Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial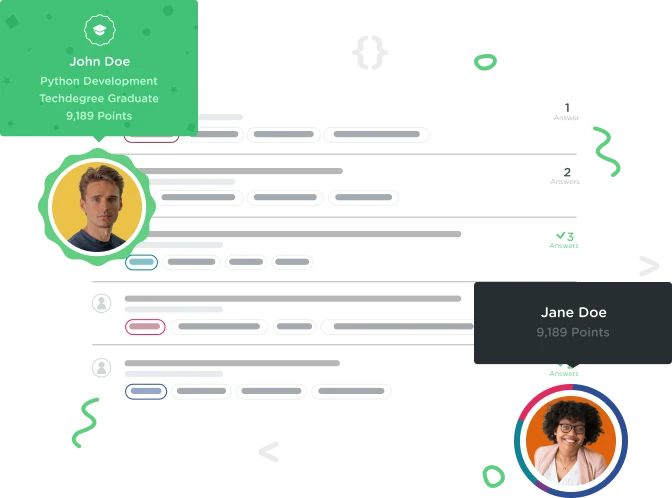

Ana Adrian
9,065 PointsThe code writes the output only after exiting the while loop.
class Student {
constructor(name, track, achievements, points) {
this.Name = name;
this.Track = track;
this.Achievements = achievements;
this.Points = points;
}
}
let students = [
new Student('Adi', 'Python', "10", 9000),
new Student('Clau', 'JS', "2", 1000),
new Student('Ade', 'C++', "4", 2000),
new Student('Hera', 'Go', "5", 3000),
new Student('Tyson', 'Ruby', "6", 4000)
]
let all_students = new Map();
for (student of students) {
let key = student.Name.toLowerCase();
all_students[key] = student;
}
const print = (msg) => {
let elOutput = document.getElementById('output');
elOutput.innerHTML = msg;
};
const getStudentRecord = (student) => {
let report = `<h2>Student: ${student.Name}</h2>`;
for (let key in student) {
if (key != 'Name') {
report += `<p>${key}: ${student[key]}</p>`
}
}
return report;
};
while (true) {
let query = prompt('Find a student record: ');
if ( query === null || query === 'quit' || query === '') {
break;
}
query = query.toLowerCase();
record = all_students[query]
if (record) {
msg = getStudentRecord(record);
print(msg);
}
}
I am trying to print out the message after each loop but it's not working only after exiting the loop. Could please someone help me better understand why is this happening? Thank you!
1 Answer

Victor Mercier
14,667 PointsBased on my knowledge, it is because of an asynchronous issue. In fact, when a function is called, it get push to the call stack and can only get popped off of the stack when the function is complete. So any other action that are not asynchronous are waiting until the loop completion is done before calling any other function. This is what block your print function of running.
Ana Adrian
9,065 PointsAna Adrian
9,065 PointsThe instructor is using the same pattern but his output gets printed after each loop. I tried using setTimeout but I am sure I am doing something wrong.