Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial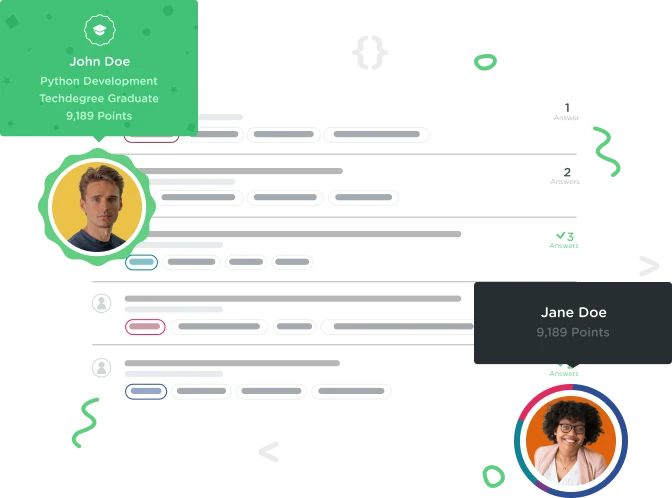

William Forbes
21,469 Pointsswift structure
I cannot figure out what I am doing wrong here? I believe I am initializing and using the right syntax but I keep getting errors
Any help would be greatly appreciated.
Thanks in advance!
struct Tag {
let name: String
init (name: String) {
self.name = name
}
}
struct Post {
let title: String
let author: String
let tag: Tag.name
init(title: String, author: String, Tag: Tag.name) {
self.title = title
self.author = author
self.tag = Tag.name
}
func description() {
return "\(title) by \(author) under \(tag)"
}
}
let swift = Tag(name: "Swift")
let firstPost = Post(title: "johN", author: "steve",tag: swift.name)
let postDescription = firstPost.description()
4 Answers
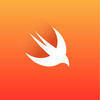
Steven Deutsch
21,046 PointsHey William,
Let's see if I can clear some things up for you:
First, you don't need an initializer when using structures. Swift automatically provides one for you - known as the memberwise initializer.
Second, Post has a constant stored property named tag of type Tag. Not Tag.name, as you have it written. You now need to create an instance of Post and assign it to the constant firstPost. You do this by passing values for each property, of their respective type. So you will need to pass in a string for title, a string for author, and an instance of Tag for tag. You can create the instance of tag inside the Post initialization, by passing it a string value for name.
// Created instance of Post with Tag instance for last parameter
let firstPost = Post(title: "iOSDevelopment", author: "Apple", tag: Tag(name: "Swift"))
Third, we have to fix your instance method. You haven't specified a return type. So your function can't return anything. It needs to return a string. Also, for the third interpolated value, it needs to be Tag.name. You are accessing the name property of the Tag instance.
// Added return type to instance method, and accessed tag.name property
func description() -> String {
return "\(title) by \(author). Filed under \(tag.name)"
}
Here is my version of the complete code:
struct Tag {
let name: String
}
struct Post {
let title: String
let author: String
let tag: Tag
func description() -> String {
return "\(title) by \(author). Filed under \(tag.name)"
}
}
let firstPost = Post(title: "iOSDevelopment", author: "Apple", tag: Tag(name: "Swift"))
let postDescription = firstPost.description()
Hope this helps. Good Luck!
PS. If anyone is looking to join a slack channel to collaborate on iOS projects and work on learning Swift in real time, reply below with an email and I will send you an invite!
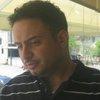
Mazen Halawi
7,806 PointsHey Steven, yeah i dont mind. how do i get around that?
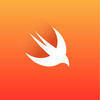
Steven Deutsch
21,046 Pointse-mail me at stevensdeutsch@gmail.com and I will send you an invite. or post an email for me to send the invite to below.

aakarshrestha
6,509 PointsGo through this code and see if it helps you:
struct Tag {
let name: String
}
struct Post {
let title : String
let author : String
let tag : Tag
init(title: String, author: String, tag: Tag) {
self.title = title
self.author = author
self.tag = tag
}
}
let myTag = Tag(name: "programming")
let post = Post(title: "Programming 101", author: "Joe The Plumber", tag: myTag)
let titleString : String = post.title
let authorString : String = post.author
let tagNameString = post.tag.name
print("\(titleString), \(authorString), \(tagNameString)")
Hope it helps!
Happy Coding!
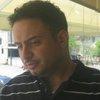
Mazen Halawi
7,806 Pointshi, how do you post your code without having it cut out like in my case?
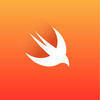
Steven Deutsch
21,046 PointsMazen Halawi, I think you just have to account for the spacing. You look pretty determined to learn iOS! Interesting in joining a Slack community dedicated to learning Swift?

William Forbes
21,469 Pointsthank you everyone!

aakarshrestha
6,509 PointsI am interested... amshrestha12@gmail.com
Happy coding!
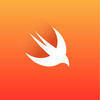
Steven Deutsch
21,046 PointsInvite sent :) In case you want to remove your email by deleting this comment/answer
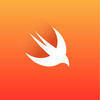
Steven Deutsch
21,046 PointsInvite sent :) In case you want to remove your email by deleting this comment/answer
Mazen Halawi
7,806 PointsMazen Halawi
7,806 Points