Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial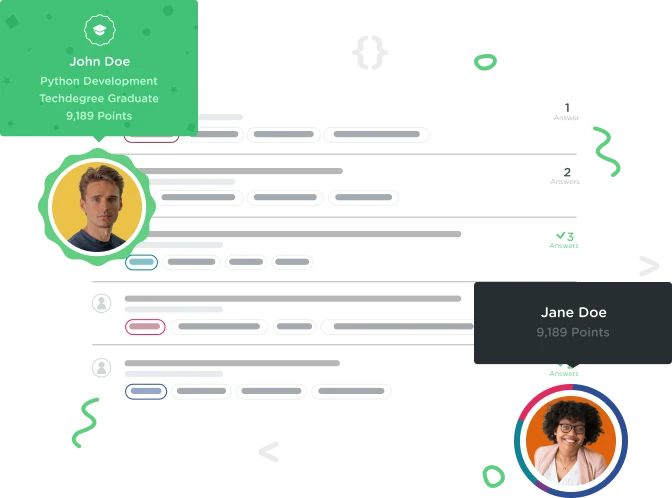

Anthony Sabberton
4,864 Pointsstruggling to add object to an array
Hi everyone. I have been experimenting with functions, loops, arrays and objects but Inevitably I have gotten hung up on an issue I'm hoping someone can help me with.
Below is the code.
const workExperience = [];
const fieldTest = test => {
const isFieldEmpty = test;
if (!isFieldEmpty) {
alert( 'You must type something!');
return true;
} else {
return false;
}
};
const YesNoTest = question => {
if ( question.toUpperCase() !== yes && question.toUpperCase() !== no ) {
alert( 'You must type Y (Yes) or N (No) to proceed' );
return true;
};
};
const buildWorkExpArray = () => {
const jobObjects = {};
let question = '';
do {
question = prompt('What is your most recent job title?');
} while ( fieldTest(question) );
jobObjects.jobTitle = question;
do {
question = prompt('What are the start and end dates? format: Year/Month/day - Current');
} while ( fieldTest(question) );
jobObjects.year = question;
do {
question = prompt('please write a description of your job role');
} while ( fieldTest(question) );
jobObjects.description = question;
workExperience.push(jobObjects);
do {
question = prompt('Do you have any other jobs you would like to add? Y or No');
} while ( YesNoTest(question) );
while ( question.toUpperCase() !== no ) {
alert('The next job you enter should be a job that was immediately preceeding the job you just entered');
do {
question = prompt('what was the job title? ');
} while ( fieldTest(question) );
jobObjects.jobTitle = question;
do {
question = prompt('What were the start and end dates? format: Year/Month/day - Year/Month/day');
} while ( fieldTest(question) );
jobObjects.year = question;
do {
question = prompt('please write a description of the job role');
} while ( fieldTest(question) );
jobObjects.description = question;
workExperience.push(jobObjects)
do {
question = prompt('Do you have any other jobs you would like to add? Y or N');
} while ( YesNoTest(question) );
};
};
In the code above I'm using question = prompt to update the jobObject object key values with the keys: .jobTitle .year .description
When I try and push the updated object (jobObject) to the workExperience array, it will update the array just fine BUT when It loops again, the newly updated jobObject will overwrite what was already pushed to the workExperience array.
if I write workExperience; in the console it will return for example:
0: { jobTitle: 'job 2', date: 'date 2', description: 'description 2' }
1: { jobTitle: 'job 2', date: 'date 2', description: 'description 2' }
where as I was expecting it to be this:
0: { jobTitle: 'job 1', date: 'date 1', description: 'description 1' }
1: { jobTitle: 'job 2', date: 'date 2', description: 'description 2' }
Any help would be much appreciated.
Cheers!
2 Answers

Brad Chandler
15,301 PointsI believe what is happening is that you are updating the first jobObjects that was pushed into the array.
When you push it into the array, this doesn't create a copy of the object in the array, it stores a reference to the object in the array.
Therefore, the proceeding updates to jobObjects are updating the first item in the array. Then when you push the jobObjects into the array the second time you end up storing 2 copies of the same object.
One quick solution is to create a new object for the second set of questions.
Another solution is to create a shallow copy of your object and push that into the array.
workExperience.push({...jobObjects});
This uses a spread operator
...
to "spread" the properties of jobObjects into a new object and push that into the array.
A third solution is to create a true copy of the object and push that into the array. This can be useful in other situations where you want to be certain you aren't altering the original object in any way.
workExperience.push(JSON.parse(JSON.stringify(jobObjects)));
This converts the object to string then parses it back to JSON which will push a completely separate version of the jobObjects object.

Anthony Sabberton
4,864 PointsCheers Brad!
The last concept I hadn't learnt about yet but good to know! I ended up using the magical ... to make a soft copy and push to the array.
Thanks again!