Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial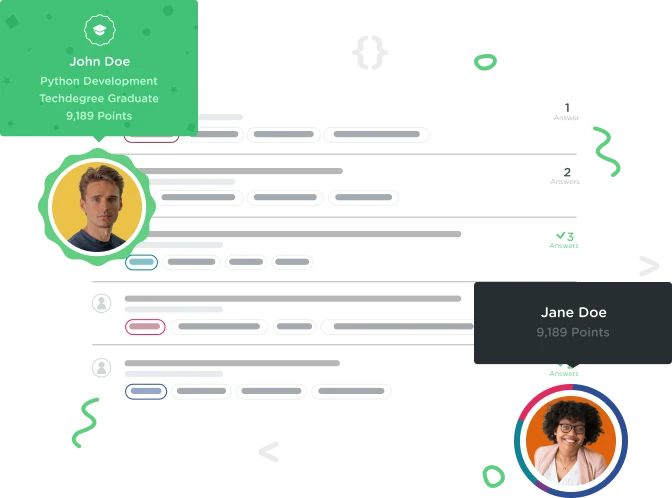

James Mackey
Courses Plus Student 994 PointsSays drive is already defined?
line 37
public class GoKart {
public static final int MAX_BARS = 8;
private String mColor;
private int mBarsCount;
public GoKart(String color) {
mColor = color;
mBarsCount = 0;
}
public String getColor() {
return mColor;
}
public void drive() {
drive(1);
}
public void drive(int laps) {
// Other driving code omitted for clarity purposes
mBarsCount -= laps;
}
public void charge() {
while (!isFullyCharged()) {
mBarsCount++;
}
}
public boolean isBatteryEmpty() {
return mBarsCount == 0;
}
public boolean isFullyCharged() {
return mBarsCount == MAX_BARS;
}
public void drive(int lapAmount) {
int newAmount = mBarsCount + lapAmount;
if (newAmount > MAX_BARS) {
throw new IllegalArguementException("Not enough battery remains");
}
mBarsCount = newAmount;
}
}
3 Answers

Simon Coates
28,694 Pointscan have multiple methods with the same name, but they have to have different signatures, so that java knows which one you mean. You have two methods called drive that accept an int. It has no means to differentiating. You have at least one other mistake. IllegalArgumentException

James Mackey
Courses Plus Student 994 Pointspublic void drive(int laps) { if (mBarsCount - laps < 0) {
throw new IllegalArgumentException("Not enough battery remains");
} else {
mBarsCount -= laps;
} } } I have tried to differentiate and change my methods. Im now here. I know I hav the same problem. Im stuck and hav no idea how to fix it

Simon Coates
28,694 Pointsi pass that challenge with:
public class GoKart {
public static final int MAX_BARS = 8;
private String mColor;
private int mBarsCount;
public GoKart(String color) {
mColor = color;
mBarsCount = 0;
}
public String getColor() {
return mColor;
}
public void drive() {
drive(1);
}
public void drive(int laps) {
if (mBarsCount - laps < 0) {
throw new IllegalArgumentException("Not enough battery remains");
}
mBarsCount -= laps;
}
public void charge() {
while (!isFullyCharged()) {
mBarsCount++;
}
}
public boolean isBatteryEmpty() {
return mBarsCount == 0;
}
public boolean isFullyCharged() {
return mBarsCount == MAX_BARS;
}
}
James Mackey
Courses Plus Student 994 PointsJames Mackey
Courses Plus Student 994 PointsHave I not differentiated drive with int(lapAmount)??
Simon Coates
28,694 PointsSimon Coates
28,694 Pointsno. the signature has to be unique enough for java to know which method you mean on the basis of name and the number/type of parameters. As you have two drive methods that accept a single int, it has no method of knowing which one you want.