Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial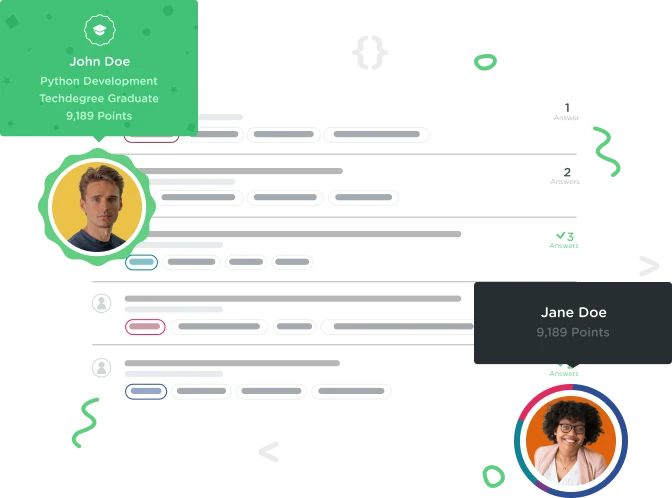
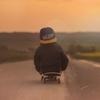
Nipi Tiri
2,988 PointsStill syntax, wtf?
I cant do this :(
6 Answers
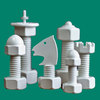
Steven Parker
231,269 PointsYou fixed the problem with 'input', but you still need to fix the issues with 'output'. Here's your code again, with comments added:
using System;
namespace Treehouse.CodeChallenges
{
class Program
{
static void Main()
{
string input = Console.ReadLine(); // good job! - you fixed the first error
// since 'output' is needed below, it should probably be declared HERE
if (input == "quit")
{
string output = "Goodbye."; // notice that 'output' is being declared as a
// string here inside the conditional block!
}
else
{
string output = "You entered " + input + "."; // 'output' is also being declared
// here inside this block!
}
Console.WriteLine(output);
}
}
}
So to fix the 2nd problem, you need a new declaration of 'output' before the 'if', and you must convert the two places where it is currently both declared and assigned into just simple assignments.
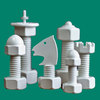
Steven Parker
231,269 PointsAs hinted at above, this challenge is about declarations, assignments and scope. The challenge has more than one thing wrong, and all of them must be corrected to make it compile and work.
Remember that you can declare and assign at the same time, with something like this:
string mytext = GetSome();
and something being assigned must be declared at the same time if it has not been before.
On the other hand, if you want something to be available outside the scope of where it is being assigned, it must be declared outside, and before it is assigned. For example, this code:
if (x == 1)
{
string mytext = "yes";
}
DoSomethingWith(mytext);
will not work, since mytext is being used outside the scope of where it is declared, but this:
string mytext = "";
if (x == 1)
{
mytext = "yes";
}
DoSomethingWith(mytext);
should be fine, since mytext is declared outside the conditional block and not inside. It is also pre-assigned so it will contain valid (if empty) contents in case the conditional test is false.
If your first attempt is not successful, be sure to check the error messages carefully to see if the problem is with your change, or if your change is good and there are still other issues to be corrected.
If you still need additional assistance, you might try posting your unsuccessful solution attempt here.
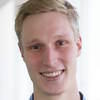
Stefan Cimander
37,115 PointsThe problem you are currently facing in your code is that the last statement tries to access the output variable, which is not in the scope of the Main() method but only visible inside the if-else-statement.
Console.Write(output) // output is not visible
Instead, try to declare the output variable outside of the if-else-statement. Within, the variable is then visible for manipulation, but pay attention for not redeclaring it but only assigning the according values.
So instead of this...
if (input == "quit")
{
string output = "Goodbye.";
}
Console.Write(output);
...you should probably try that...
string output = "";
if (input == "quit") {
// Manipulation of the output variable without redeclaring it
}
Console.Write(output);
Hope this helps.
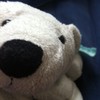
bothxp
16,510 PointsHi,
The challenge is to make the code compile, as at the moment it won't. You need to take a close look at the input & output variables. How and where they are being declared and also their 'scope'.
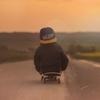
Nipi Tiri
2,988 PointsIf I put the output variable outside if else statement then i get 2 another syntax error, but if i dont then i get one, which says that i have to declare this variable outside the scope :/
using System;
namespace Treehouse.CodeChallenges
{
class Program
{
static void Main()
{
string input = Console.ReadLine();
if (input == "quit")
{
string output = "Goodbye.";
}
else
{
string output = "You entered " + input + ".";
}
Console.WriteLine(output);
}
}
}

Javier Busto
12,917 PointsHere's the solution. First you need to declare the variable type (string) for the "input" variable inside the Main () method. Then you need to include 'Console.WriteLine(output)' into the scope of the 'if/ else' statement. Like this:
using System;
namespace Treehouse.CodeChallenges
{
class Program
{
static void Main()
{
//Declare the "input" variable type here as 'string'
string input = Console.ReadLine();
if (input == "quit")
{
string output = "Goodbye.";
}
else
{
string output = "You entered " + input + ".";
// Move this into the if/ else scope.
Console.WriteLine(output);
}
}
}
}
Nipi Tiri
2,988 PointsNipi Tiri
2,988 PointsLOL, thanks, i figured out now, i was so dumb here :D