Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial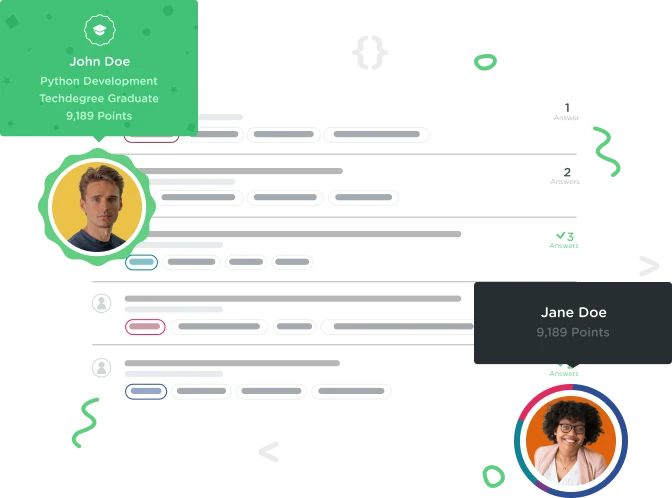

Ryley Ameden
1,590 PointsRunning a function if certain parameters within said function are met, if not re-run the function.
Good day everyone,
I was hoping someone might be able to assist me with a predicament I am having. I am looking to create a function that does the following: (sudo)
- query the database of all authors pertaining to a certain category
- return a random author ONLY if the author has all of his information filled out (IE: Name, Picture, Description)
- If the above dependencies are met print out this information, if not get another random author.
I have worked with PHP in the past but for some reason I just can't wrap my head around this.
Any help is welcome!
Thanks so much.
1 Answer
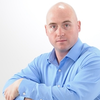
Benjamin Payne
8,142 PointsHey Ryley,
I am fan of recursion so here is what i would do. Not sure of your exact setup so convert this to your appropriate ORM or whatever you're using:
<?php
/**
* Fetch a random author if all required fields have been filled out.
*
* @param array $required_fields
* @return mixed
*/
function fetchRandomAuthor($required_fields)
{
$authors = ORM::query('authors')->where('category', 'non-fiction')->get();
$author = $authors[array_rand($authors, 1)];
foreach ($required_fields as $required_field) {
if (empty($author[$required_field]) || ! isset($author[$required_field])) {
return fetchRandomAuthor($required_fields);
}
}
return $author;
}
For the check if the fields are filled, this should be done dynamically by passing in a required_fields
array that can be updated outside of the application, most likely from a database table. That way if more or less fields become required down the road you can simply update that table.
Also there might be a more efficient way of comparing the required fields array with the author than a loop, but I'll leave that for you to explore ;).
Hopefully that gets you down the road.
Thanks,
Ben