Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial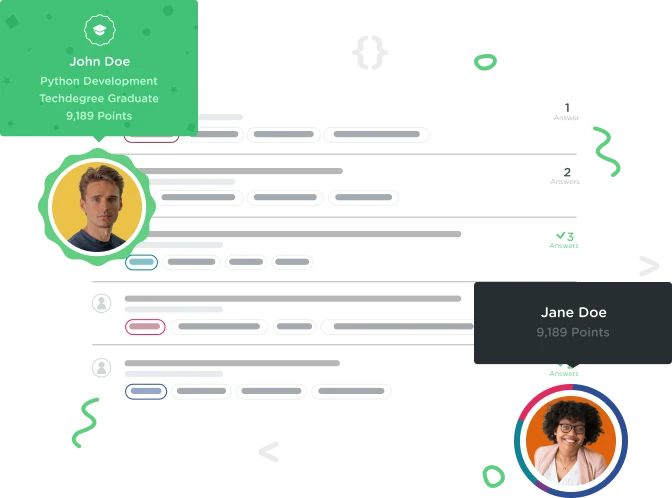
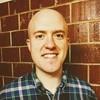
Brian Barrow
6,925 PointsSorting Lists IComparer Interface
For the extra exercise in Sorting Lists video it is asking that we implement the IComparer<T> interface. I have tried but I haven't been able to figure it out. This is what I have for my code so far:
using System;
using System.Collections.Generic;
namespace Treehouse
{
class Student : IComparer<Student>
{
public string Name { get; set; }
public int GradeLevel { get; set; }
public int Compare(Student x, Student y)
{
int result = x.Name.CompareTo(y.Name);
if(result == 0)
{
result = x.GradeLevel.CompareTo(y.GradeLevel);
}
return result;
}
}
}
I am getting the "System.InvalidOperationException: No IComparable<T> or IComparable interface found for type 'Treehouse.Student'." error and can't figure out why.
2 Answers
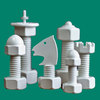
Steven Parker
231,124 PointsYou have to explicitly provide a Comparer to Sort.
The issue is on line 17 of Program.cs, where you call the Sort method with no arguments. Called that way, Sort relies on the objects being sorted to have implemented the IComparable
interface. But since you implemented IComparer
instead, you have to give Sort the comparer to use. You can give it any of your existing objects or create a new one:
students.Sort(students[0]); // use existing object as the comparer
// ...or...
students.Sort(new Student()); // create a new one to compare with
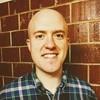
Brian Barrow
6,925 PointsSo it doesn't matter which student I pass in? Why is that?
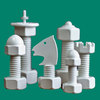
Steven Parker
231,124 PointsBecause it doesn't get data from it (it gets that from the array), it only uses it for the Compare method it implements.
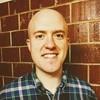
Brian Barrow
6,925 PointsOkay, thanks. Just trying to sort (no pun intended) it out in my head. So whatever I pass in is basically telling the Sort function what type of thing it will be comparing? Like an example to start things out?
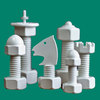
Steven Parker
231,124 PointsIt knows what kind of thing it is comparing, remember the error message you got before? What this does is tell it how to compare things of that type.
This was good for an exercise, but practically it would probably be better to just implement IComparable and then you can use Sort without the argument.
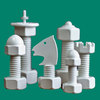
Steven Parker
231,124 PointsIt compiles without errors for me.
I'm guessing there's more to the project than just this module. Perhaps you should make a snapshot of your workspace and post the link to it here.
Then describe exactly what steps (and what commands) you use to see the error.
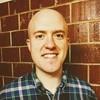
Brian Barrow
6,925 PointsHere is the snapshot. I didn't know I could do that. https://w.trhou.se/5nzbn9niqn
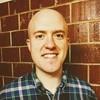
Brian Barrow
6,925 PointsI ran the mcs *.cs
and then the mono Program.exe
to run it. That is when I got the error.
Antonio De Rose
20,885 PointsAntonio De Rose
20,885 PointsCan you direct me where this exercise is in Treehouse.