Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial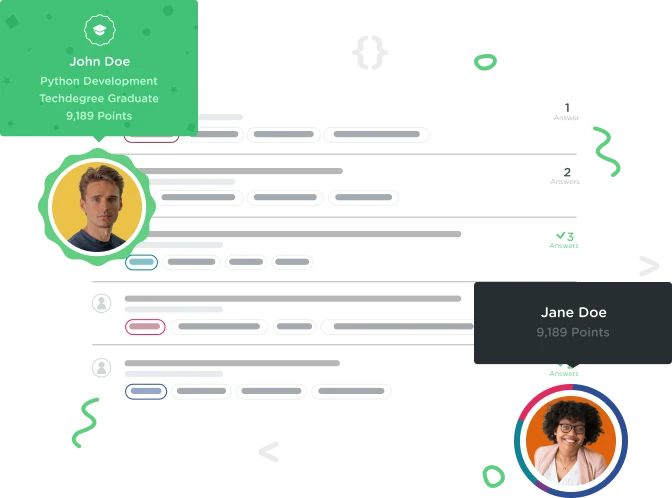
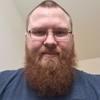
Henrik Christensen
Python Web Development Techdegree Student 38,322 Pointssign up?
Hi,
I have been creating sign up and log in scripts a couple of times now (for practice) and I always tend to do something similar every time, and now I'm wondering if it's "okay" or if it's awfull? :-)
Any input is appreciated :-)
sign up script
<?php
if ($_SERVER['REQUEST_METHOD'] == 'POST') {
// getting data entered by the user
// filter and sanitize the data
$username = trim(filter_input(INPUT_POST, 'username', FILTER_SANITIZE_STRING));
$email = trim(filter_input(INPUT_POST, 'email', FILTER_SANITIZE_EMAIL));
$password = filter_input(INPUT_POST, 'password', FILTER_SANITIZE_STRING);
$confirmPassword = filter_input(INPUT_POST, 'confirmpassword', FILTER_SANITIZE_STRING);
// checking for empty fields
if (empty($username) || empty($email) || empty($password) || empty($confirmPassword)) {
$error_message = 'All fields are required.';
header('location: signup?error=' . urlencode($error_message));
die();
}
// checking if password and confirmPassword are identical
if ($password !== $confirmPassword) {
$error_message = 'Please confirm your password.';
header('location: signup?error=' . urlencode($error_message));
die();
}
// if no empty fields and passwords are matching
if ($password == $confirmPassword && !empty($username) && !empty($email)) {
// require bootstrap for database connection
require(__DIR__ . '/bootstrap.php');
require(__DIR__ . '/classes/User.php');
$user = new User($pdo);
// checking if username already exist in database
if ($user->getByUsername($username)) {
$error_message = 'There is already a user with that name.';
header('location: signup?error=' . urlencode($error_message));
die();
} else if (!$user->getByUsername($username)) {
// username does not exist in database
// checking if email already exist in database
if ($user->getByEmail($email)) {
$error_message = 'Email is already in use.';
header('location: signup?error=' . urlencode($error_message));
die();
} else if (!$user->getByEmail($email)) {
// email does not exist in database
// hashing password
$hashed_password = password_hash($password, PASSWORD_DEFAULT);
if ($user->create($username, $email, $hashed_password)) {
// user has successfully been created
header('location: /');
die();
} else {
$error_message = 'Something went wrong.';
header('location: signup?error=' . urlencode($error_message));
die();
}
} else {
// something went wrong
$error_message = 'Something went wrong.';
header('location: signup?error=' . urlencode($error_message));
die();
}
} else {
// something went wrong
$error_message = 'Something went wrong.';
header('location: signup?error=' . urlencode($error_message));
die();
}
}
}
1 Answer
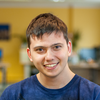
Konrad Traczyk
22,287 PointsHello Henrik, at first glance.
You could make function for this redirect because it repeats 8 times
<?php
function error_message($message) {
if($message){
$error_message = $message;
header('location: signup?error=' . urlencode($error_message));
die();
} else {
header('location: /');
die();
}
}
?>
Also i see you're using twice $user->getByEmail($email) and $user->getByUsername($username) what probably queries your database twice, you could write this to variable, and put this variable to your if statemantes what would make only one query, so your script have better performance.