Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial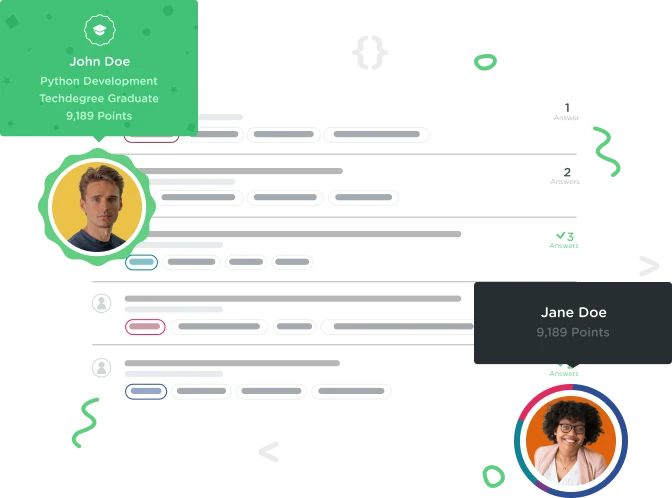

Nick Davies
7,972 PointsSubmitting a form with PHP
Hello I have been trying to roll out the PHP code to submit a form on my site although when pressing send message it just goes to sendformemail.php with nothing on it where as it should be redirecting to contact-thanks.php or throwing up an error message? This is for a project I am working on.
Here is my code so far: HTML
<form id="contact-form" method="post" action="sendformemail.php">
<fieldset>
<legend>Contact Me</legend>
<label for="name">Name:</label>
<input type="text" id="name" name="user_name" placeholder="Enter your name here" class="clear-right">
<label for="email">Email:</label>
<input type="email" id="email" name="user_email" placeholder="Enter your email address here!" class="clear-right">
<label for="job">Reason for contact:</label>
<select id="job" name="user_job" class="clear-right">
<optgroup label="Work">
<option value="Application Development">Application Development/Design</option>
<option value="Website Design">Website Development/Design</option>
<option value="Computer Diagnostics">Computer Diagnostics</option>
</optgroup>
<optgroup label="Business">
<option value="looking for a job">Looking for a Job</option>
</optgroup>
<optgroup label="Alternative">
<option value="Other Reason">Other Reason</option>
<option value="Ask a question" selected>Ask a Question</option>
</optgroup>
</select>
<label for="textarea">Message:</label>
<textarea id="textarea" name="user_text" rows="10" cols= "50" placeholder="Write your message here..."></textarea>
<label style="display:none;" for="address">address</label>
<input style="display:none;" type="text" id="address" name="address" placeholder="Humans do not enter anything in here." class="clear-right">
</fieldset>
<button type="submit" class="secondary">Send Message</button>
</form>
PHP
<?php
if ($_SERVER["REQUEST_METHOD"] == "POST") {
$name = trim($_POST["user_name"]);
$email = trim($_POST["user_email"]);
$jobtype = trim($_POST["user_job"]);
$message = trim($_POST["user_text"]);
if ($name =="" OR $email == "" OR $job == "" OR $message == "") {
echo "you must specify a value for name, email address and message";
exit;
}
foreach ($_POST as $value) {
if (stripos($value, 'Content-Type:')!== FALSE) {
echo "There was a problem submitting your form";
exit;
}
}
if ($_POST["address"] != "") {
echo "Your form submission has an error.";
exit;
}
require_once('inc/class.phpmailer.php');
$mail = new PHPMailer();
if (!$mail->ValidateAddress($email)) {
echo "You must specify a valid email address";
exit;
}
$email_body = "";
$email_body = $email_body . "Name: " . $name . "<br>";
$email_body = $email_body . "Email Address: " . $email . "<br>";
$email_body = $email_body . "Reason for comntact: " . $jobtype . "<br>";
$email_body = $email_body . "Message: " . $message;
Server: smtp.postmarkapp.com
Ports: 25, 2525, or 587
Username/Password: **************************
Authentication: Plain text, CRAM-MD5, or TLS
$mail->SetFrom($email,$name);
$address = "me@Domain.info";
$mail->AddAddress($address, "Name");
$mail->Subject = $job;
$mail->MsgHTML($email_body);
if(!$mail->Send()) {
echo "The was a problem sending your message: " . $mail->ErrorInfo;
exit;
}
header("location: contact-thanks.php");
exit;
}
?>
Can anyone figure out and let me know why it is doing this?
Thanks in advance
4 Answers
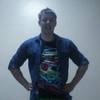
Ernestas Sipavicius
6,327 Pointstry this:
<?php
if ($_SERVER["REQUEST_METHOD"] == "POST") {
$name = trim($_POST["user_name"]);
$email = trim($_POST["user_email"]);
$job = trim($_POST["user_job"]);
$message = trim($_POST["user_text"]);
if ($name == "" OR $email == "" OR $job == "" OR $message == "") {
echo "you must specify a value for name, email address and message";
exit;
}
foreach ($_POST as $value) {
if (stripos($value, 'Content-Type:')!== FALSE) {
echo "There was a problem submitting your form";
exit;
}
}
if ($_POST["address"] != "") {
echo "Your form submission has an error.";
exit;
}
require_once('inc/class.phpmailer.php');
$mail = new PHPMailer();
if (!$mail->ValidateAddress($email)) {
echo "You must specify a valid email address";
exit;
}
$email_body = "";
$email_body = $email_body . "Name: " . $name . "<br>";
$email_body = $email_body . "Email Address: " . $email . "<br>";
$email_body = $email_body . "Reason for comntact: " . $job . "<br>";
$email_body = $email_body . "Message: " . $message;
//Server: smtp.postmarkapp.com
//Ports: 25, 2525, or 587
//Username/Password: **************************
//Authentication: Plain text, CRAM-MD5, or TLS
$mail->SetFrom($email,$name);
$address = "name@domain.com";
$mail->AddAddress($address, "Name");
$mail->Subject = $job;
$mail->MsgHTML($email_body);
if(!$mail->Send()) {
echo "The was a problem sending your message: " . $mail->ErrorInfo;
exit;
}
header("location: contact-thanks.php");
exit;
}
?>

