Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial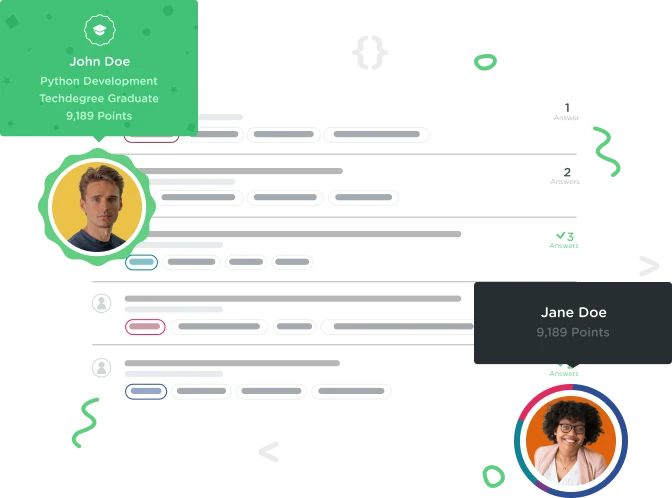
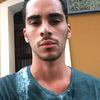
Gregory Millan
3,719 PointsThe following question. Return vs...
In the following question I believe it can be completed the either of 2 ways.
class Button {
var width: Double
var height: Double
init(width:Double, height:Double){
self.width = width
self.height = height
}
func incrementBy (points:Double) {
width = width + points
height = height + points
}
}
OR
class Button {
var width: Double
var height: Double
init(width:Double, height:Double){
self.width = width
self.height = height
}
func incrementBy(points: Double) -> Double {
return (width + points + height + points)
}
}
The difference between the two is the method. The first modifies the stored values and the second returns them
However when I enter the second code for the objective it marks it wrong, why is this if they both get the same value?
3 Answers
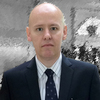
Nathan Tallack
22,164 PointsYour code block in your method is executed sequentually. That means because the first return will always be executed (which exits the scope of that code block) the second return will never be executed.
Consider the changes I have made to your code below.
class Button {
var width: Double
var height: Double
init(width:Double, height:Double){
self.width = width
self.height = height
}
func incrementBy(points: Double) -> (Double, Double) {
return (self.width + points, self.height + points)
}
}
So in the one return statement we are returning tuple containing the two doubles, width and height.
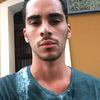
Gregory Millan
3,719 PointsHi,
Thanks, I literally just copied and pasted that. It works in Xcode but not for the objective. I guess they want us to modify the the stored properties than use a return.
Thanks though!
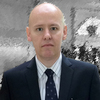
Nathan Tallack
22,164 PointsIf you link the challenge I can help work out what the problem is. Sometimes it is all up to how you read the challenge task questions. :)
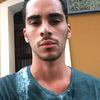
Gregory Millan
3,719 PointsHey Nathan,
Here is the link https://teamtreehouse.com/library/objectoriented-swift/classes-and-objects/classes-and-their-methods-2
Let me know if it works for you.
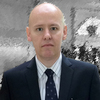
Nathan Tallack
22,164 PointsOh right. They are not wanting you to return anything. They just want you to be able to use the object's method to modify it's property. This is common in object oriented programming.
Consider the new code below.
class Button {
var width: Double
var height: Double
init(width:Double, height:Double){
self.width = width
self.height = height
}
func incrementBy(points: Double) {
self.width += points
self.height += points
}
}