Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial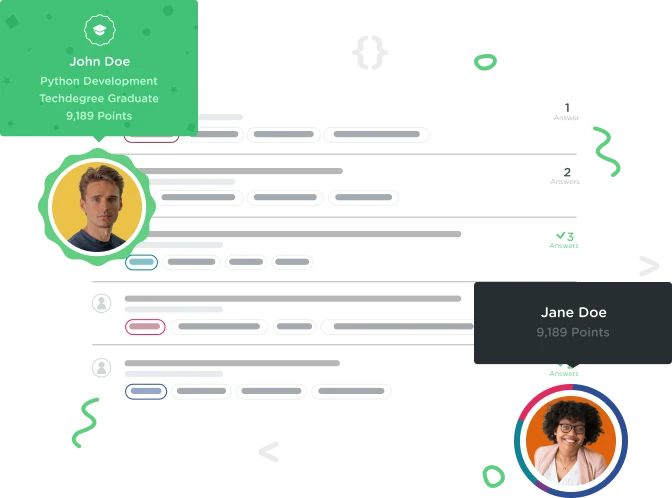
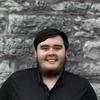
Michael Hulet
47,913 PointsRuby Extra Credit Help
For the extra credit of "Build a Bank Account Class" in "Ruby Objects and Classes", we are asked to rewrite the balance
method of the BankAccount
class we had written in the course to use the inject
method. inject
takes a block, which in turn takes 2 parameters: an accumulator variable and the object it is currently operating on, like this:
(5..10).inject do |sum, n|
sum + n
end
#In the end, sum will be 45, and inject will return that
The balance
method we wrote during the course looks like this:
def balance
value = 0
@transactions.each do |transaction|
value += transaction[:amount]
end
return value
end
@transactions
is an array of hashes, which might look something like this:
{description: "Paycheck", amount: 100}
Each hash in the @transactions
array has a description, which is supposed to list the purpose of the transaction, and it also holds the amount of that transaction. The goal of the balance
method is to iterate over all the hashes in the @transactions
array and add together all the amounts. Here's my attempt at doing it with the inject
method, like the Extra Credit asks:
def balance
@transactions.inject do |sum, transaction|
sum[:amount] + transaction[:amount]
end
end
However, this returns the following error & stack trace:
bank.rb:19:in `[]': no implicit conversion of Symbol into Integer (TypeError)
from bank.rb:19:in `block in balance'
from bank.rb:18:in `each'
from bank.rb:18:in `inject'
from bank.rb:18:in `balance'
from bank.rb:30:in `print_register'
from bank.rb:36:in `<main>'
How can I adapt the balance
method to properly use inject
?
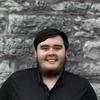
Michael Hulet
47,913 PointsHeck yeah! I'm up in Hendersonville. How about you?
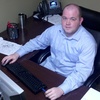
Christopher Loyd
Courses Plus Student 5,806 PointsI live in Smyrna and work in Nashville :)
1 Answer

Clayton Perszyk
Treehouse Moderator 48,863 PointsHey Michael,
You can set the initial value of the accumulator variable when you invoke inject. Here's the example you provided, but using an initial value for sum:
(5..10).inject(5) do |sum, n|
sum + n
end
# sum will be set to 5 initially and each n will be added to sum giving it a final value of 50
You can do something similar with your balance method by setting sum to zero.
hopefully this helps,
Clayton
Also, you wont need to access :amount with sum.
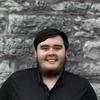
Michael Hulet
47,913 PointsOh, that makes so much sense! Thanks for the help!

Clayton Perszyk
Treehouse Moderator 48,863 PointsGlad I could help.
Christopher Loyd
Courses Plus Student 5,806 PointsChristopher Loyd
Courses Plus Student 5,806 PointsGlad to see Nashville being represented! (Sorry, I don't know Ruby).