Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial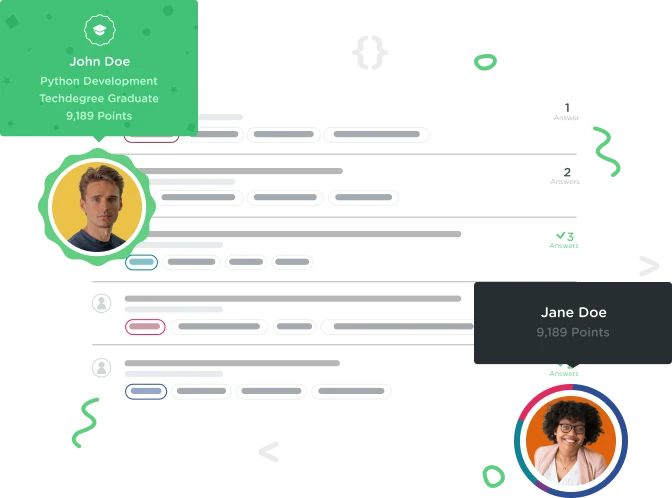

Iden Rosenthal
797 PointsSimple Debug Question <identifier expected>
I'm getting an error <identifier expected> on the following code which as far as I can tell I'm 99% sure was just copied directly from the video tutorial on building an interactive story app.
Here is my code:
`
package com.idenrosenthal.interactivestory.ui;
import com.idenrosenthal.interactivestory.R;
import com.idenrosenthal.interactivestory.model.Choice;
import com.idenrosenthal.interactivestory.model.Page;
public class Story {
private Page[] pages;
** ERROR HERE <identifier> expected : pages = new Page[7]; **
^
pages[0] = new Page(R.drawable.page0,
R.string.page0,
new Choice (R.string.page0_choice1, 1),
new Choice(R.string.page0_choice2), 2);
pages[1] = new Page(R.drawable.page1,
R.string.page1,
new Choice(R.string.page1_choice1, 3),
new Choice(R.string.page1_choice2, 4));
pages[2] = new Page(R.drawable.page2,
R.string.page2,
new Choice(R.string.page2_choice1, 4),
new Choice(R.string.page2_choice2, 6));
pages[3] = new Page(R.drawable.page3,
R.string.page3,
new Choice(R.string.page3_choice1, 4),
new Choice(R.string.page3_choice2, 5));
pages[4] = new Page(R.drawable.page4,
R.string.page4,
new Choice(R.string.page4_choice1, 5),
new Choice(R.string.page4_choice2, 6));
pages[5] = new Page(R.drawable.page5, R.string.page5);
pages[6] = new Page(R.drawable.page6, R.string.page6);
public Page getPage(int pageNumber) {
if (pageNumber >= pages.length) {
. I pageNumber = 0;
}
return pages[pageNumber];
}
}
Possibly it has something to do with the Page class declarations. I don't understand why Page is declare twice but I think this is way it is done in the tutorial see this:
package com.idenrosenthal.interactivestory.model;
public class Page {
private int imageId;
private int textID;
private Choice choice1;
private Choice choice2;
private boolean isFinalPage = false;
public Page(int imageId, int textID) {
this.imageId = imageId;
this.textID = textID;
this.isFinalPage = true;
}
public Page(int imageId, int textID, Choice choice1, Choice choice2) {
this.imageId = imageId;
this.textID = textID;
this.choice1 = choice1;
this.choice2 = choice2;
}
public boolean isFinalPage() {
return isFinalPage;
}
public void setFinalPage(boolean finalPage) {
isFinalPage = finalPage;
}
public int getImageId() {
return imageId;
}
public void setImageId(int imageId) {
this.imageId = imageId;
}
public int getTextID() {
return textID;
}
public void setTextID(int textID) {
this.textID = textID;
}
public Choice getChoice1() {
return choice1;
}
public void setChoice1(Choice choice1) {
this.choice1 = choice1;
}
public Choice getChoice2() {
return choice2;
}
public void setChoice2(Choice choice2) {
this.choice2 = choice2;
}
}
8 Answers
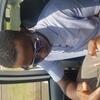
Malcom T.L Musuma
11,363 PointsHey Iden. Go to your Choice class that is in Choice.java and make sure the constructor takes in 2 parameters of type int. It should look like👇.
public Choice(int textId, int nextPage) {
this.textId = textId;
this.nextPage = nextPage;
}
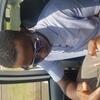
Malcom T.L Musuma
11,363 Pointspublic class Story {
private Page []pages;
public Story() {
pages = new Page[7];
pages[0] = new Page(R.drawable.page0 ,
R.string.page0 ,
new Choice(R.string.page0_choice1 , 1),
new Choice(R.string.page0_choice2 , 2));
pages[1] = new Page(R.drawable.page1,
R.string.page1,
new Choice(R.string.page1_choice1, 3),
new Choice(R.string.page1_choice2, 4));
pages[2] = new Page(R.drawable.page2,
R.string.page2,
new Choice(R.string.page2_choice1, 4),
new Choice(R.string.page2_choice2, 6));
pages[3] = new Page(R.drawable.page3,
R.string.page3,
new Choice(R.string.page3_choice1, 4),
new Choice(R.string.page3_choice2, 5));
pages[4] = new Page(R.drawable.page4,
R.string.page4,
new Choice(R.string.page4_choice1, 5),
new Choice(R.string.page4_choice2, 6));
pages[5] = new Page(R.drawable.page5, R.string.page5);
pages[6] = new Page(R.drawable.page6, R.string.page6);
}
public Page getPage(int pageNumber) {
if (pageNumber>=pages.length){
pageNumber=0;
}
return pages[pageNumber];
}
}
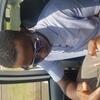
Malcom T.L Musuma
11,363 PointsHello mate! Check carefully on the third line of my code. Your code is missing the constructor for story i.e
public Story() {pages = new Page[7]; //and the rest of the code}.
After creating the story class, there is need for a constructor from which the pages will be initialized. Hope that helps!
Happy coding :)

