Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial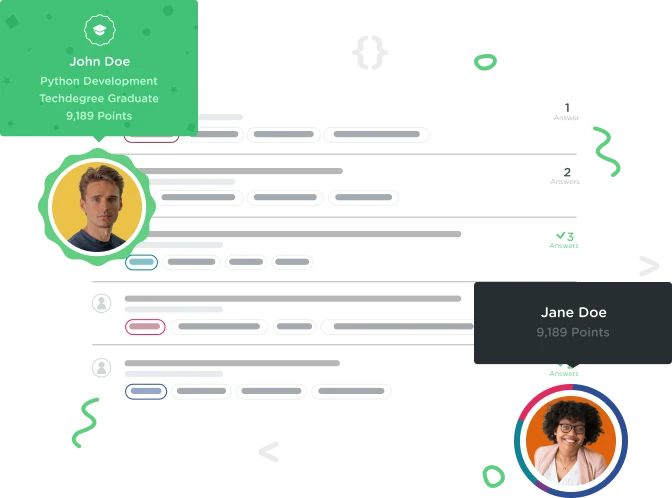
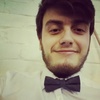
Benjamin Ing
910 Pointssyntax error
I have a syntax error and can't find where I went wrong
public class GoKart {
public static final int MAX_BARS = 8;
private String mColor;
private int mBarsCount;
public GoKart(String color) {
mColor = color;
mBarsCount = 0;
}
public String getColor() {
return mColor;
}
public void drive() {
drive(1);
}
public void drive(int laps) {
// Other driving code omitted for clarity purposes
newAmount = mBarsCount -= laps;
if(newAmount > mBarsCount)
{
throw new IllegalArgumentException("Not enough battery remains");
}
mBarsCount -= laps;
}
public void charge() {
while (!isFullyCharged()) {
mBarsCount++;
}
}
public boolean isBatteryEmpty() {
return mBarsCount == 0;
}
public boolean isFullyCharged() {
return mBarsCount == MAX_BARS;
}
}
1 Answer
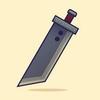
Allan Clark
10,810 Points public void drive(int laps) {
// Other driving code omitted for clarity purposes
newAmount = mBarsCount -= laps;
if(newAmount > mBarsCount)
{
throw new IllegalArgumentException("Not enough battery remains");
}
mBarsCount -= laps;
}
The newAmount variable does not have a type. Also actually the newAmount variable is not needed, you set it to be the number of bars minus the laps then use it in the conditional. What this does is finds the amount of bars that will be left after the laps have been driven and then checks if that amount is less than what you started with (i.e. mBarsCount). This will always be true so it will always throw the exception. Instead we just want to check if the laps variable is more than the number of bars we have. It could look something like this:
public void drive(int laps) {
// Other driving code omitted for clarity purposes
if(laps > mBarsCount)
{
throw new IllegalArgumentException("Not enough battery remains");
}
mBarsCount -= laps;
}