Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial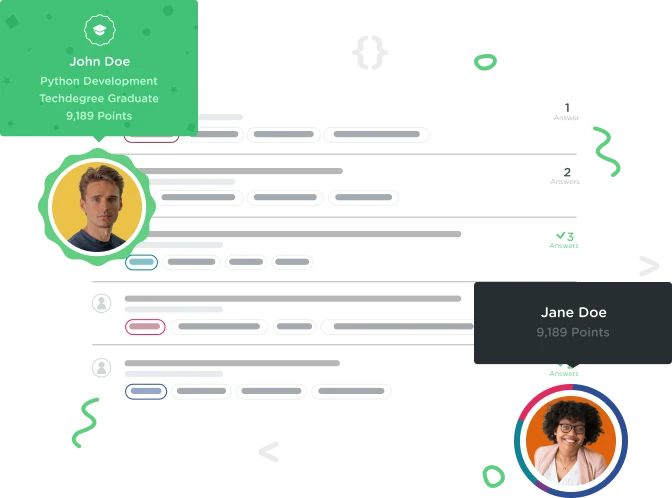

Devin Gray
39,261 PointsSendmail won't submit. Page won't even load. Slim v3.
I have been racking my brain all night trying to figure out how to get this form to work with Slim v3. My email variable keeps getting recognized as empty because I don't know how to pass in the value from the form. my redirect variables aren't working for the same reason. When I enter the values manually I get Fatal error: Call to a member function withHeader() on a non-object when it seemed to be working just fine to redirect pages earlier in the lesson. Has anyone finished this project correctly? I'd love to know how.
<?php
<?php
use \Psr\Http\Message\ServerRequestInterface as Request;
use \Psr\Http\Message\ResponseInterface as Response;
require 'vendor/autoload.php';
date_default_timezone_set('America/Los_angeles');
use Monolog\Logger;
use Monolog\Handler\StreamHandler;
// $log = new Logger('name');
// $log->pushHandler(new Monolog\Handler\StreamHandler('app.log', Logger::WARNING));
// $log->addWarning('Zoinks');
// Configure App Settings
$configuration = [
'settings' => [
'displayErrorDetails' => true,
],
];
$c = new \Slim\Container($configuration);
// Create App
$app = new \Slim\App($c);
// Get container
$container = $app->getContainer();
// Register component on container
$container['view'] = function ($c) {
$view = new \Slim\Views\Twig('templates', [
'cache' => false
]);
// Instantiate and add Slim specific extension
$basePath = rtrim(str_ireplace('index.php', '', $c['request']->getUri()->getBasePath()), '/');
$view->addExtension(new Slim\Views\TwigExtension($c['router'], $basePath));
return $view;
};
// Render Twig template in route
$app->get('/', function ($request, $response, $args) {
return $this->view->render($response, 'about.twig', [
'name' => $args['name']
]);
})->setName('about');
$app->get('/contact', function ($request, $response, $args) {
return $this->view->render($response, 'contact.twig', [
'name' => $args['name']
]);
})->setName('contact');
$app->post('/contact', function ($request, $response, $args) {
$body = $this->request->getParsedBody();
$name = $body['name'];
$email = $body['email'];
$msg = $body['msg'];
$uri = $request->getUri();
$basePath = $body['index'];
if(!empty($name) && !empty($email) && !empty($msg)) {
$cleanName = filter_var($name, FILTER_SANATIZE_STRING);
$cleanEmail = filter_var($email, FILTER_SANATIZE_EMAIL);
$cleanMsg = filter_var($msg, FILTER_SANATIZE_STRING);
} else {
//message the user that there was a problem
return $response->withHeader('Location', $uri);
}
});
// Swiftmailer setup
// Create the Transport
$transport = Swift_MailTransport::newInstance();
// Create the Mailer using your created Transport
$mailer = \Swift_Mailer::newInstance($transport);
// Create a message
$message = Swift_Message::newInstance()
->setSubject('Thank you for your input')
->setFrom(array($cleanEmail => $cleanName))
->setTo(array('devin@devingray.me', 'devin.gray92@gmail.com' => 'Devin Gray'))
->setBody($cleanMsg);
// Send the message
if(!$mailer->send($message, $errors)) {
echo "Error: " . $logger->dump();
} else {
$result = $mailer->send($message);
}
// After the message sends
if ($result > 0) {
// send a message that says thank you
return $response->withHeader('Location', $basePath);
} else {
// send a message to the user that the message failed to send
// log that there was an error
return $response->withHeader('Location', $uri);
}
// Run app
$app->run();
2 Answers

Devin Gray
39,261 PointsI figured out my biggest problem was that I was running Swift Mailer outside of my $app->post function. The pages work but I never got the flash message or anything else working that I wanted, but I did get it to do everything the project asked of me.
I'll try to find solutions to the other problems later.
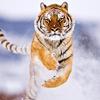
Shaun Moore
6,301 PointsNot sure if this will help much but you have two opening tags :-)
<?php
<?php

Devin Gray
39,261 PointsWhoops, I added it because I've been posting code snippits a lot and posting the php syntax doesnt work unless you have the <?php tag open. Basically I forgot that I already had one on my index.php page and added another one. There's only one on my actual page.