Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial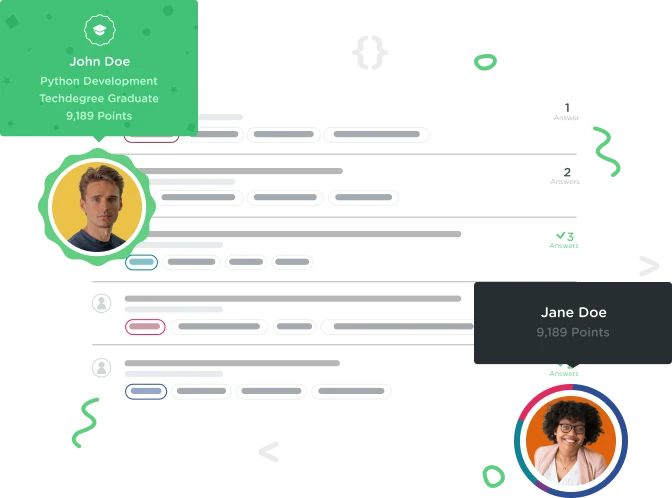
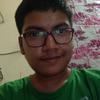
Abirbhav Goswami
15,450 PointsRuby program giving TONS of errors!
I tried building a Ruby app to create a To Do list by myself as the Treehouse server was down. The program ran fine until a few minutes ago, as now it shows tons of different errors. I'll put in the files I used so someone could help me out.
ToDoList.rb:
require "./ToDoItem.rb"
def printSeparator(char = "-")
puts char * 40
end
class ToDoList
attr_reader :name
attr_accessor :items
def initialize(name)
@name = name
@items = []
end
def addItems(description)
@items.push(ToDoItem.new(description))
end
def run
choice = ""
puts "To Do List: #{@name}"
printSeparator()
while choice != "quit"
puts "add: Adds new items"
puts "check: Check every task to see if it's complete"
puts "print: Prints the to do list to the screen."
puts "quit: Quits the program."
print "What do you want to do? "
choice = gets.chomp.downcase
case choice
when "add"
print "What item do you want to add? "
addItems(gets.chomp)
when "print"
printList()
when "quit"
exit
end
end
end
def isComplete?
@items.each do |item|
print "Is this item complete? (y/n) #{item.description} "
choice = gets.chomp.downcase
if choice == "n"
puts "Okay. Be sure to complete it soon!"
elsif choice == "y"
item.complete
puts "Good job! Onwards!"
else
puts "Invalid option selected. Try again"
end
end
end
def printList
puts @name
printSeparator()
@items.each do |item|
puts "To Do: #{item.description}"
end
printSeparator()
end
end
toDoList = ToDoList.new("My list")
# toDoList.addItems("Get groceries")
# toDoList.addItems("Solve 3 papers")
# toDoList.printList
# toDoList.isComplete?
toDoList.run
ToDoItem.rb:
class ToDoItem
attr_reader :description
attr_reader :complete
def initialize(description)
@description = description
@complete = false
end
def complete
@complete = true
end
end
I know I'm using camelCase, and Ruby mostly uses underscore_case, but I am from a Java background, and I find it more comfortable to work with.
The errors I'm getting are as follows:
abbygriffiths@abbygriffiths:~/Programming/Ruby/ToDoList$ ruby ToDoList.rb
ToDoList.rb:28: syntax error, unexpected tIDENTIFIER, expecting keyword_end
puts "print: Prints the to do list to the screen."
^
ToDoList.rb:28: syntax error, unexpected tSTRING_BEG
ToDoList.rb:29: syntax error, unexpected tIDENTIFIER, expecting keyword_end
puts "quit: Quits the program."
^
ToDoList.rb:29: syntax error, unexpected tSTRING_BEG
ToDoList.rb:32: syntax error, unexpected tCONSTANT, expecting keyword_end
print "What do you want to do? "
^
ToDoList.rb:36: syntax error, unexpected tIDENTIFIER, expecting keyword_end
when "add"
^
ToDoList.rb:37: syntax error, unexpected tCONSTANT, expecting keyword_end
print "What item do you want to add? "
^
ToDoList.rb:40: syntax error, unexpected tIDENTIFIER, expecting keyword_end
when "print"
^
ToDoList.rb:43: syntax error, unexpected tIDENTIFIER, expecting keyword_end
when "quit"
^
ToDoList.rb:51: syntax error, unexpected tCONSTANT, expecting keyword_end
print "Is this item complete? (y/n) #{item.description} "
^
ToDoList.rb:68: syntax error, unexpected keyword_do_block, expecting keyword_end
@items.each do |item|
^
ToDoList.rb:69: syntax error, unexpected tSTRING_BEG, expecting keyword_do or '{' or '
('
puts "To Do: #{item.description}"
^
ToDoList.rb:84: syntax error, unexpected $end, expecting keyword_end
toDoList.run
^
abbygriffiths@abbygriffiths:~/Programming/Ruby/ToDoList$
1 Answer
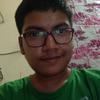
Abirbhav Goswami
15,450 PointsGot it. Nothing wrong with the program. I'd forgotten to save the file before running. My bad! Rookie mistake!