Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial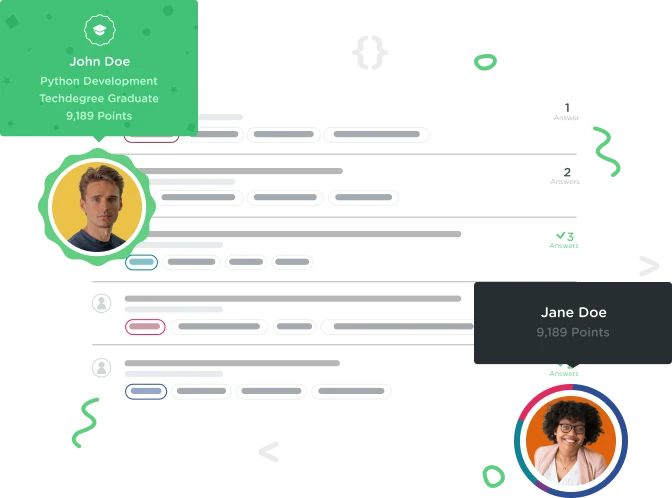

Jessica Mylius
Courses Plus Student 1,269 PointsSubclass Challenge Question I cannot get past and feel I'm doing what's asked!
Challenge: In the editor you've been provided with two classes - Point to represent a coordinate point and Machine. The machine has a move method that doesn't do anything.
Your task is to subclass Machine and create a new class named Robot. In the Robot class, override the move method and provide the following implementation. If you enter the string "Up" the y coordinate of the Robot's location increases by 1. "Down" decreases it by 1. If you enter "Left", the x coordinate of the location property decreases by 1 while "Right" increases it by 1.
Note: If you use a switch statement you can use the break statement in the default clause to exit the current iteration.
class Point { var x: Int var y: Int
init(x: Int, y: Int) { self.x = x self.y = y } }
class Machine { var location: Point
init() { self.location = Point(x: 0, y: 0) }
func move(_ direction: String) { print("Do nothing! I am a machine!") } }
Here is my answer:
class Robot: Machine { func move(direction: String) { switch direction{ case "Up": location.y += 1 case "Down": location.y -= 1 case "Left": location.x -= 1 case "Right": location.x += 1 default: break } } }
And the error message I keep receiving says "Bummer! For the Up direction make sure the location's y property increases by 1"
I feel like I have retried writing every which way. Please help me move on through this challenge!
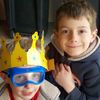
Chris Stromberg
Courses Plus Student 13,389 PointsTo display your code type the following
```swift
Your code would go here.
```
Also what video/course is this challenge from?
2 Answers
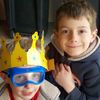
Chris Stromberg
Courses Plus Student 13,389 PointsYou need to add "override" to your function per the challenge. I have attached an explanation for why you would use override.
override func move(_ direction: String) {
switch direction{
case "Up": location.y += 1
case "Down": location.y -= 1
case "Left": location.x -= 1
case "Right": location.x += 1
default: break }
}
From the Swift Programming Guide
Overriding
A subclass can provide its own custom implementation of an instance method, type method, instance property, type >property, or subscript that it would otherwise inherit from a superclass. This is known as overriding.
To override a characteristic that would otherwise be inherited, you prefix your overriding definition with the override >keyword. Doing so clarifies that you intend to provide an override and have not provided a matching definition by >mistake. Overriding by accident can cause unexpected behavior, and any overrides without the override keyword are >diagnosed as an error when your code is compiled.
The override keyword also prompts the Swift compiler to check that your overriding classβs superclass (or one of its >parents) has a declaration that matches the one you provided for the override. This check ensures that your overriding >definition is correct.

Jessica Mylius
Courses Plus Student 1,269 PointsThank you! I entered "override" before and it was giving me an error, but for some reason it worked today.
Jessica Mylius
Courses Plus Student 1,269 PointsJessica Mylius
Courses Plus Student 1,269 PointsAlso sorry if the way I pasted the code is confusing. I don't know how to post the code as it looks in the challenges.