Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial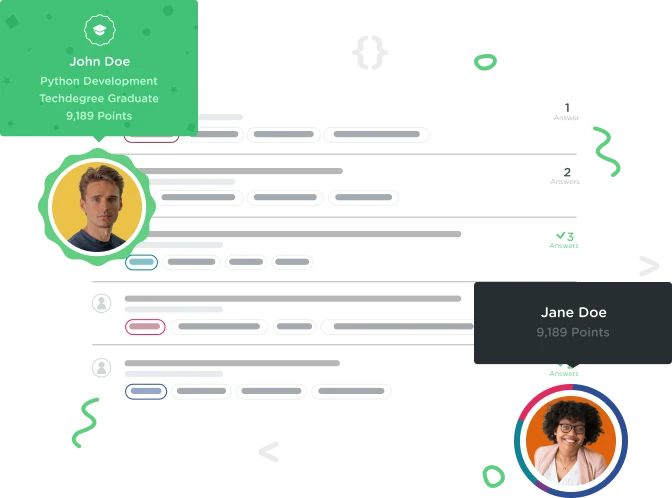

Aaron Arkie
5,345 Points[SOLVED]Stumped on this challenge.
I have modeled an assistant for a tech conference. Since there are so many attendees, registration is broken up into two lines. Lines are determined by last name, and they will end up in line 1 or line 2. Please help me fix the getLineFor method.
Can anyone lend a hand? Dont want answers just a guide to a correct one. thanks in advance!
public class ConferenceRegistrationAssistant {
public int getLineFor(String lastName) {
/* If the last name is between A thru M send them to line 1
Otherwise send them to line 2 */
int line = 0;
return line;
}
}

boog690
8,987 PointsYou're on the right track. Why are you comparing 'A' to 'M'? Maybe we should compare something else to 'M'?

Aaron Arkie
5,345 PointsI was also given :
NEW INFO: chars can be compared using the > and < symbols. For instance 'B' > 'A' and 'R' < 'Z'

Aaron Arkie
5,345 PointsDo you mean substitute something else for M?

boog690
8,987 PointsThat's right. Characters can be compared to other characters. Booleans will be returned based on their position in the English alphabet.
Again, perhaps 'A' shouldn't be what we use to place attendees on a specific line. The exercise asks us to assign line number based on the first letter of the last name.

Aaron Arkie
5,345 PointsSo if a is the first letter of the english alphabet is its value = 1? So if I said
If('A' <13) { Line = 1; }
would that be okay? Also does the if statement recognize 'A' as a char or do I have to state its type in the statement? I appreciate your patience and time for your help.

boog690
8,987 PointsYou don't have to use numbers here. Java knows 'A' is a char.
We want to use the first letter of the String lastName. You saved this char in your variable letter. Why not test if THAT letter is less than 'M'. If it's less than 'M', we do one thing. Otherwise, we do another.

Aaron Arkie
5,345 PointsAwesome thanks! When I get home ill try it out! Thanks again!
EDIT* So as you plainly laid it out i followed your instructions however i am still not getting it here is my code.
public class ConferenceRegistrationAssistant {
public int getLineFor(String lastName) {
/* If the last name is between A thru M send them to line 1
Otherwise send them to line 2 */
int line = 0;
char letter = lastName.indexOf(0);
if(letter < 'M'){
line = 1;
}
if('M' > letter){
line = 2;
}
return line;
}
}

Aaron Arkie
5,345 PointsOkay i got it. i had lastName.indexOf(); when it should read lastName.charAt(). Wrong method! thanks again!

boog690
8,987 PointsGreat! Glad you got it! I didn't catch that you used the wrong method, either. Sorry.
6 Answers
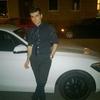
Cristiano Monteiro
5,942 Pointspublic class ConferenceRegistrationAssistant {
public int getLineFor(String lastName) {
int line = 0;
if(lastName.charAt(0) < 'M'){
line = 1;
} else {
line = 2;
}
return line;
}
}
```

Andrei Villasana
4,244 PointsThis is what I used~ And it worked perfectly fine.
public class ConferenceRegistrationAssistant {
public int getLineFor(String lastName) {
/* If the last name is between A thru M send them to line 1
Otherwise send them to line 2 */
int line = 0;
char lastNameChar = lastName.charAt(0);
if (lastNameChar <= 'M') {
line = 1;
}
else {
line = 2;
}
return (line);
}
}

tapiwa chauruka
2,502 Pointsi did the same too and it worked. thanx
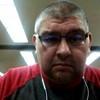
micram1001
33,833 Pointschar letter = lastName.charAt(0); not char letter = lastName.indexOf(0);

Hugo Davis
1,234 Pointsif(letter < 'N')
not: if(letter < 'M')
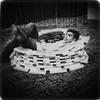
Saad Khan Malik
25,199 Pointspublic class ConferenceRegistrationAssistant {
public int getLineFor(String lastName) {
/* If the last name is between A thru M send them to line 1
Otherwise send them to line 2 */
int line = 0;
char letter = lastName.indexAt(0);
if(letter < 'M'){
line = 1;
} else {
line = 2;
}
return line;
}
}

Charles Robinson
4,152 PointsTo clean up your code a little bit you could take out the if statement for the second line and just put else like this:
public class ConferenceRegistrationAssistant {
public int getLineFor(String lastName) {
/* If the last name is between A thru M send them to line 1
Otherwise send them to line 2 */
int line = 0;
char letter = lastName.indexOf(0);
if(letter < 'M'){
line = 1;
} else {
line = 2;
}
return line;
}
}
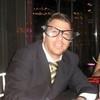
Gonzalo Torres del Fierro
Courses Plus Student 16,751 Pointspublic class ConferenceRegistrationAssistant {
public int getLineFor(String lastName) { /* If the last name is between A thru M send them to line 1 Otherwise send them to line 2 */ int line; if(lastName.charAt(0)<='M'){ line = 1; } else { line =2; }return line; } }
Aaron Arkie
5,345 PointsAaron Arkie
5,345 PointsAm I on the right track?