Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial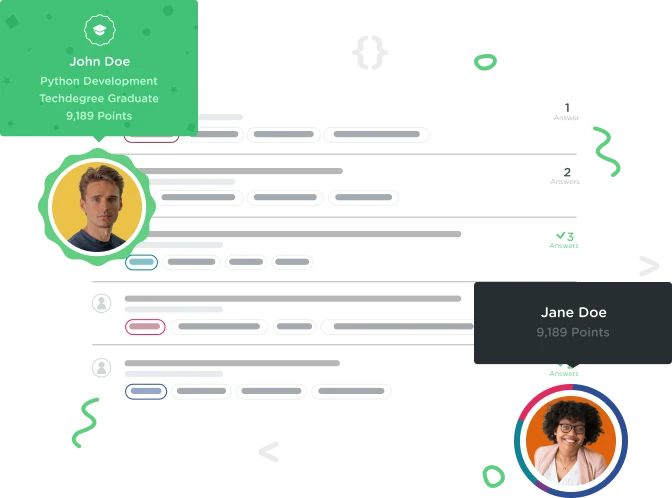

ana ana
945 PointsScrabblePlayer
What is wrong with this:
So back to that ScrabblePlayer. I found that it's not enough to know if they just have a tile of a specific character. We need to know how many they actually have. Can you please add a method called getTileCount that uses the for each loop you just learned to loop through the characters and increment a counter if it matches? Return the count, please, thanks!
public class ScrabblePlayer {
private String mHand;
private int count = 0 ;
public ScrabblePlayer() {
mHand = "";
}
public String getHand() {
return mHand;
}
public void addTile(char tile) {
// Adds the tile to the hand of the player
mHand += tile;
}
public boolean hasTiler(char tile) {
return mHand.indexOf(tile) > -1;
}
public int getTileCount(char tile) {
for (char znak : mHand.toCharArray()) {
if (znak == tile) {count++;}
}
return count;
}
}
8 Answers

ana ana
945 PointsBut in our case getTileCount(char tile) method is accessing variables of class, and it belongs to class ScrabblePlayer class ? It should be no problem for that method which belongs to class to access to members of that class.
can you provide some web link with more details about that

Gagandeep Virk
19,741 PointsTry this public int getTileCount(char tile) { int count = 0; for(char letter : mHand.toCharArray()) { if(letter == tile) { count++; } } return count; }
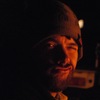
Preston Skaggs
11,818 PointsYou need to use the "for each" loop instead of the "for" loop.

ana ana
945 Pointsfor (char znak : mHand.toCharArray()) { if (znak == tile) {count++;} }
is this for each loop cause they used this in previous lecture video
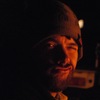
Preston Skaggs
11,818 PointsYes, sorry I haven't used Java in a while. It could have to do with you returning a private member variable from a public method.
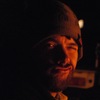
Preston Skaggs
11,818 PointsYou could try this
public int getTileCount(char tile){
String newHand = getHand();
int count = 0;
for( char znak : newHand.toCharArray()){
if(znak == tile){
count++;
}
}
return count;
}

ana ana
945 PointsAnd what about returning a private member variable from a public method, is that allowed in Java and java script?
does variable count need to bi declared in getTileCount method, or it can be declared in class.
and why did you use String newHand = getHand();, mHand is private variabe, I understamd that, but getTileCount is located in class, so it has access to vartiable of main class even it is private, right ?
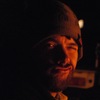
Preston Skaggs
11,818 PointsPrivate member variables are only accessible in their own class. Public variables are accessible outside the class. So when you create an instance of a class you are only able to access variables or methods that are public. This is why you create getter and setter methods for private member variables i.e. getHand(). You should read a little about Encapsulation to understand why this is done.
In short to use private class variables outside the class you need to use getter and setter methods. That is why I declared count in the getTileCount() method. If other methods needed the count you could create a private class variable and then created accessor methods like getCount() increaseCount() or decreaseCount().

ana ana
945 PointsYes, but public int getTileCount(char tile) is located inside public class ScrabblePlayer class, so it should have access to all members of ScrabblePlayer, no matter they are private or public, right ?
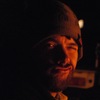
Preston Skaggs
11,818 PointsYes, but when you instantiate your class to use in your program you are only allowed to access public methods and variables.

Craig Dennis
Treehouse TeacherAna, just keep the count variable local instead of as a property of the class. It doesn't make sense to expose it there, and I am calling the method multiple times during my testing, so your count isn't resetting. Set it to zero then increment in the method.
Hope it helps!
Preston Skaggs
11,818 PointsPreston Skaggs
11,818 PointsSo you can read about Encapsulation here: http://www.tutorialspoint.com/java/java_encapsulation.htm
I may have misspoke before, I think the issue was you were accessing mHand outside the class by using .toCharArray(). Your use of the private variable count was ok.