Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial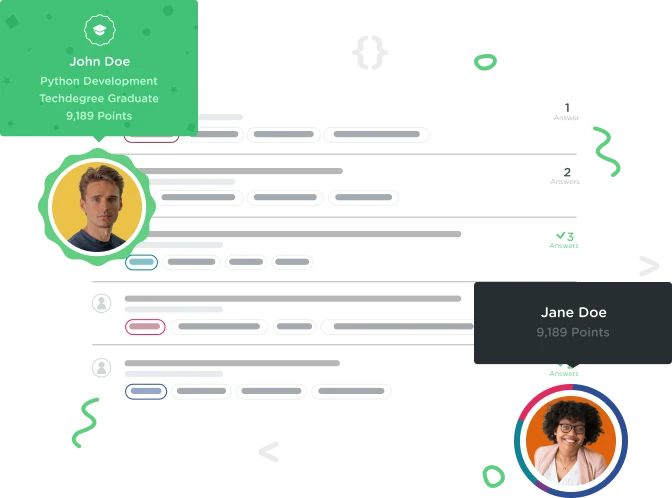
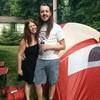
Carter Pringle
3,416 PointsStage 4 exercise question
This should work, yet it doesn't. I mean it checks if the name is correct, and if so it returns it, otherwise it returns failed. There's no code errors, but it says "Expected firstName to fail, but it passed." Or something along those lines. This question is seriously getting on my nerves!
public class TeacherAssistant {
public static String validatedFieldName(String fieldName) {
try {
if (fieldName.startsWith("m")) {
if (Character.isUpperCase(fieldName.charAt(1))) {
return fieldName;
}
}
} catch (IllegalArgumentException iae) {
}
return "failed";
}
}
2 Answers
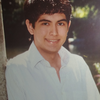
Jon Kussmann
Courses Plus Student 7,254 PointsIt's a little tricky, but the question is asking you to throw an exception, not try to catch it.
Modifying your code a little...
if (fieldName.startsWith("m") && Character.isUpperCase(fieldName.charAt(1))) {
return fieldName;
} else {
throw new IllegalArgumentException("Invalid name");
}

Jess Sanders
12,086 Pointsprivate char validateGuess(char letter) {
if (!Character.isLetter(letter)) {
throw new IllegalArgumentException("A letter is required."
}
letter = Character.toLowerCase(letter);
if (mMisses.indexOf(letter) >= 0 || mHits.indexOf(letter) >= 0) {
throw new IllegalArgumentException(letter + " has already been guessed") }
return letter;
}
- The above code is the example to follow.
- We care about charAt for this challenge, rather than indexOf
- In pseudocode, we want to check for the following condition, and throw an error: " if the character at index 0 of fieldName does not equal 'm' OR the character at index 1 of fieldName is not upper case"
Carter Pringle
3,416 PointsCarter Pringle
3,416 PointsAwesome, thanks a bunch!
Youngchul Kim
4,258 PointsYoungchul Kim
4,258 PointsJon, could you briefly explain why I must use Character.isUpperCase in this scenario? Why do I get an error when using fieldName.isUpperCase?
Jon Kussmann
Courses Plus Student 7,254 PointsJon Kussmann
Courses Plus Student 7,254 PointsSure,
The isUpperCase method is a static method. This means that it is called by the class, and not any one specific object of that class.
fieldName is a string and it does not have a method that you can give an index to see if it is upper case or not (it's just not built into Java.
The Character class does have such method, all you need to do is give it a char and it will tell you if it's uppercase or not.
(You give it a char, by getting the character from the 1 position of the string)
Let me know if anything needs more explanation.