Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial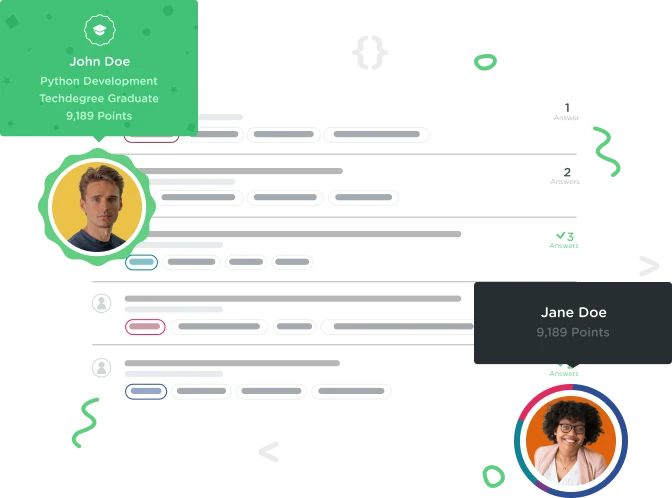
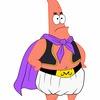
<noob />
17,063 Pointsstruggling with this challange..
Hi,
I dont understand why we need to use getters and setters, Im quite new to OOP, i had expirience with javascript and python so far and im focus my attention new on c# and i find this topic very diffecult...
amespace Treehouse.CodeChallenges
{
class Frog
{
private GetNumFliesEaten _numFliesEaten;
public int GetNumFliesEaten()
{
return _numFliesEaten;
}
public void SetNumFliesEaten(int val)
{
_numFliesEaten = val;
}
}
}
i find this solution in the forum, someone can explain to me what is the purpose of adding the "_" to numFliesEaten, Why we need to return _numFliesEaten and then assign it to a value?
why we use getters and setters?
namespace Treehouse.CodeChallenges
{
class Frog
{
private GetNumFliesEaten _numFliesEaten;
public int GetNumFliesEaten()
{
return _numFliesEaten;
}
public void SetNumFliesEaten(int value)
{
_numFliesEaten = value;
}
}
}
1 Answer

Tyler B
5,787 PointsHey Noob,
To answer your first and most straight forward question the "_" is a naming convention to identify that the variable in question has an access of private
. This is not required by the compiler but is HIGHLY recommended for readability.
Now to your other question we use getters and setters to conform to the OOP core concept of Encapsulation. Encapsulation gives us the notion that only an object itself should be able to directly access and/or modify data it "owns" and that the object has full authority to decide how data is or is not bundled for access or modification. The reasons for this on there on can seem confusing especially when all your doing is accessing and modifying a single value in a single field but, as complexity builds these rules will be life savers. Lets take the example above and try to give a good example. Lets say the Frog IRS (LOL) descends upon our frogs that we build with Frog.cs, they tell us that every time we collect any number over 3 flies we have to give 1 fly to them. Now we could try and remember every time we collect flies to do this math but if we forget 1 time or if someone else extends our code and doesn't notice it's required we'd be in for a froggy audit! So instead we could just update our setter
public void SetNumFliesEaten(int value)
{
if(value > 3)
{
_numFliesEaten = value - 1;
}
_numFliesEaten = value;
}
Now every time we set our flies eaten the math is done for us. No froggy audit for these frogs! I hope that helps! Let me know if I can provide more context/answers.
Steven Parker
241,970 PointsSteven Parker
241,970 Points