Christian Andersson
8,712 PointsGood point. There is some text there that isn't code. Maybe it's what's causing the problem.

Nick Davies
7,972 PointsBingo, that was the issue. Thanks :)
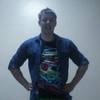
Ernestas Sipavicius
6,327 PointsAt the beginning you have starting tag
<form action='my-file.php' method='post'> ?

Nick Davies
7,972 PointsYes that not all the HTML for the page, I figured the form HTML was all that was needed, along with the PHP

Nick Davies
7,972 PointsMy apologise I have just added it now

Christian Andersson
8,712 PointsWhat you should do is start writing some else
's in your conditionals and have some "test" echoes in there to see where it doesn't enter where it should. Every echo you have is within an if-clause so perhaps they don't enter for some reason.
For example, your entire code will only run provided the ($_SERVER["REQUEST_METHOD"] == "POST")
returns true. Perhaps this is false (although your code seems correct)? You should put this to the test with an else
. It's a great way to troubleshoot.

Nick Davies
7,972 PointsI added these else's in my conditionals and still nothing is happening.
<?php
if ($_SERVER["REQUEST_METHOD"] == "POST") {
$name = trim($_POST["user_name"]);
$email = trim($_POST["user_email"]);
$jobtype = trim($_POST["user_job"]);
$message = trim($_POST["user_text"]);
if ($name =="" OR $email == "" OR $jobtype == "" OR $message == "") {
echo "you must specify a value for name, email address and message";
exit;
else {
echo "this doesnt work"
}
}
foreach ($_POST as $value) {
if (stripos($value, 'Content-Type:')!== FALSE) {
echo "There was a problem submitting your form";
exit;
else {
echo "this doesnt work 2"
}
}
}
if ($_POST["address"] != "") {
echo "Your form submission has an error.";
exit;
else {
echo "this doesnt work 3"
}
}
require_once('inc/class.phpmailer.php');
$mail = new PHPMailer();
if (!$mail->ValidateAddress($email)) {
echo "You must specify a valid email address";
exit;
else {
echo "this doesnt work 4"
}
}
$email_body = "";
$email_body = $email_body . "Name: " . $name . "<br>";
$email_body = $email_body . "Email Address: " . $email . "<br>";
$email_body = $email_body . "Reason for comntact: " . $jobtype . "<br>";
$email_body = $email_body . "Message: " . $message;
Server: smtp.postmarkapp.com
Ports: 25, 2525, or 587
Username/Password: *********************
Authentication: Plain text, CRAM-MD5, or TLS
$mail->SetFrom($email,$name);
$address = "me@nickdavies.info";
$mail->AddAddress($address, "Nick Davies");
$mail->Subject = $job;
$mail->MsgHTML($email_body);
if(!$mail->Send()) {
echo "The was a problem sending your message: " . $mail->ErrorInfo;
exit;
else {
echo "this doesnt work 4"
}
}
header("location: contact-thanks.php");
exit;
}
?>```

Christian Andersson
8,712 PointsTry the very first if
.
if ($_SERVER["REQUEST_METHOD"] == "POST") {

Nick Davies
7,972 PointsThat hasnt seemed to work either, possibly it is a syntax error?
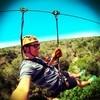
thomascawthorn
22,986 PointsPut this at the very beginning of your script - the very first thing that gets included/hit by the request.
<?php
error_reporting(-1);
ini_set('display_errors', 'On');
Are you getting any errors back?
Christian Andersson
8,712 PointsChristian Andersson
8,712 PointsEdited for markdown