Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial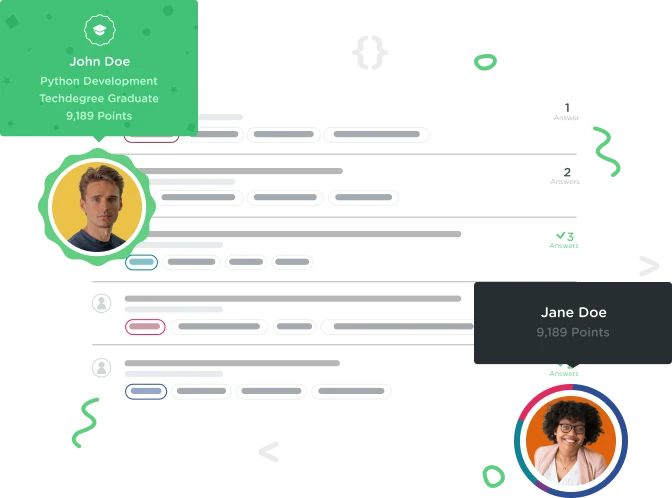

Frank Peer
4,780 PointsStumped on the challenge
I get this error message with my current code: "I entered Anderson and expected to get back line 1 as A comes before M. "
I don't think that's the problem because my parameters are set to be greater than or equal to A and less than or equal to M. I think I'm missing something else, but I can't put my finger on it.
public class ConferenceRegistrationAssistant {
public int getLineFor(String lastName) {
/* If the last name is between A thru M send them to line 1
Otherwise send them to line 2 */
if (lastName.charAt(0) >= 'A' && lastName.charAt(0) <= 'M') {
int line = 1;
} else {
int line = 2;
}
int line = 0;
return line;
}
}
3 Answers

Axel McCode
13,869 PointsHi Frank!
Here is the code I used for this code challenge:
First, I declared a char ch, and set its value to the character at index 0 of string lastName (1)
In the if-else block I test whether ch is between 'A' and 'M'. (2)
Remember that in Java, A < M and Z > M
So if this test evaluates to true, int line would be set to 1. If the test evaluates to false then int line would be set to 2.
Hope this helps!
-Axel
public class ConferenceRegistrationAssistant {
public int getLineFor(String lastName) {
/* If the last name is between A thru M send them to line 1
Otherwise send them to line 2 */
int line = 0;
char ch = lastName.charAt(0); // 1
if(ch <= 'M') { // 2
line = 1;
} else {
line = 2;
}
return line;
}
}

Nikolay Mihaylov
2,810 PointsYou have declared int line 3 times. The last one overrides the first two.
public int getLineFor(String lastName) {
/* If the last name is between A thru M send them to line 1
Otherwise send them to line 2 */
if (lastName.charAt(0) >= 'A' && lastName.charAt(0) <= 'M') {
int line = 1;
} else {
int line = 2;
}
int line = 0; // int line is always 0.
return line;
}
}
Jesse James
3,020 PointsAnother way to look at this and shorten the total amount of code is that since this is more or less a "Is it the same or bigger than X" type question, you only have to account for that portion of it when you test.
if (lastName.chartAt(0) >= 'M') { line = 2 ; } else { line = 1; }
By design, if you're not M or greater than M, you'd have to be in the other group.
Just sharing a thought :)

Frank Peer
4,780 PointsA good thought. Thanks.
Frank Peer
4,780 PointsFrank Peer
4,780 PointsThanks, Axel! That's a helpful way of utilizing Characters.