Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial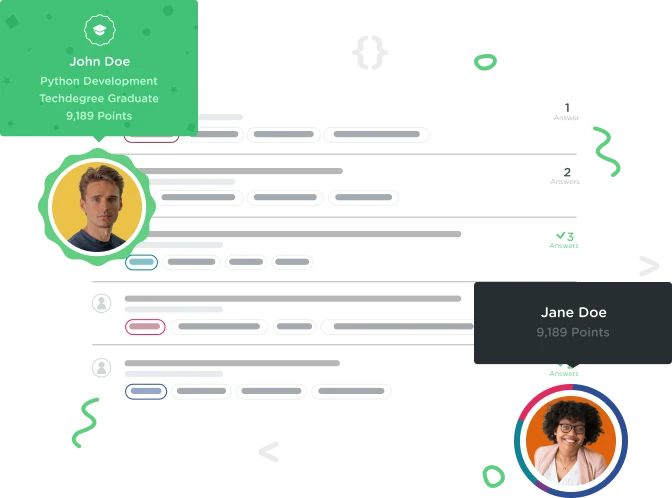

Zoltan Szalas
1,580 PointsStuck on last forum exercise- please help! I've been trying to figure out the 'I expected to see the names passed in...'
I was able to get past all the Forum exercises relatively easy, but this last one has me stuck and I've spent 2 hours trying to figure it out. Any and all help is much appreciated
public class ForumPost {
private User mAuthor;
private String mTitle;
private String mDescription;
public String getDescription(){
return mDescription;
}
public User getAuthor() {
return mAuthor;
}
public String getTitle() {
return mTitle;
}
public ForumPost(User author, String title, String description){
mAuthor = author;
mTitle = title;
mDescription = description;
}
// TODO: We need to expose the description
}
public class User {
private String mFirstName;
private String mLastName;
public String getFirstName(){
return mFirstName;
}
public String getLastName(){
return mLastName;
}
public User(String firstName, String lastName) {
// TODO: Set the private fields here
mFirstName = firstName;
mLastName = lastName;
}
}
public class Forum {
private String mTopic;
public String getTopic() {
return mTopic;
}
public Forum(String topic){
mTopic = topic;
}
public void addPost(ForumPost post) {
System.out.printf("New post from %s %s about %s.\n",
post.getAuthor().getFirstName(),
post.getAuthor().getLastName(),
post.getTitle());
}
}
public class Example {
public static void main(String[] args) {
System.out.println("Starting forum example...");
if (args.length < 2) {
System.out.println("first and last name are required. eg: java Example Craig Dennis");
}
Forum forum = new Forum("Java");
// Take the first two elements passed args
User author = new User("Zoltan", "Szalas");
// Add the author, title and description
ForumPost post = new ForumPost(author, "Fuckin Eh! I did it", "Can't stop me");
forum.addPost(post);
}
}
3 Answers
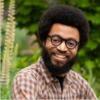
Nicolas Hampton
44,647 PointsZoltan,
so many people have spent 2 hours on this question that there's tons of answers on the forum, and I'm pretty sure one of them has your answer. Click on the wrapping up button on this question and take a look, see if you can find the answer. If it's not there, or you have a specific question, shout again. I personally put a couple hours into this one, so don't worry about it. That's programming, and a lot of it is looking up the answer. Good luck!
Nicolas
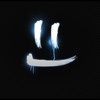
Grigorij Schleifer
10,365 PointsO man, i have the feeling, that i spent my whole life on this challenge and i need help anyway here ...
We need something like this, but i dont understand where in the User class is the array?
User author = new User(args[0], args[1]);
Shout out, if you could figure it out
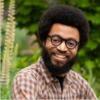
Nicolas Hampton
44,647 PointsAlright, from what I can see of the challenge in the comment code, it wants you to take the arguments passed in from the String[] args in the main class declaration of Example.java and use them to fill the arguments for the declaration of your new user. So the line, so far as I can see, that needs to be changed, is:
User author = new User(arg[0], arg[1]);
at a long glance, that's all I can see wrong, but be careful. Your code looks good (I say preemptively, I haven't fine combed it yet), I think it's just an issue of being able to read and follow the directions, which I recall on theis challenge is no walk in the park. It's not your code skill that's the problem I believe, it's being able to correctly identify the problem for what it is.
Happy coding! Nicolas
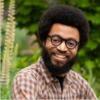
Nicolas Hampton
44,647 PointsWhat does the question say, and what error are you getting?
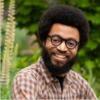
Nicolas Hampton
44,647 PointsYeah, I looked it over, everything is right. It just wants you to make the program so that the arguments you pass into the program are your first and last name, and then it makes this post from that. That's why it's telling you 'I expected to see the names passed in...'.