Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial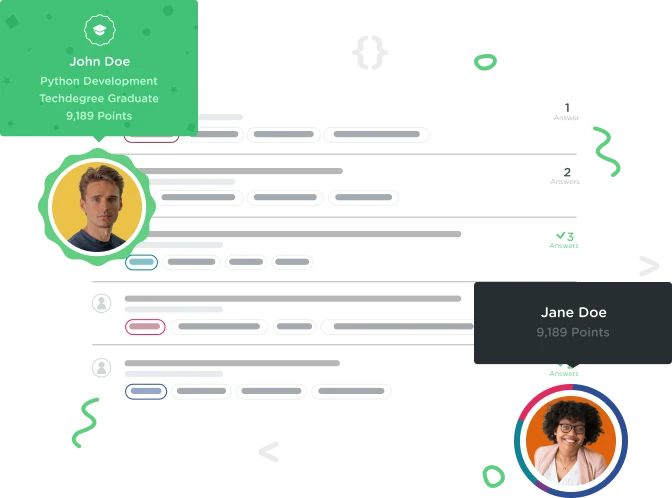
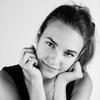
Dorota Parzych
5,706 Pointstask with initials
Hi! I'v wanted to write a function that converts a name into initials.
The output should be capital letters with a dot separating them.
It should look like this:
Sam Harris => S.H
Patrick Feeney => P.F
My code looks like this and it doesn't work (There is a space in between )
function abbrevName(name){
var initials =[];
for(var i=0; i<name.length; i++){
if( (name[i] != ' ') && (i!=0) ){
if(name[i] === name[i].toUpperCase() ) {
initials += '.'+name[i];
}
}else{
initials += name[i];
}
}
return initials;
}
console.log(abbrevName('Harrison Ford'));
1 Answer

Zimri Leijen
11,835 PointsYou're making it pretty hard for yourself.
Why not just seperate the name into words by using split?
You can split at the space, to get an array of words (in this case, an array of names). Then you loop over the array and take just the first letter of each (the initial) and add a period.
Then return the result.
Also, if you want a placeholder that is meant to hold strings, use an empty string, not an empty array. An array will work, but it just makes it look confusing.
Dorota Parzych
5,706 PointsDorota Parzych
5,706 PointsSo I have changed the code... the part with adding the period still confuses me though... I think that it could be done differently... Anyway...everything would work fine but the output is: "undefinedH.B.F"
Zimri Leijen
11,835 PointsZimri Leijen
11,835 PointsGood progress,
one way of fixing the 'undefined' and the . would be to do it like this:
There's also another thing you can do, which is basically the opposite of split.
That way, you'd store the initials in an array, and in the end you join the array with
initials.join('.')
.Like so:
note that in this way, if you want the dot at the end, you'd have to add it.
Dorota Parzych
5,706 PointsDorota Parzych
5,706 PointsNow it works! Thanks! :D