Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial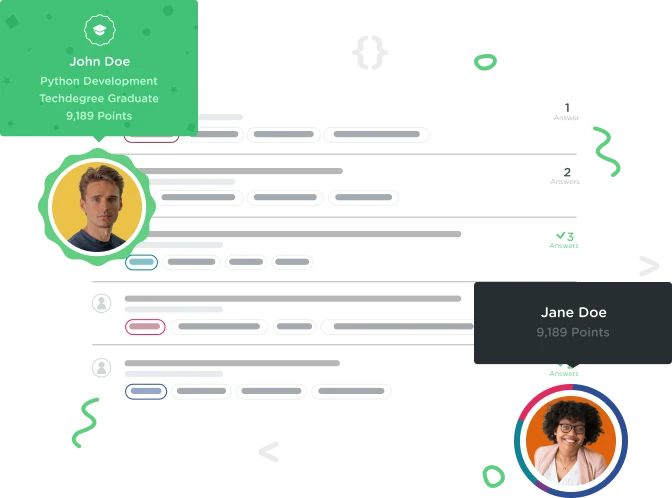
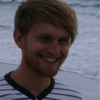
Daniel Schwemmlein
4,960 PointsStill no solution in sight with task 2/4 of the java challenge
Tried to clearify the private fields like suggested from a fellow user. Nothing helped. Its under user.java. They say I have to add getters. Should I just add getmFirstName and getmLastName?
public class ForumPost {
private User mAuthor;
private String mTitle;
private String mDescription;
public User getAuthor() {
return mAuthor;
}
public String getTitle() {
return mTitle;
}
// TODO: We need to expose the description
}
public class User {
private String mSomeName;
mSomeName = someName;//what type do we need? What name would be more appropriate?
private String mAnotherName;
mAnotherName = anotherName;
public User(String firstName, String lastName) {
// TODO: Set the private fields here
}
}
public class Forum {
private String mTopic;
public String getTopic() {
return mTopic;
}
public Forum(String topic) {
mTopic = topic;
}
public void addPost(ForumPost post) {
/* When all is ready uncomment this...
System.out.printf("New post from %s %s about %s.\n",
post.getAuthor().getFirstName(),
post.getAuthor().getLastName(),
post.getTitle());
*/
}
}
public class Example {
public static void main(String[] args) {
System.out.println("Starting forum example...");
if (args.length < 2) {
System.out.println("first and last name are required. eg: java Example Craig Dennis");
}
// Forum forum = new Forum("Java");
// Take the first two elements passed args
// User author = new User();
// Add the author, title and description
// ForumPost post = new ForumPost();
// forum.addPost(post);
}
}
3 Answers
Christopher Augg
21,223 PointsHey Daniel,
Sorry you are having these issues. I will try and go over this step by step with you.
The instructions say:
"Okay, so let's work on the User object now. I really didn't get too far. Can you please add the private fields to store the parameters defined in the constructor? Ooh while you're at it can you add the appropriate getters? Thanks!"
We only need to work on the User object for 2/4 of the challenge. We are provided:
public class User {
public User(String firstName, String lastName) {
// TODO: Set the private fields here
}
}
We need to add private fields. Notice that the TYPE of the firstName and lastName arguments being passed into the constructor are a String. Therefore, we will need to make our private variables a String TYPE. Also, we should name these variables similar to what is being passed into the constructor and consider a good naming convention like using mFirstName / mLastName:
public class User {
private String mFirstName; //we make it a String type : TYPE and mSomeName was a hint
private String mLastName; //we also make this one a String type : TYPE and mAnotherName was also a Hint
public User(String firstName, String lastName) {
// TODO: Set the private fields here
mFirstName = firstName; //we are assigning firstName to mFirstName
mLastName = lastName; //we are assigning lastName to mLastName
}
}
Now we just need some get methods: Spoiler: This is working code. Please attempt the challenge before using.
public class User {
private String mFirstName; //we make it a String type : TYPE and mSomeName was a hint
private String mLastName; //we also make this one a String type : TYPE and mAnotherName was also a Hint
public User(String firstName, String lastName) {
// TODO: Set the private fields here
mFirstName = firstName; //we are assigning firstName to mFirstName
mLastName = lastName; //we are assigning lastName to mLastName
}
// TYPE , getSomeName(), and mSomeName were all hints.
// We will also need a String TYPE to return because we are returning mFirstName and it is a String
public String getFirstName() {
return mFirstName;
}
// TYPE , getAnotherName(), and mAnotherName were all hints
public String getLastName() {
return mLastName;
}
}
I would recommend going back through the videos and practicing. It is important to understand these concepts before moving forward. Another thing I like to do is keep a notebook and take notes when watching the videos. And the extra credit exercises are very good for practicing the concepts learned.
Regards,
Chris

Sandy Woods
1,082 PointsI don't think the challenge was difficult, I believe it was just the wording (like it always is with me that is). I guess he's stating these things in plain English and wrapping my brain around translating that into java code is still a bit tough
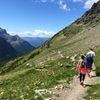
Stephen Wall
Courses Plus Student 27,294 PointsYou need to declare your member variables above the methods so they are within the scope of the class. Then you can set the member variables to the signature variables of the constructor, then you can create the getter methods like so:
public class User {
private String mFirstName; // First need to declare your private member variables here
private String mLastName;
public User(String firstName, String lastName) {
mFirstName = firstName; // Then you can add them to the constructor here
mLastName = lastName;
}
public String getFirstName(){ // Then worry about the getters methods
return mFirstName; // for the above member variables.
}
public String getLastName(){
return mLastName;
}
}