Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial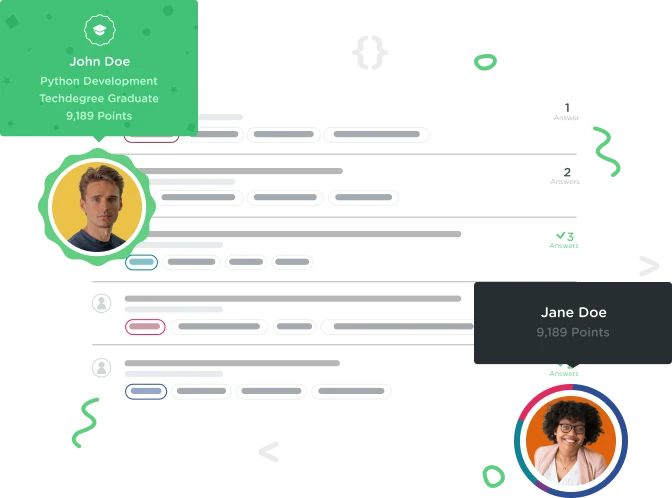

APRIL GOLDEN
812 PointsShopping Cart Challenge
Still having problems with Shopping Cart challenge. Can Someone tell me what I'm still doing wrong?
public class Example {
public static void main(String[] args) {
System.out.printf("Adding %d of %s to the cart. %n", quantity, item, getName());
/* Other code omitted for clarity*/
}
ShoppingCart cart = new ShoppingCart();
Product pez = new Product("Cherry PEZ refill (12 pieces)");
cart.addItem =(PEZ, 5);
/* Since a quantity of 1 is such a common argument when adding a product to the cart,
* your fellow developers have asked you to make the following code work, as well as keeping
* the ability to add a product and a quantity.
*/
Product dispenser = new Product("Yoda PEZ dispenser");
/* Uncomment the line following this comment,
after adding a new method using method signatures,
to solve their request in ShoppingCart.java
*/
cart.addItem(dispenser);
}
public class ShoppingCart {
public void addItem(Product item, int quantity) {
System.out.printf("Adding %d of %s to the cart.%n", quantity, item, getName());
/* Other code omitted for clarity. Please imagine*/
}
public String getName(){
return mName;
}
public void addItem(Product item){
addItem(item, 1);
}
}
public class Product {
/* Other code omitted for clarity, but you could imagine
it would store price, options like size and color
*/
private String mName;
public Product(String name) {
mName = name;
}
public String getName() {
return mName;
}
public void addItem(Product addedItem){
addItem(addedItem, 1);
}
2 Answers
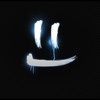
Grigorij Schleifer
10,365 PointsHey April,
you are already done ...
You created two addItem methods
Delete this code in the Product class, you do´t need it there
public void addItem(Product addedItem){
addItem(addedItem, 1);
}
Then delete the getName method from the ShopingCart method. You already have this method inside the Product class (see above)
Inside the ShoppingCart class your code should then look like this (without the getName method)
// first addItem method
public void addItem(Product item, int quantity) {
System.out.printf("Adding %d of %s to the cart.%n", quantity, item.getName());
/* Other code omitted for clarity */
}
// New overloaded method
public void addItem(Product item) {
// Call the original addItem method from the overloaded method, and give them the Product item and the quantity
addItem(item, 1);
}
And don´t forget to change this and in the example class
cart.addItem =(PEZ, 5);
to
// Your instance of the Product class is pez not PEZ (Product pez = new Product("Cherry PEZ refill (12 pieces));
// So give this parameter ( same as Product item ) to your first addItem method
cart.addItem =(pez, 5);
Try to put everything together :) If you need help, don´t hesitate to shout out :)
Grigorij
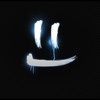
Grigorij Schleifer
10,365 PointsHi APRIL,
I found that you need an extra semicolon for your main-method.
public class Example {
public static void main(String[] args) {
System.out.printf("Adding %d of %s to the cart. %n", quantity, item, getName());
/* Other code omitted for clarity*/
}
ShoppingCart cart = new ShoppingCart();
Product pez = new Product("Cherry PEZ refill (12 pieces)");
cart.addItem =(PEZ, 5);
/* Since a quantity of 1 is such a common argument when adding a product to the cart,
* your fellow developers have asked you to make the following code work, as well as keeping
* the ability to add a product and a quantity.
*/
Product dispenser = new Product("Yoda PEZ dispenser");
/* Uncomment the line following this comment,
after adding a new method using method signatures,
to solve their request in ShoppingCart.java
*/
cart.addItem(dispenser);
// an extra semicolon to complete the main method
}
}
In the code below you create an object pez of the Product class. But you add a uppercased PEZ to your cart. This seems to be wrong to te.
Product pez = new Product("Cherry PEZ refill (12 pieces)");
cart.addItem =(PEZ, 5);
Please add your error message and let me know if I am wrong ...
Grigorij
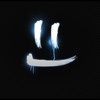
Grigorij Schleifer
10,365 PointsHey April,
have you figured it out?
I found one another possible error:
public String getName() {
return mName;
}
The getName method is called twice ... in the Product class, and in the ShoppingCart class :)

APRIL GOLDEN
812 PointsHi Grigoriji! It didn't matter if I added a semi-colon, it still came up with a bunch of errors!