Iden Rosenthal
797 PointsThanks so much for your assistance! Now if I may be so forward as to ask for help with the next error that arose after I fixed the one you pointed out is this:
!https://gardenofiden.com/Screenshot%20from%20Android%20Studio.png "'.class' expected"
the code is
private void loadPage(int pageNumber) {
Page page = story.getPage(int pageNumber);
Drawable image = ContextCompat.getDrawable(this, page.getImageId());
storyImageView.setImageDrawable(image);
String pageText = getString(page.getTextID());
pageText = String.format(pageText, name);
storyTextView.setText(pageText);
choice1Button.setText(page.getChoice1().getTextId());
choice2Button.setText(page.getChoice1().getTextId());
}
the highlighted expression is pageNumber and the error is '.class' expected followed by another error reading ';' expected there is no mising ';' for sure. Is there a problem how I'm calling story.getPage ?
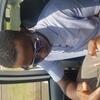
Malcom T.L Musuma
11,363 PointsHello im not sure what you are asking for but you can try to rewrite you second line to - Page page = story.getPage(pageNumber); Hint: remove the int variable

Iden Rosenthal
797 PointsI took out the offending int and now my error has changed to:
screenshot: !https://gardenofiden.com/Screenshot2020-09-16.png
It appears to be telling me I am giving arguments to new Choice where no arguments are required. Very puzzling. I'm definitely copying the video closely but getting weird errors.
the code for Choice.java is:
package com.idenrosenthal.interactivestory.model;
public class Choice {
private int textId;
private int nextPage;
public int getTextId() {
return textId;
}
public void setTextId(int textId) {
this.textId = textId;
}
public int getNextPage() {
return nextPage;
}
public void setNextPage(int nextPage) {
this.nextPage = nextPage;
}
}
and the code for Story.java where the Choice.java is called is:
package com.idenrosenthal.interactivestory.ui;
import com.idenrosenthal.interactivestory.R;
import com.idenrosenthal.interactivestory.model.Choice;
import com.idenrosenthal.interactivestory.model.Page;
public class Story {
private Page []pages;
public Story() {
pages = new Page[7];
pages[0] = new Page(R.drawable.page0 ,
R.string.page0 ,
// 1st error here:
new Choice(R.string.page0_choice1 , 1),
new Choice(R.string.page0_choice2 , 2));
pages[1] = new Page(R.drawable.page1,
R.string.page1,
new Choice(R.string.page1_choice1, 3),
new Choice(R.string.page1_choice2, 4));
pages[2] = new Page(R.drawable.page2,
R.string.page2,
new Choice(R.string.page2_choice1, 4),
new Choice(R.string.page2_choice2, 6));
pages[3] = new Page(R.drawable.page3,
R.string.page3,
new Choice(R.string.page3_choice1, 4),
new Choice(R.string.page3_choice2, 5));
pages[4] = new Page(R.drawable.page4,
R.string.page4,
new Choice(R.string.page4_choice1, 5),
new Choice(R.string.page4_choice2, 6));
pages[5] = new Page(R.drawable.page5, R.string.page5);
pages[6] = new Page(R.drawable.page6, R.string.page6);
}
public Page getPage(int pageNumber) {
if (pageNumber>=pages.length){
pageNumber=0;
}
return pages[pageNumber];
}
}
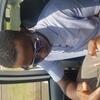
Malcom T.L Musuma
11,363 PointsHello, can you sent a screenshot of the error and the file from which the error is originating from

Iden Rosenthal
797 Pointshttps://gardenofiden.com/Screenshot2020-09-16.png is a screen shot of the error with the code it points to.
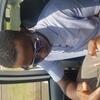
Malcom T.L Musuma
11,363 PointsHope it worked!!!