave you with a reversed version of your array. You can then use a method to convert that into a string. And voila, you will have a reversed version of your original string.
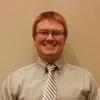
Patrick Shushereba
10,911 PointsThank you for the help,
This is where I'm at so far:
def reverse(string)
temp_array = []
reverse_array = []
i = string.length
temp_array.push(string.split(""))
until i == 0
reverse_array.push(temp_array[i])
i -= 1
end
end
print reverse("This is some input")
I can't seem to get it to run appropriately. I'm going to keep looking for my error. When I inspect the array, it's not pushing the reversed characters. I'm getting nil for all of the indices in the array.
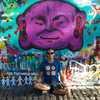
Andrew Stelmach
12,583 PointsHave you tried testing your code? i.e. running it? Even if you're on Windows you can install Ruby and run irb. Well, actually, I think you can just do that in Workspaces. I solved it by writing the code in irb, but it's a bit more fiddly that way.
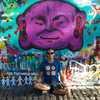
Andrew Stelmach
12,583 PointsBe mindful of your indentation. Not only does it make it more readable if it's properly indented, but it also helps you understand what's going on i.e. what is nested inside what. I've reposted the exact same code here, with proper indentation. It's important to get into the habit early, because it is very important and a hard habit to break further down the line.
def reverse(string)
temp_array = []
reverse_array = []
i = string.length
temp_array.push(string.split(""))
until i == 0
reverse_array.push(temp_array[i])
i -= 1
end
end
print reverse("This is some input")
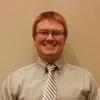
Patrick Shushereba
10,911 PointsI have tried running the code, and when it runs, all of the values in the reverse_array return as nil. I know it's not pushing the letters, but I'm not sure why.
EDIT:
I've managed to get the string to reverse. At first I wasn't iterating over the array like I was supposed to. Then when I discovered that, shortly after I realized that I wasn't creating a placeholder in between the || pipes. But I solved that and it worked. Now I'm just trying to join the string back together.
This is the code I have so far:
def reverse(string)
temp_array = []
reverse_array = []
i = string.length
temp_array.push(string.split(""))
temp_array.each do |item|
until i < 0
reverse_array.push(item[i])
i -= 1
end
#print reverse_array.inspect
end
end
print reverse("This is some input")
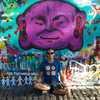
Andrew Stelmach
12,583 PointsGood work on getting this far, Patrick; nearly there! The best thing you can do now is google "ruby convert array to string" OR (preferred and actually easier in this case): google "ruby array methods" and click on the top listing. That will take you to the Ruby docs. Have a look through the methods on that page and you should find something suitable. Post here when you've solved it!
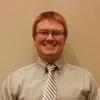
Patrick Shushereba
10,911 Pointsdef reverse(string)
temp_array = []
reverse_array = []
i = string.length
temp_array.push(string.split(""))
temp_array.each do |item|
until i < 0
reverse_array.push(item[i])
i -= 1
end
#print reverse_array.inspect
end
reverse_array.join
end
#print reverse("This is some input")
# These are tests to check that your code is working. After writing
# your solution, they should all print true.
puts(
'reverse("abc") == "cba": ' + (reverse("abc") == "cba").to_s
)
puts(
'reverse("a") == "a": ' + (reverse("a") == "a").to_s
)
puts(
'reverse("") == "": ' + (reverse("") == "").to_s
)
This is what I ended up with. I figured that the join method was the easiest (only?) way to return a string. Thank you again for all of your help. I'm trying to learn how to program so that I can apply for a coding bootcamp like App Academy, or a program like LaunchCode.
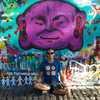
Andrew Stelmach
12,583 PointsYes the join method is by far the simplest solution. Good work, it's persisting like this that helps you. I'm on a 12 week coding bootcamp in London as we speak - Makers Academy.