Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial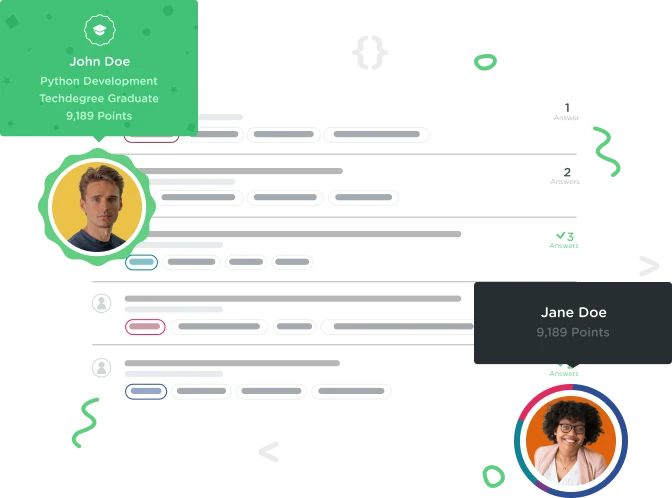
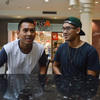
Stephen Chamroeun
7,095 PointsSharing my code
alert(`Let's do some math!`);
const num1 = prompt('Please provide a number.');
const num2 = prompt('Please provide another number.');
const input1 = parseInt(num1);
const input2 = parseInt(num2);
let message = `<h1>Math with the numbers ${num1} and ${num2}</h1><br>
${input1} + ${input2} = ${input1 + input2}<br>
${input1} * ${input2} = ${input1 * input2}<br>
${input1} / ${input2} = ${input1 / input2}<br>
${input1} - ${input2} = ${input1 - input2}<br>`;
document.write(message);
The code works. I just wanted feedback to see if the code could be refactored? Thank you!
2 Answers
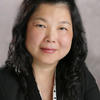
Julia Lam (Daly)
5,230 PointsHi Stephen,
Your code looks great.
I have two suggestions:
- indent `<h1>Math with the numbers ${num1} and ${num2}</h1><br> .
- remove <br> after </h1> . Unless you need extra line break. ```
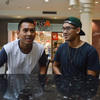
Stephen Chamroeun
7,095 PointsI was in the zone and overlooked that <br> haha. Thank you for spotting that. I shall make that correction.
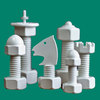
Steven Parker
231,153 PointsGood job! And just for fun...
A purely optional but cute trick is to take advantage of the fact that any math done with NaN will return NaN:
if (isNaN(input1 + input2)) {
And since you have template literals, another cute trick with them for much more compact code:
document.write(`<h1>Math with the numbers ${num1} and ${num2}</h1>`);
for (let c of "+*/-") // use a loop to do all 4 operations
document.write(`${input1} ${c} ${input2} = ${eval(`input1 ${c} input2`)}<br>`);
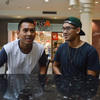
Stephen Chamroeun
7,095 Pointsahhhhh, I shall try this. Thank you for that. I really appreciate it!
Stephen Chamroeun
7,095 PointsStephen Chamroeun
7,095 PointsI've made a few quick edits. Dave had mentioned the NaN method towards the end of the video, so I included that too. Thank you to whomever reviews my code!